parseInt() in Java
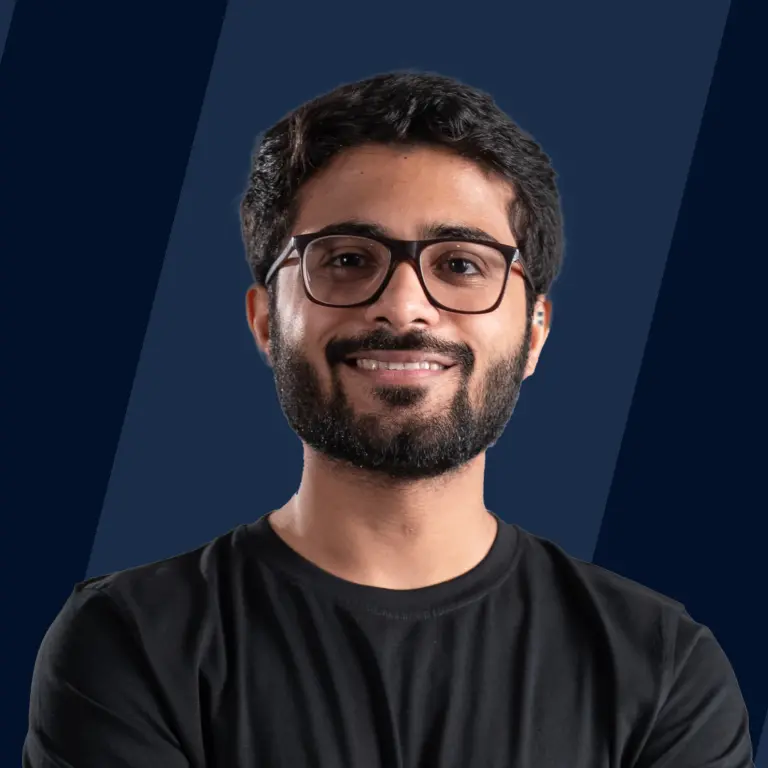
The method parseInt() belongs to the Integer class which is under java.lang package. It is used to parse the string value as a signed decimal value. It is used in Java for converting a string value to an integer by using the method parseInt().
Syntax of parseInt() in Java
Following is how the parseInt() method can be declared in java:
Parameters of parseInt() in Java
Data type | Parameter | Description |
---|---|---|
int | startIndex | It is the starting index of the string and is inclusive. |
int | endIndex | It is the ending index of the string and is exclusive. |
int | radix | It represents the base of the number in the string. |
String | s | The string that is to be converted into an integer. |
CharSequence | s | It is the sequence of characters that is to be converted to an integer. |
Return Values of parseInt() in Java
Method name | Return |
---|---|
parseInt(String s) | Returns an integer value in its decimal equivalent |
parseInt(String s, int radix) | Returns an integer value with base specified as radix. |
Note :
Radix is the parameter that specifies the number system to be used.
For example, binary = 2, octal = 8, hexadecimal = 16, etc.
Exceptions of parseInt() in Java
There are three types of exceptions possible by using the method parseInt() in java.
NullPointerException: It arises when the string s given as argument is null.
IndexOutOfBoundsException: It arises when:
- startIndex is negative
- startIndex > endIndex
- endIndex > s.length (length of given string)
NumberFormatException: It occurs when:
- CharSequence does not contain parsable int at a specified radix
- radix Character.MIN_RADIX
- radix Character.MAX_RADIX
Note:
Example of parseInt() in Java
Output:
More Examples
Example 1
Two strings are passed and are returned as their respective signed decimal Integer objects
Output:
Example 2
A user-defined example where anyone using this code can put a value of their choice and get the equivalent integer as output.
Output:
Explanation:
- Here, trim() is used to removed ending spaces from the left and right sides.
- These spaces otherwise, can cause NumberFormatException in Java.
Conclusion
- The method parseInt() belongs to the Integer class which is under java.lang package. It is used to parse the string value as a signed decimal value.
- Following are how the parseInt() method can be declared in java :
- public static int parseInt (String s).
- public static int parseInt (String s, int radix).
- public static int parseInt (CharSequence s, int startIndex, int endIndex, int radix).
- It is used in java for converting a string value to an integer by using the method parseInt().
- The parseInt() method throws three exceptions: NullPointerException, IndexOutOfBoundsException, NumberFormatException when the input arguments are not according to the conventions.