Perfect Number Program in C
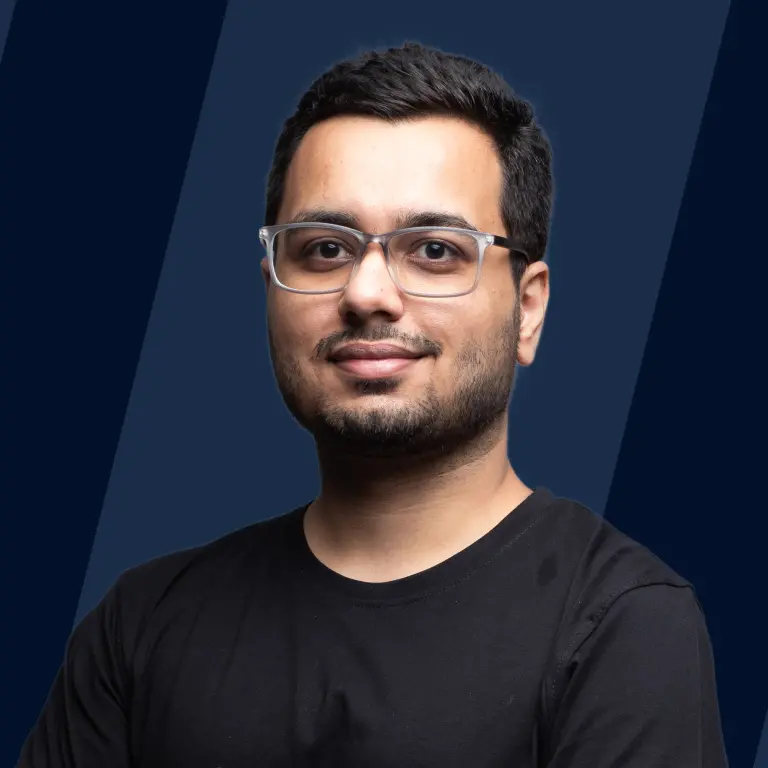
A perfect number in mathematics is an integer with a sum equal to its positive divisor, not including the number itself. This blog will cover all the details about perfect numbers using C.
Introduction
A perfect number program in c is a number whose sum equals its positive divisor but excludes the number itself. For instance, 6 is divisible by 1, 2, and 3. The number also divides itself, but the number divisible by itself is excluded for a perfect number. When the divisors are added, , the result is also 6, which equals the number considered. Hence, the number 6 is perfect.
C Program to Check Perfect Number or Not
Using for Loop
Program to check whether the number taken from a user is perfect or not in C
Output :
Explanation:
In the given example, the loop is iterated for every value of i. A remainder is calculated, and if it is equal to 0, then sum=sum+j. Out of the loop, there is a condition that checks whether or not the sum is equal to the number, and accordingly, the print statement is executed.
Using While Loop
Program to check whether the number taken from a user is perfect or not in C
Output :
Explanation:
In the given example, the while loop is iterated for every value of i. A sum is calculated. Out of the loop, a condition checks whether or not the sum is equal to the number, and accordingly, the print statement is executed.
Using Recursion
Program to check whether the number taken from a user is perfect or not in C using recursion.
Output :
Explanation:
In the given example, the function PerfectNum does the calculation and returns the sum. Out of the loop, a condition checks whether or not the sum is equal to the number, and accordingly, the print statement is executed.
C Program to Find Perfect Number between 1 to 1000
Output :
Explanation:
In the given example, a and b are variables used for iteration; the end is the last number. For instance, in 1 to 1000, 1000 is the end since it is the last number. It iterates from 1 to 1000, and at each iteration, it checks whether the number is perfect or not. If the number is perfect, then the print statement will be executed.
Conclusion
- In mathematics, A perfect number program in C is an integer with a sum equal to that of its positive divisor, not including the number itself. For instance, the number 6 is completely divisible by 1, 2, and 3.
- The number also divides itself, but for a perfect number, the number divisible by itself is excluded. When the divisors are added, 1+2+3, the result is also 6, which is equal to the number that is considered. Hence, the number 6 is perfect.