Building a Password Generator in Java
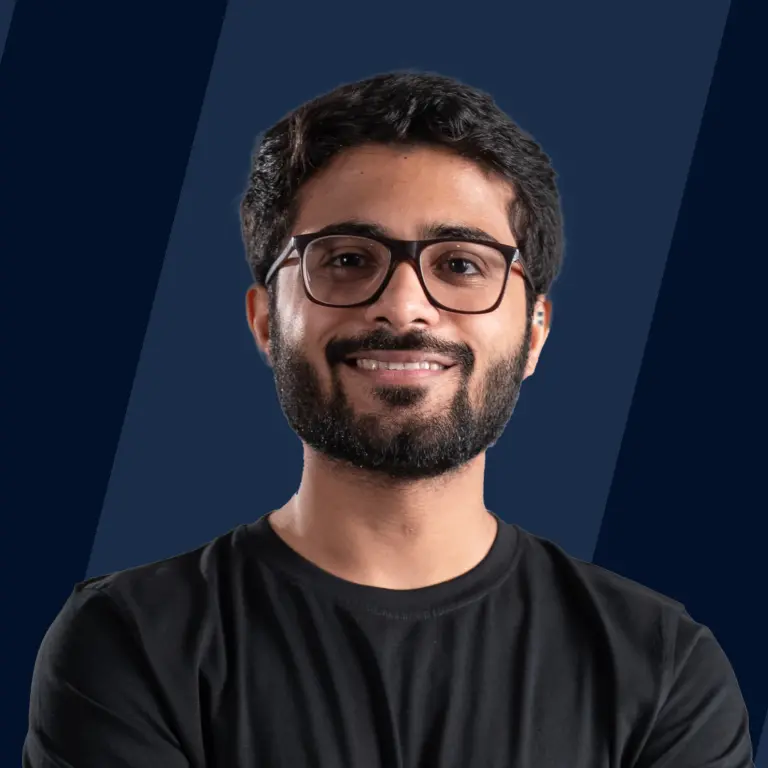
Overview
We frequently forget our passwords, so we choose the Forget password option. We quickly receive a new password to our registered email address or phone number, allowing us to log into our accounts. Additionally, we receive a new password each time. Sometimes we access our bank accounts while making purchases from an online store or in a variety of other ways. To confirm our bank account activities, they quickly send us an OTP (One Time Password) to our registered phone number or email address. This post describes how to quickly construct such passwords and OTPs as well as the codes we can use if necessary.
What are We Building?
Nearly all websites these days now ask users to create temporary passwords. If a user forgets their password, the system creates a new password at random while still complying to the company's password policy
These are the specifications for a string password.
- It should contain at least one capital case letter.
- It should contain at least one lower-case letter.
- It should contain at least one number.
- Length should be 8 characters.
- It should contain one of the following special characters:@, $, #, !
We'll use a variety of techniques, including passy, Custom Utility Method, RandomStringGenerator(), RandomStringUtils, and Math.random(), to meet these requirements.
Pre-requisites
1. Passay
Passay is a Java-based toolkit for creating and validating passwords. It is extremely flexible and offers a complete feature list for validating/generating passwords
i) CharcterCharacterisitcsRule Compared to previous rules, CharacterCharacteristicsRule is more complicated. We must supply a list of CharacterRules in order to generate a CharacterCharacteristicsRule object. Additionally, we need to specify the minimum number of password matches. This is how we can handle it:
Presentation of Characteristics Rule demands that a password contain three of the four rules offered
ii) generatePassword() The Passay library gives us the ability to generate passwords in addition to password validation. We can offer guidelines that the generator should follow.
We require a PasswordGenerator object in order to generate a password. We then use the generatePassword() method and supply the list of CharacterRules. This is an example of code:
We should be aware that in order to establish a CharacterRule, we require an object of CharacterData. The fact that the library gives us EnglishCharacterData is another intriguing feature. There are five sets of characters in it.
- digits
- lowercase English alphabet
- uppercase English alphabet
- combination of lowercase and uppercase sets
- special characters
Nothing, however, can prevent us from developing our cast of characters. Implementing the CharacterData interface is as simple as this. See how we can accomplish it:
2. RandomStringGenerator
i) generate()
creates a random string with the number of code points you provide.
Between the minimum and maximum numbers specified in the generator, code points are chosen at random. Despite the fact that the final string can contain pairs of surrogates that collectively encode an additional character, surrogate and private use characters are not returned.
It should be noted that if the string contains extra characters, the number of char code units created will be greater than the length. For more information about how Java stores Unicode values, consult the Character documentation
Parameters: length - the number of code points to generate Returns: The generated string Throws: IllegalArgumentException - if length < 0
Creates a random string with a range of code points between the minimum (inclusive) and maximum (inclusive).
The following parameters are required:
- minLengthInclusive - the number of code points to generate
- maxLengthInclusive - the number of code points to generate
3. RandomStringUtils
i) random
Generates a random string with the required amount of characters.
The set of all characters will be used to select the characters.
Parameters: count - the length of random string to create
Returns: the random string
ii) random numeric
Generates a random string with the required amount of characters.
The collection of numerical characters will be used to select the characters.
Parameters: count - the length of random string to create
Returns: the random string
iii) random Alphanumeric
generates a random string with the required amount of characters.
The set of Latin alphabetic characters (a-z, A-Z) and the numerals 0-9 will be used to select the characters.
Parameters: count - the length of random string to create
Returns: the random string
How are We Going to Build the Password Generator?
1) Using Passay For implementing password restrictions, Passay is one of the most well-known libraries. Using a set of customizable criteria, we create a password using the library. By utilizing the CharacterData implementation's default settings, we will be able to build the required password rules. In addition, we are provided with a unique CharacterData implementation according to your requirements.
2) Using RandomStringGenerator
By using the RandomStringGenerator in Apache Commons Text, it offers an additional method for creating secure passwords. With a predetermined amount of code points, the RandomStringGenerator creates Unicode strings. By using the Builder class of the RandomStringGenerator, we may use the RandomStringGenerator by generating an instance of the generator.
We can also modify the properties of the generator. One disadvantage of this approach is that, unlike Passay, we are unable to specify the number of characters in each batch. However, by mixing the output of other sets, we may get around this problem.
3) Using RandomStringUtils
A secure password can also be created using the RandomStringUtils class found in the Apache Commons Lang Library. Several techniques for creating a secure random password are available through the RandomStringUtils class. The class's random() method is crucial in the process of creating a password.
To better understand how the RandomStringUtils class can be used to create secure passwords, let's look at an example
4) Using a Custom Utility Method
The last approach, the Custom Utility Method, can also be used to construct a strong password. We use the "SecureRandom" class to create a special utility class. In this section, we construct four techniques for getting a character stream with the necessary length that includes numerals, capital letters, lowercase letters, and special characters. Then, we concatenate each of them to produce a secure password.
5) Using Math.random()
To return a pseudorandom double type number larger than or equal to 0.0 and less than 1.0, use the java.lang.Math.random() method. The random number generated by default was always between 0 & 1.
You must multiply the returned value by the magnitude of the range if you want a specific range of values. For instance, the resultant address needs to be multiplied by 20 in order to produce a random number between 0 and 20.
Syntax
Final Output
- Using passay
- Using RandomStringGenerator
- Using RandomStringUtils
- Using Custom Utility Method
Requirements
1. Using Passay
a) adding dependencies
In order to use Passay library we need to add the following dependency in the POM.xml file:
b) Setup Java This section will walk you through downloading and installing Java on your computer if you decide to set up your environment for the Java programming language. Please set up the environment by following the instructions listed below.
This link to Download Java will take you to Java SE, which is free. You then download the appropriate version for your OS system.
Run the .exe to install Java on your computer after following the on-screen directions to download Java. You must configure environment variables to point to the proper installation folders once Java has been installed on your computer.
i) Setting up the Path for Windows 2000/XP We are assuming that you have installed Java in the c:\Program Files\java\jdk directory:
- Click Properties from the right-click menu on My Computer.
- On the Advanced page, select the Environment variables button.
- Update the Path variable to include the location of the Java executable. Example, if the path is currently set to C:\WINDOWS\SYSTEM32, then change your path to read C:\WINDOWS\SYSTEM32;c:\Program Files\java\jdk\bin.
ii) Setting up the Path for Windows 95/98/ME We are assuming that you have installed Java in c:\Program Files\java\jdk directory
Edit the C:\autoexec.bat file and add the following line at the end − SET PATH=%PATH%;C:\Program Files\java\jdk\bin
iii) Setting up the Path for Linux, UNIX, Solaris, FreeBSD The location of the Java binaries should be indicated in the environment variable PATH. If you run into issues, consult your shell's documentation.
For instance, you would add the following line at the end of your.bashrc: export PATH=/path/to/java:$PATH
iv) Download Passay Archive Download the latest version of Passay jar file from Maven Repository
OS | Archive name |
---|---|
Windows | passay-1.6.1.jar |
Linux | passay-1.6.1.jar |
Mac | passay-1.6.1.jar |
v) Set Passay Environment
Set the PASSAY environment variable to the base directory location on your computer where the Passay jar is kept. Assuming we extracted passay-1.6.1.jar in the Passay folder using different Operating Systems, please see the instructions below.
OS | Output |
---|---|
Windows | Set the environment variable PASSAY to C:\Passay |
Linux | export PASSAY=/usr/local/Passay |
Mac | export PASSAY=/Library/Passay |
vi) Set CLASSPATH Variable Set the Passay jar location as the value for the CLASSPATH environment variable. The following assumes that you have placed passay-1.6.1.jar in the Passay folder on various Operating Systems.
OS | Output |
---|---|
Windows | Set the environment variable CLASSPATH to %CLASSPATH%;%Passay%\passay-1.6.1.jar;.; |
Linux | export CLASSPATH=CLASSPATH:PASSAY/passay-1.6.1.jar:. |
Mac | export CLASSPATH=CLASSPATH:PASSAY/passay-1.6.1.jar:. |
2. Using RandomStringGenerator
Import the following in order to implement RandomStringGenerator in our Java program to generate passwords
3. Using RandomStringUtils class in Apache Commons Lang Library
To manipulate the essential Java API classes, use the Apache Commons Lang 3 package. This support includes methods for handling strings, numbers, dates, concurrency, object reflection, and more.
To pull the Commons Lang 3 library from the main Maven repository, just use the dependency shown below:
4. Using a custom utility method
Import the following in order to implement custom utility method in our Java program to generate passwords
Building the Password Generator in Java
Using Passay
Three main parts make up the Passay API.
- Rule: one or more rules that make up the rule set for a password policy.
- PasswordValidator: A component of a validator that checks a password against a specified set of rules.
- PasswordGenerator: A generator element that generates passwords in accordance with a specified rule set.
A typical password policy includes a set of guidelines for determining if a password complies with company standards. Think about the following regulation:
- Length of the password should be in between 8 to 16 characters.
- A password should not contain any whitespace.
- A password should contains each of the following: upper, lower, digit and a symbol.
Now, let's see how we can implement it in Java for generating passwords
Here, we've first created a GeneratePasswordExample1 class for generating a secure and random password
In the main method we've then called the generateSecurePassword() method for generating a random password using the Passay library
The generateSecurePassword() method is then created with an aim of finding a secure password and returns it to the main() method
In generateSecurePassword() we create a character rule for lower case and upper case as
Character rule for digits and special characters is also created in a similar fashion
An instance of PasswordGenerator class is then created
generatePassword() method of PasswordGenerator class is called to get Passay generated password This passay generated password is then returned back to the main method()
Using RandomStringGenerator
You've already read about how we use RandomStringGenerator previously in this article, now to comprehend how we can create a secure password utilizing, let's look at an example. RandomStringGenerator.
Here we first create a GeneratePasswordExample2 for generating a random and secure password
Inside main() method, we call generateSecurePassword() method for generating random password using RandomStringGenerator() method
The RandomStringGenerator() method is then created with an aim of finding an 8-digit password and returning it back to the main method
Inside this method, a string with 2 special characters, numbers, upper and lower case are generated
A list of char for storing these are then created like
The shuffle() method is then used to shuffle the list of elements
A random string using list stream() and collect() method is then created as
RandomStringGenerator password is then returned to the main() method
The generateRandomSpecialCharacters() method is then created which returns a string of special chars of the specified length A string of special characters is then generated using Builder(), withinRange(), filteredBy(), build() methods like
generateRandomAlphabet() method is then created which either returns an upper case string or a lowercase string of a certain length based upon the boolean variable check
Using RandomStringUtils
To better understand how the RandomStringUtils class can be used to create secure passwords, let's look at an example.
A class GeneratePasswordExample3 is created for generating a random and secure password
Inside the main method generateSecurePassword is then called to generate random password using RandomStringUtils
The generateSecurePassword() method is created to find a secure 10-digit password and return it back to the main() method Strings are then generated as follows
They're then concantenated into a single one
A list of chars is created to store all the numbers, characters and special characters using
We then use shuffle() method to shuffle list elements
A random string is then generated by using list stream() and collect() method and is then eventually returned back to the main method
Using a Custom Utility Method
There is another last way to generate a secure password, i.e., by using Custom Utility Method. We use the SecureRandom class for creating a custom utility class. Here, we create four methods for getting a character stream of numbers, upper case letters, lower case letters, and special characters of the specified length. After that, we concatenate each one of them to generate a strong password.
Let's take an example to understand how we can generate a secure password using Custom Utility Method:
GeneratePasswordExample4 is created for generated a random secure password
main() method calls the generateSecurePassword() method to generate random passwords
This method is then created to find 10-digit secure passwords and a string is then generated with 2 numbers, special characters, upper and lower case characters
A list of chars is then created for storing and the shuffle() method is used to shuffle list elements
using list stream() and collect() method we generate a random string
getRandomSpecialChars() method is then created which returns a stream of special characters at the specified length
Inside this method, an instance of secure random is created The ints() method is used to get IntStream of special characters of the specified length This stream is returned to the main method
getRandomNumber(), getRandomAlphabet() method is created wich returns a stream of number as well as upper and lower case characters at specified length baaed on boolean variables and are then returned to main() method
Using Math.random()
Output
Here, in this program we've generated an OTP using Math.randon()
int randomPin is declared with an aim to store OTP, we typecast it to int since we're using Math.random() here which is supposed to return a decimal value, OTP's value is then returned and printed
What’s Next?
Let's discuss ways to improve upon this project:
1. Password Strength
Make a function that, based on the length of the password, returns the password's strength!
The length of the passwords should be passed to this function, which should then return one of three strings: weak, medium, or strong.
To determine whether to return weak, medium, or strong, use conditionals. Return weak for instance, if the length is less than 5.
At the end of your main procedure, print out the password strength.
As a clever proposal, you could build on our password strength function by, for instance, determining whether the password contains at least this many numbers.
You might also add symbols to your random password, as another possibility!
2. Hash the Passwords
For further security, you can also choose to hash the generated password. The process of hashing is the creation of a string from a message using a mathematical formula called a cryptographic hash function. There are many hash functions available, but for them to be secure, they must possess four key characteristics:
- Deterministic means that the same message should always produce the same hash when processed by the same hash algorithm.
- It cannot be reversed, and generating a message from its hash would be impracticable.
- It has high entropy, which means that even a tiny alteration to a message should result in a very different hash.
- Additionally, it avoids collisions: two distinct messages shouldn't generate the same hash.
Conclusion
- We've used a variety of techniques, including passy, Custom Utility Method, RandomStringGenerator(), RandomStringUtils, and Math.random() in this article to generate passwords securely and randomly
- The Passay library gives us the ability to generate passwords in addition to password validation
- A secure password can also be generated using the Custom Utility Method. We developed four ways for obtaining a character stream comprising numbers, capital letters, lowercase letters, and special characters that has the desired length. Then, in order to create a strong password, we concatenated each one of them.
- RandomStringGenerator in Apache Commons Text offers an additional method for creating secure passwords. By using the Builder class of the RandomStringGenerator, we may use the RandomStringGenerator by generating an instance of the generator.
- Three main parts make up the Passay API Rule, PasswordValidator, and PasswordGenerator.