PDB Python Debugger
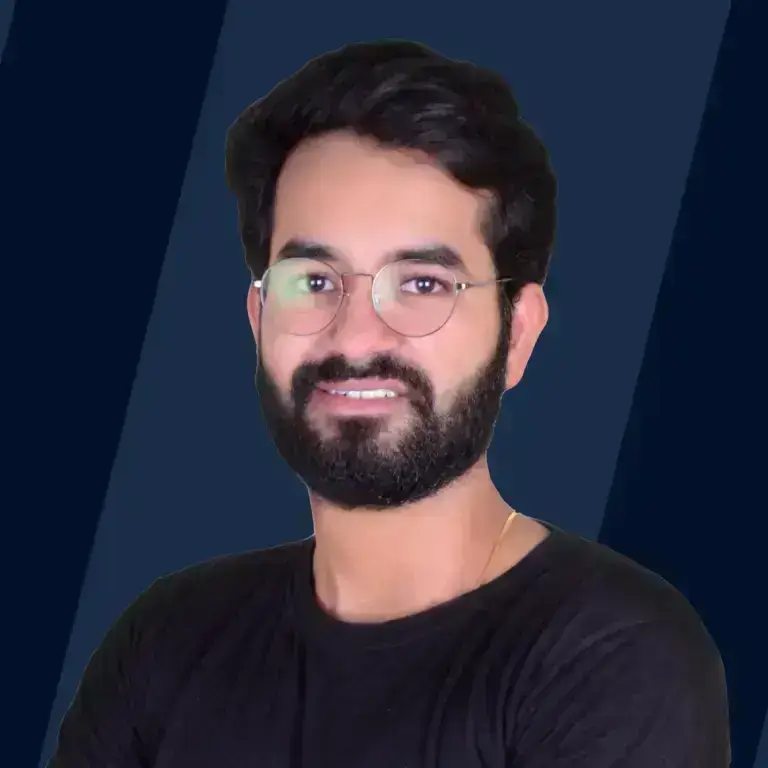
Overview
Debugging is a term frequently used in the language of software development to describe the process of identifying and fixing programmatic faults or so-called errors. The standard library for Python includes the PDB module, which is a collection of tools for debugging Python scripts.
So we will go over the fundamentals of using PDB, Python's debugger, in this article.
PDB Python Module
The Python debugger, or PDB module, is a built-in feature of the Python standard library that makes debugging in Python easier. It is specified as the class Pdb, which utilizes the cmd (support for line-oriented command interpreters) and BDB (basic debugger functions) modules internally. Pdb's main benefit is that it only operates on the command line, which makes it ideal for debugging code on remote servers when we don't have access to a GUI-based debugger.
PDB is compatible with:
- establishing breakpoints
- viewing the code
- list of the source code
- looking at stack traces
PDB Python Module Features
1. Printing Variables or expressions
When using the print order p, you are sending Python an expression to evaluate. If you supply a variable name, PDB will print its current value. However, there is much more you can do to assess the state of your running application. PDB debugging is used in the recursion to examine variables.
In this example, we'll use PDB trace to build a recursive function and examine the values of variables at each call. We'll use the straightforward print keyword along with the variable name to print the value of the variable.
Output
2. Moving in code by steps
That's the most important feature that is offered by PDB. The two primary commands for doing this are as follows:
n- within the same function, move on to the next line.
s- in this function or a called function, move to the next line
Let's use an example to further grasp how it works.
Output using n:
Output using s:
3. Using Breakpoints
With the help of this functionality, we can dynamically set breakpoints at certain lines in the source code.
Set a break at the line in the current file with a line number as an argument. Set a break at the function's first executable line using the function name. If a second parameter is given, it is a string that specifies an expression that, for the breakpoint to be respected, must evaluate to true.
To specify a breakpoint in a different file (perhaps one that hasn't been loaded yet), the line number may be prefixed by a filename and a colon. The .py suffix may be dropped as the file is found on the sys.path.
Output:
4. Execute code until the specified line
Output:
5. List the code
Listing out the source code is the other feature that is used to track the source code with a line number as a list. It uses the ll command for this. Let's use an example to better understand how longlists work.
Output:
6. Displaying Expressions
You can use the order show [expression] to tell pdb to immediately display the estimation of articulation, if it changed, when execution terminates, just like when printing articulations with p and pp. Clear a showcase articulation by using the order undisplay [expression] display [expression]
When execution halts in the current frame, show the expression's value if it changed. List every display expression for the current frame without an expression.
Remove the phrase from the current frame's display. Clear all display expressions for the current frame without using any expression.
Output
7. Frames up-down
Each trace can be used as a frame in this situation, and we can also switch between frames.
The most recent frame should be at the bottom of a printed stack trace. The "current frame," which establishes the context for most instructions, is shown by an arrow. This command's alias is "bt."
Increase the stack trace's current frame count (which is set to one) by one (to an older frame).
Lower the stack trace's current frame count, which is always set to one (to a newer frame).
Output
Starting Python Debugging with PDB Module
There are various methods to launch a debugger. You only need to import the pdb and pdb.set trace() commands to begin debugging the program. Run your script normally, and the breakpoint we set will cause the execution to end. Consequently, we are essentially hard coding a breakpoint on the line below when we execute a set trace (). Breakpoint(), a built-in function in Python 3.7 and subsequent versions, performs the same job. For information on inserting the set trace() function, see the sample below.
Example1: Debugging a Simple Python program of multiplication of numbers using the Python pdb module
Indentation Error: The program cannot multiply strings since input() returns a string. Thus, ValueError will be raised.
Output
The directory path to our file, the line number where our breakpoint is located, and the word "module" can all be found in the output on the first line following the angle bracket. In essence, it indicates that line 6 of x.py, the module level, has a breakpoint. If the breakpoint is added inside the code, its name will show up inside <>. The code line where the execution is interrupted is displayed in the next line. This line is not yet being executed. Using the following commands, we can now explore the code:
Command | Function |
---|---|
where | Show the current line's line number and stack trace. |
help | To show every command |
step | Enter the functions that were called at the current line. |
next | Ignore function calls and run the current line before moving on to the next one. |
Write whatis and the variable name to determine the variable's type. The output of type x is returned as a
Example 2: Checking variable type using pdb ‘whatis’ command
To determine the type of a variable, we can use the 'whatis' keyword and the variable's name (locally or globally defined).
Output
Example 3: Navigating in pdb prompt
Using the keys n (next), u (up), and d, we can move around the pdb prompt (down). Using the aforementioned instructions, we can explore and debug the Python code as a whole.
Output
Example 4: Post-mortem debugging using Python pdb module
Debugging in post-mortem mode refers to doing so after the program has completed its execution phase (failure has already occurred). The pm() and post mortem() functions in pdb provide post-mortem debugging. When one of these procedures detects an active traceback, it starts the debugger at the call stack line where the exception first appeared. When a program encounters an exception, you may see pdb appear in the output of the provided example.
Output:
Checking Variables on the Stack
The stack keeps track of all variables, including both global variables and local variables specific to the function being used in the program. To print all the arguments for a function that is presently active, use args (or use a). The p command evaluates an expression that is sent to it and outputs the outcome.
To demonstrate how to check for variables, example 4 is debugged here:
Output
PDB Python Breakpoints
We frequently wish to put a lot of breakpoints where we know problems might occur while dealing with large programs. You only need to utilize the break command to accomplish this. The debugger gives a breakpoint a number when you insert one, starting with 1. To view, all of the program's breakpoints, use the break command.
Syntax:
Output
Managing PDB Python Breakpoints
We may manage the breakpoints using the enable, disable, and remove commands after adding them with the help of the numbers supplied to them. Enable turns on the deactivated breakpoints while disable instructs the debugger not to halt when that breakpoint is reached.
The implementation to manage breakpoints using example 4 is provided below.
Output
Conclusion
- A pdb is an effective debugger. You can also utilize the PDB debugger from a Python script.
- Any breakpoint may be turned on or off using the disable/enable command, or it may be completely cleared with the clear command.
- The command line interface for the PDB module is quite user-friendly.