Pattern Programming in C++
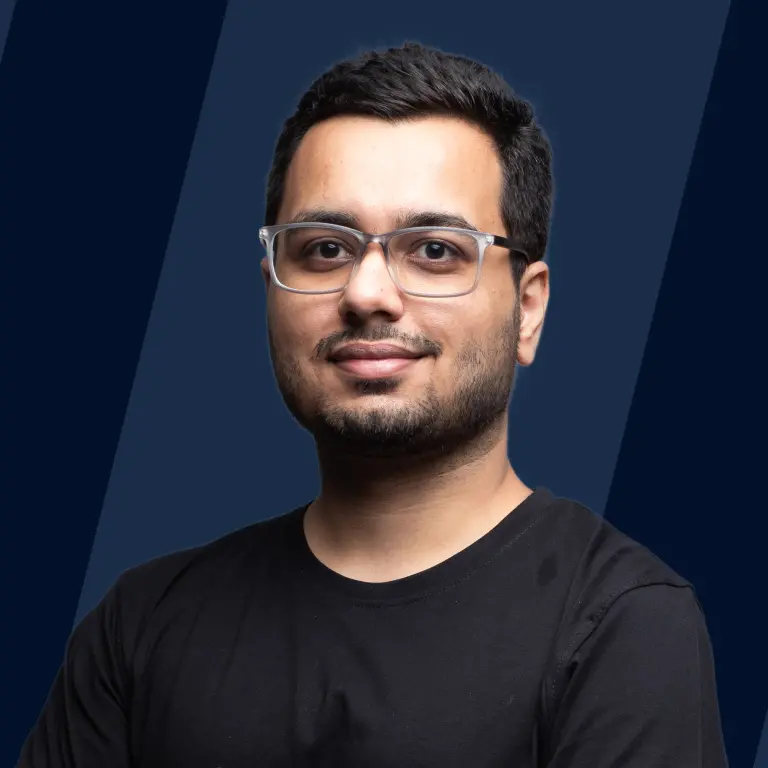
Introduction
Pattern programming means printing different patterns, such as triangles, pyramids, rectangles, etc., using a programming language.
Pattern programming in C++ requires a decent knowledge of loops or, more specifically, nested loops. Writing code(s) to print patterns using special characters, numbers, and alphabets makes one understand how the loops work internally.
- In this article, the code to print triangles, pyramids, and rectangles using *, numbers, and alphabets has been shown.
- Other complex patterns, like the Butterfly diagram and Pascal's triangle, have also been shown.
Top C++ Pattern Programs:
- Print triangles using *, numbers, and characters
- Rectangle Pattern
- Hollow Rectangle Pattern
- Inverted Half Pyramid
- Floyd’s Triangle
- Butterfly Pattern
- Print Pascal's triangle
- Print Full Pyramid Pattern of Stars
- Print Pattern of Stars
- C++ Print Triangle Star Pattern
- C++ Print Pattern of Numbers
C++ Programs for Important Patterns
Print triangle using "*", numbers, and characters
To print a triangle pattern program in C++, we will use two nested loops with iterable variables as i and j. The whole logic to print a triangle using x is as follows, where x can be *, number, and character:
- Take input from the user of the number of rows in the triangle. Let it be rows.
- Iterate for to and do :
- Iterate for to and print + space each time i.e cout << x << " ";
- Terminate the current line, i.e. cout << endl;
When we implement the above logic, the inner loop will print x 1 time in the first row, then two times in the second row, and so on.
Note: if we want to print the inverted triangle, the only change that is required is to change the first for loop such that we iterate from to , and we are good to go.
Code 1: Printing half pyramid using *
To print a half pyramid/triangle using *, we will replace the with *, and we are good to go.
Source Code:
Output:
Code 2: Printing half pyramid using numbers
Again to print a pyramid of numbers, we need to replace with , where denotes the row number. Here it is because we are starting from 0.
Source Code:
Output:
Code 3: Printing half pyramid using Alphabets
Similarly, we will replace the with the desired alphabets to print a pyramid of alphabets.
Source Code:
Output:
Rectangle pattern
Coding a rectangle pattern program in C++ is similar to printing a matrix of dimensions .
The logic is as follows :
- Take the input of rows and columns (dimensions) of the rectangle. Let them be rows and cols.
- Iterate from to and do the following :
- Iterate from to and print + space each time cout << x << " ";
- Terminate the current line, cout << endl;
Source Code:
Output :
Hollow Rectangle Pattern
The process to code the hollow rectangle pattern program in C++ is almost the same as printing a normal rectangle with the only difference that while printing a hollow rectangle, we need to take care of printing empty spaces for non-border cells.
The logic is as follows :
- Take the input of rows and columns (dimensions) of the rectangle. Let them be rows and cols.
- Iterate from to and do the following :
- Iterate from to and do the following :
- If current is the border cell of rectangle i = 0 OR i = rows - 1 OR j = 0 OR j = cols - 1 print * + space.
- Otherwise, print blank space.
- Iterate from to and do the following :
Source Code:
Output:
Floyd's triangle
In Floyd's triangle, we need to print numbers, but it is also required to increase the number by 1 each time we print it. Floyd's Triangle is similar to the half triangle using numbers, with the only difference being that in Floyd's triangle, we need to maintain a counter which will be incremented by one each time it is printed. The logic is as follows :
- Take input from the user of the number of rows in the triangle. Let it be .
- Define a variable, say and initialize its value to 0.
- Iterate for to and do :
- Iterate for to and do the following :
- Print + space each time cout << count << " ";
- Increase the value of count by 1 count++.
- Terminate the current line, cout << endl;
- Iterate for to and do the following :
Source Code:
Output:
Butterfly Pattern
To easily print the butterfly pattern in C++, we will split our code into two parts, i.e., print the upper part in the first go and then the second part.
The logic is as follows :
- Take input from the user of the number of rows in the Butterfly pattern. Let it be .
- Iterate from to and do the following -
- Iterate from to and print *+ Blank space cout << "*" << " ";
- Define a variable to find the number of spaces needed to be printed; say it to be .
- Initialize its value to be .
- Iterate from to and print blank spaces.
- Iterate from to and print *+ Blank space cout << "*" << " ";
- Terminate the line, count << endl;
- Repeat the above-given steps, but this time Iterate from to . This will print the lower part, as we have already printed the upper part.
Source Code :
Output:
Print Pascal's triangle
Pascal's triangle is a triangle that consists of a binomial coefficient (number of ways to choose things elements a set of elements). We can easily find the value of using pascal's triangle by looking at the value in the row.
The logic for printing pascal's triangle is as follows :
- Take input from the user of the number of rows in the triangle. Let it be .
- Iterate from to and do the following :
- Print spaces using a for a loop.
- Define a variable, say coefficient.
- Iterate from to and do the following :
- If , then set coefficient's value to be 1.
- Otherwise multiply (i - j + 1) to coefficient and divide it by j coefficient * = (i - j + 1) / j;
- Print coefficient + space i.e. cout << coefficient << " ";
- Terminate the line cout << endl;
Source Code:
Output:
Print Full Pyramid Pattern of Stars
Printing the full pyramid is almost the same as printing the half pyramid. We need to print space before printing the *s. The logic is as follows :
- Take input from the user of the number of rows in the triangle. Let it be .
- Iterate from to and do the following :
- Define a variable to find the number of spaces needed to be printed. Say it to be .
- Initialize its value to be .
- Iterate from to and print double spaces.
- Iterate from to and print *+space.
- Terminate the line i.e. cout << endl;
Source Code:
Output:
Print pattern of Stars.
The following code contains some of the pattern programs in c++ combined, which we have already seen in the previous sections of this article.
Source Code:
Output:
C++ Print Triangle Star Pattern
1. Simple triangle.
Code
Output
2. Inverted triangle.
Code
Output
C++ Print Pattern of Numbers
Code
Output
Conclusion
- All kinds of patterns can be easily printed by correctly using nested for loops (or while/do-while loops).
- i and j are the variables commonly used in nested loops to print patterns.
- To make it easier, code can be split into two parts, just like in the Butterfly pattern.
- In some pattern programs in C++, we should take utmost care of blank spaces to make the pattern.