Build a Password Generator with JavaScript
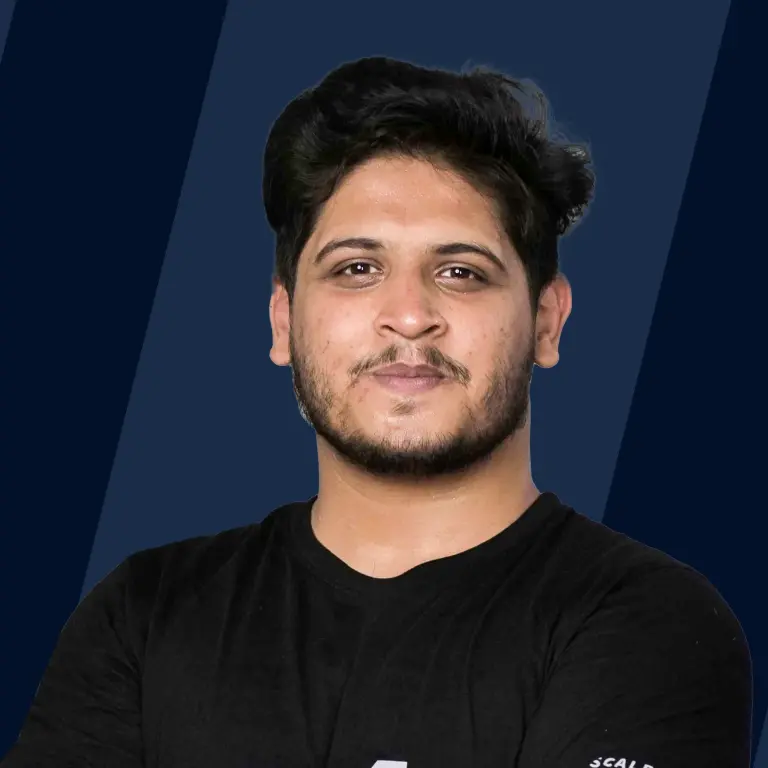
Overview
A Random Password Generator helps you generate a password that is strong enough to prevent your account from any kind of attack. A strong password generation can be done by having a combination of characters like letters, numbers, and symbols. The Random Password Generator app comprises:
- length setter for the password,
- choice among which of the characters to be included in the password, and
- copy button to copy the password for our use.
What are We Building?
In this article, we are going to build a simple yet customizable Random Password Generator using HTML, CSS, and JavaScript. It is up to us when we need to include a combination of letters, numbers, as well as symbols, or any one of them.
In order to prevent unauthorized access, we sometimes need a strong password for our accounts on different websites. It is easy to determine an easy password that has more often only letters. A strong password is always one with a combination of letters, symbols, and numbers.
Pre-Requisites
It is required to know the basics of HTML, CSS, and JS to keep up with the concepts that we'll use in this article. Here are some of the concepts to get started. These will be enough to make you understand the entire project that we're going to build.
- HTML tags
- CSS flexbox
- Padding in CSS
- CSS units
- Difference Between Var, Let, and Const in Javascript
- Difference Between == and === in Javascript
- Javascript functions
- Javascript Array
How are We Going to Build the Password Generator App?
We'll begin with creating the structure of the page with HTML. We are going to make use of div element, input fields like checkbox and number, and buttons.
Then, we'll style the page with CSS. We'll use flexbox properties as well. Moreover, to make the page responsive, media queries will be used.
To make it operational, we'll create functions to generate as well as copy passwords in JavaScript. We'll use objects and arrays in JavaScript.
We'll add some conditions to check if it fulfills the password criteria in the JavaScript code.
Final Output
This is what our final output would look like.
Building the Password Generator App using HTML, CSS, and JavaScript
Let's begin by creating the structure of the password generator with HTML. The first thing for an HTML page is to have the proper sequence to create the structure of a web page. Here's the initial code for reference.
The first thing we'll do is create a div element that will serve as a container for all the components of a password generator. So, we can add a straightforward heading. After the heading, there is a password box, which is enclosed by another div element. When the user selects the generate button, the password will be shown inside the inner div element of the password box.
Output:
Although the current code won't display these two nested div elements, we'll just put it for the time being. Moreover, you can check them by clicking on Inspect on the web page.
Now, since the password generator needs to be customizable, the user should be able to set the password length and the mixture of symbols, uppercase/lowercase letters, and numbers as well.
To begin with, there has to be a field to set the password length, for which, the <input type="number"> will be used. Also, the attributes, min and max will be used to define the lower and upper lengths for the password length. And for the default length, the value attribute will be used.
Output:
We'll also have multiple checkboxes to choose the combination of symbols, numbers, uppercase, and lowercase letters. By default, all these checkboxes will remain checked and users can uncheck one or more of these checkboxes to make their own combination. The checked attribute for the <input type="checkbox"> indicates that the <input> element will be checked already when the web page loads.
However, it is mandatory to keep at least one of these checkboxes checked, or else there won't be any character to include in the password. We'll alert the user if all the checkboxes are unchecked while writing the JavaScript code.
Output:
Note: We have put each of the checkboxes in their distinct div box to use flexbox properties so that the label and the checkbox could be put at the extreme ends of the container. For an idea, look at the image below.
Output:
Now, we are only left with adding the buttons to generate a password and copy it as well. We'll put these two buttons in a div element with class name buttons to further apply flexbox properties in our stylesheets file.
Output:
We are done with creating the structure of the page. This is just the skeleton. All it needs is a bit of styling to make it attractive. So, we need to work on the CSS part.
CSS To add styles to the HTML code, it should be first linked with the CSS file and we'll use a <link> tag to link it with an external stylesheet. The href attribute is where you need to put the name of the required CSS file.
The <link> tag is mentioned inside the <head> tag of the HTML code. Here, a stylesheet with the name password-generator.css has been created.
For the entire body, we'll pick up a font-family and choose a background for the entire page and color for the font as well.
Output:
We'll use attributes to set the border radius, padding, background color, and width of the container.
Below is the additional code to put the <div> container at the center of the page.
Here's the entire CSS code that we've written till now.
Output:
Since the <p> tags have been used for the description, they should be presented with a different color. Also, let's put the title at the center of the container horizontally.
Output:
Remember that we had created a password box in the beginning. We need to make it visible now. We'll also add padding to it so that the password is not aligned with the border of the box, but a little away from it.
For all the div elements inside the main container, we'll have to provide spacing at the top and bottom. Moreover, the justify-content will set the labels and checkboxes at the extreme ends.
Here is the complete CSS code that we have created so far.
Output:
Now, we need to work on the buttons. Let's use a flexbox to put the two buttons in the same row. Also, put justify-content to put the buttons at the two extreme ends.
Here's the CSS code written so far.
Output:
Now, the password generator looks impressive. However, we also have to make it responsive so that it adjusts itself according to the changing size of the viewport. We need to use media queries to define what happens when the width shrinks down. The respective elements will have to reduce their size accordingly.
Now, here's our final CSS code and the final output.
Output:
Since the styling of the page is done, we just need to make it work and actually perform what it was being made for. This can be done by providing it with JavaScript code.
JavaScript Create a JS file and link it with the HTML code by adding <script> tag inside the <head> tag in the HTML.
Firstly, there needs to be an object that contains four different strings, each for different types of characters (symbols, numbers, uppercase, and lowercase letters). The characters for the password will be generated by randomly selecting one character from each of these strings.
Now, how would the random selection take place? We'll have a function that randomly selects a character from each string. We'll have to create a function that randomly selects a character from 0 to stringLength - 1, where stringLength is the length of the string from the object. We'll use the following code for this purpose:
Math.floor(Math.random() * string.length) selects a random number in the range of 0 to stringLength - 1.
Create an array with four distinct functions so that you can pick one at random to fulfill the required password length.
From this array, there will be another random number to select one of these functions for each character of the password. But, we need to first fetch the boolean values from the checkboxes using document.getElementById(). For a checkbox, the HTML checkbox element will be accessed by the code given below. Also, it has to be ensured that at least one of the boxes has been left checked by the user. If not, alert the user to do the same and return the function.
Now, we need to access the password box as well as the required length set for password generation.
Along with it, we also need an empty string, pw, whose length will be incremented up to the set length for the password. We'll run a while loop until the length of string pw is equal to the required or set length for the password.
Till now, this is the entire code for the function generatePassword():
Now, any of the characters among numbers, symbols, uppercase, and lowercase letters should be randomly selected. So, another random number generation should be added to randomly select one of the functions from the array, getType. And these functions would, in turn, look for a random character in their respective strings from the object, types. Let us name this random number for function selection as typeAdder.
However, we need to make sure that the randomly selected function has its checkbox checked, or else it must not be included in the string, pw.
Finally, the string, pw, will be put in the password box.
Here's the final code for the function generatePassword():
Now that we have created the function, generarePassword(), we need to create a function to copy the password, copyPassword().
Create a temporary element to attach to the contect for the document. We'll access the textarea element which is a multi-line text input.
We need to access the generated password. If the generated password is empty, then return the function.
Attach the tempElement in the document. Return the entire text in <textarea> element. The following command copies the password to the clipboard.
Here's the code for the function copyPassword():
Here's the final JavaScript code:
Conclusion
- What's included in the Random Password Generator app?
-
- length setting for the password,
-
- character selection checkboxes for the password, and
-
- copy button to save the password for later use.
- The input fields in HTML will let you create checkboxes and length setter for the password.
- The random selection in JavaScript code will be done between 0 to length of the string - 1 by using:
- The random selection will be done among the JavaScript objects and JavaScript arrays.
- The following command will help us copy the password to the clipboard: