Pointer Arithmetics in C
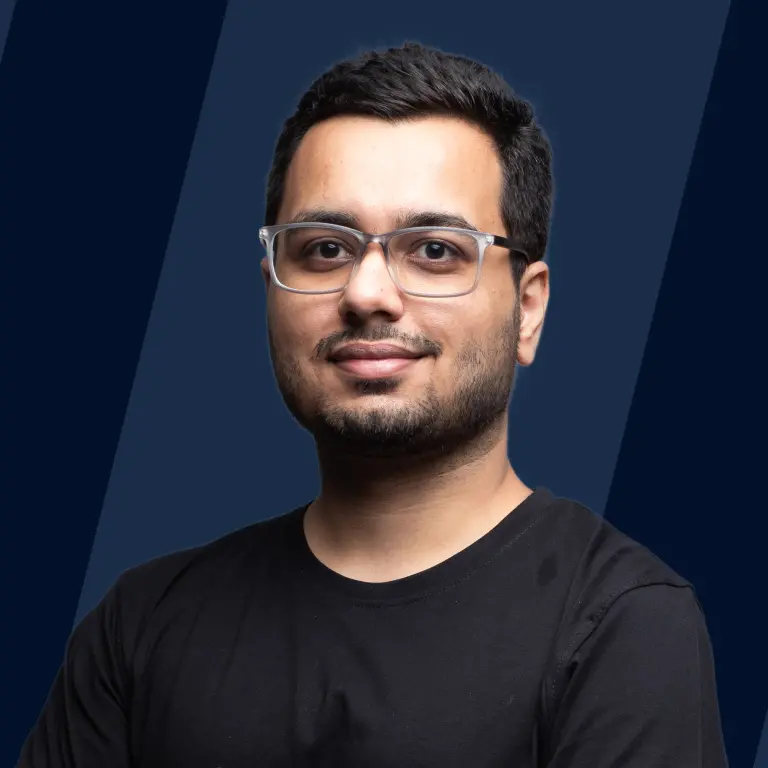
In C, pointer arithmetic is a fundamental concept that includes manipulating memory locations via pointers. It allows developers to execute numerous actions on pointers, boosting their ability to effectively maintain and explore data structures. Adding and subtracting integers to pointers are everyday pointer arithmetic operations that help navigate arrays and structures.
Increment/Decrement of a Pointer
In C programming, pointer arithmetic is a basic notion that allows you to manipulate memory locations contained in pointers. Understanding how to increment and decrement pointers will help you deal with data structures, arrays, and memory management much more effectively. In this section, we'll go into the complexities of pointer arithmetic, using explicit code examples to demonstrate the process.
Incrementing a Pointer
Incrementing a pointer means advancing its memory address to point to the next element of the same data type. This comes in handy when traversing arrays or linked lists. The syntax for incrementing a pointer is simple:
Example:
Let's consider an example using an integer array:
Decrementing a Pointer
Decrementing a pointer is identical to incrementing it, except it transfers it to the prior memory address. This is useful for traversing data structures backwards. Here is how to accomplish it:
For instance, using our integer array:
Pointer arithmetic is not limited to increments or decrements by one. You can adjust the pointer by a certain number of elements based on your needs:
Remember that pointer arithmetic should be performed within the boundaries of allocated memory to prevent accessing invalid memory locations.
Finally, knowing pointer arithmetic allows for efficient data manipulation in C. Increment and decrement pointers provide smooth navigation between arrays and linked structures. You may improve the efficiency and readability of your C programs by optimizing your code for memory traversal and manipulation using these basic yet effective approaches.
Addition of Integer to a Pointer
Pointer arithmetic is an important topic in C programming since it allows you to control memory addresses using integers. Adding an integer value to a pointer is a basic operation with major ramifications for memory management and data traversal.
Pointers in C have memory addresses as their values, indicating the location of a certain data piece. When an integer is added to a pointer, the pointer is advanced by the number of memory places equal to the product of the integer and the size of the data type to which the pointer corresponds. This operation is handy when exploring arrays, structs, and dynamically allocated memory.
Example:
Consider an integer pointer intPtr that points to the start of an integer array. Bypassing an integer value to an integer, the pointer is moved to another entry in the array based on the number of elements skipped.
For example:
Here, the pointer intPtr is advanced by two elements, bringing it to the array's third element (30).
Another use case is traversing memory chunks allocated dynamically using functions like malloc. For instance:
To avoid undefined behaviour and memory issues, pointer arithmetic should be performed inside the confines of the allocated memory.
Adding an integer to a pointer is a strong C method for speeding up array and memory block traversal. However, attention is required to maintain adequate memory management and avoid potential hazards. With the help of these code examples, you may now navigate the world of pointer arithmetic with more clarity, maximizing its potential for efficient data manipulation.
Subtraction of Integer from a Pointer
When handling pointers in programming, executing arithmetic operations may initially appear difficult. Subtracting an integer from a pointer is a frequent operation. This section will go into this notion, describing it in simple words and offering instructive code examples.
Pointers are used in C and C++ programming to retain memory addresses. Pointer arithmetic entails updating these addresses depending on the size of the data type to which they point. When subtracting an integer from a pointer, the integer value is considered an offset.
Subtracting an integer from a pointer essentially pushes the pointer backwards in memory by the computed offset. This can be handy for exploring arrays or controlling dynamic memory allocation.
Example:
Let's consider an array manipulation scenario using C++ to illustrate this concept:
In this example, the pointer ptr initially points to the array's 4th element (40) arr. Subtracting two from the pointer now points to the 2nd element (20). This showcases the essence of integer subtraction from a pointer.
Pointer arithmetic is a strong tool, but it has drawbacks as well. It is critical to prevent shifting pointers outside of their assigned memory range. Improper use might result in undefined behaviour and memory-related issues.
This pointer arithmetic approach has uses in memory management, particularly when dealing with dynamically created memory blocks. It also helps to optimize algorithms that entail traversing arrays backwards or executing particular calculations in a specified order.
Subtracting Two Pointers
When effectively manipulating data in programming, subtracting two-pointers is a must-have technique. This strategy may appear difficult, but it is a strong approach that may simplify and improve the performance of your code. In this post, we'll look at the complexities of subtracting two pointers, replete with code samples.
Subtracting two pointers fundamentally determines the distance between two memory addresses or array items. This is very handy when you must compute the components between two places. Subtract one pointer from another to do this. The outcome is an integer number representing the difference in memory addresses.
Example:
Consider an application in which you operate with an array. Calculate the amount of elements between two pointers by subtracting them. Let's look at a simplistic C++ code sample:
In this example, we generate two pointers (ptr1 and ptr2) that point to separate array members. We can tell there are two items between them by subtracting these points. This result demonstrates the basic notion of subtracting two points.
Beyond arrays, subtracting two-pointers may be used in a variety of circumstances. It is frequently used in dynamic memory allocation, linked list traversal, and other memory management and optimization tasks.
The allocated memory should be freed with free() when it's no longer needed to prevent memory leaks.
To summarise, subtracting two pointers is a technique for calculating the distance between memory locations or array members that is both simple and efficient. If you grasp this method, you may improve your programming abilities and write more streamlined, effective code. Remember that practice is essential. Experiment with various data structures and scenarios to understand how pointer functions.
Comparison of Pointers
Pointers are strong and necessary components of programming languages such as C and C++. They enable us to alter memory addresses directly, giving us better control and efficiency.
Example
- Declaration and Initialization of Pointers:
A pointer is defined by an asterisk (*) symbol followed by the data type to which it will point. The memory address of a variable is assigned to the pointer during initialization. As an example:
- Null Pointers:
Pointers can be assigned a specific value known as a null pointer, indicating that they do not refer to any valid memory location. This can help prevent unauthorized memory access.
- Pointer Arithmetic:
Pointer arithmetic is the process of increasing or decreasing a pointer dependent on the size of the data type to which it points. This is handy for traversing arrays or memory blocks.
- Comparison of Pointers:
Comparing pointers involves checking if they point to the same memory address. This is different from comparing the values they point to.
- Void Pointers:
Pointers with no associated data type are referred to as void pointers. They provide a method for storing and manipulating memory addresses without specifying the data type.
- Pointer to Pointer (Double Pointer):
A pointer to a pointer (double pointer) is valuable when dealing with functions that modify pointers because it allows you to pass a pointer to a function and have that function modify the original pointer directly. This is crucial in scenarios where you want to change the memory address a pointer points to or want to return a new pointer from a function.
For example, when dynamically allocating memory within a function and you want to ensure that the newly allocated memory is accessible in the calling code, you would use a double pointer. By passing a pointer to a pointer as an argument to the function, the function can update the original pointer, making the allocated memory accessible after the function call.
Here's an example:
In this case, allocateMemory takes a double pointer int **ptr, and by dereferencing it with *ptr, it modifies the original pointer value to point to the newly allocated memory. This enables you to manage memory and data effectively in functions while retaining changes in the calling code.
To summarise, pointers are flexible memory manipulation tools in programming. Developers may harness their power by knowing their different characteristics, such as declaration, null pointers, arithmetic, comparison, void pointers, and pointer to pointer. However, inappropriate usage can result in memory leaks, crashes, and defects. Therefore, pointers must be handled with caution. Experimenting with different code samples and eventually grasping pointer principles will improve your programming and problem-solving abilities.
Pointer Arithmetic on Arrays
Arrays are sturdy data structures in C programming, essential for organizing collections of items. The subtle interplay with pointers, known as pointer arithmetic, gives arrays their power. This deft manipulation enables programmers to explore arrays efficiently, unfurling a tapestry of possibilities. In this research, we debunk pointer arithmetic on arrays using illustrated examples to shed light on its mechanisms.
A pointer is a variable that stores the memory address of another variable. When it is used with arrays, it produces a symphony. Consider the following scenario:
Here, we create a pointer, ptr, that takes on the identity of the array's first element. It explores the array neatly using pointer arithmetic (ptr++), with each step going to the next element's doorstep.
The use of pointers goes further into dynamic memory allocation. Here's an example:
This embodiment underscores pointer arithmetic’s role in memory management and element assignment within dynamically allocated arrays.
What makes pointer arithmetic powerful is its compatibility with multiple data types. Behold its prowess with a character array:
The text dances in front of us as the pointer pirouettes rhythmically through the character array, ending on the null terminator.
However, extreme vigilance is required. Mishandling pointer arithmetic can result in undefined behaviour and havoc with memory. It is necessary to walk carefully, verify limits and keep order.
In conclusion, pointer arithmetic on arrays in C has a significant impact. It exudes elegance, strength, and refinement, gracefully connecting pieces and memory addresses. A new chapter develops with each stride of the pointer, showing the labyrinthine beauty of array manipulation in C programming.
Conclusion
- Pointer arithmetic enhances memory navigation, allowing developers to manipulate bytes and data structures precisely for in-depth operations.
- It simplifies array traversal by enabling efficient element-to-element movement through arithmetic operations, which is crucial for tasks like sorting and searching.
- The impact of pointer arithmetic operations depends on the pointed data type, such as moving by an integer size with int* or a single byte with char*.
- It is essential to understand pointer increments to prevent errors like unintentional memory access or segmentation faults.
- While pointer arithmetic facilitates comparisons and complex data structure creation, misuse can lead to undefined behavior and challenging bugs.