Pointer to Structure in C
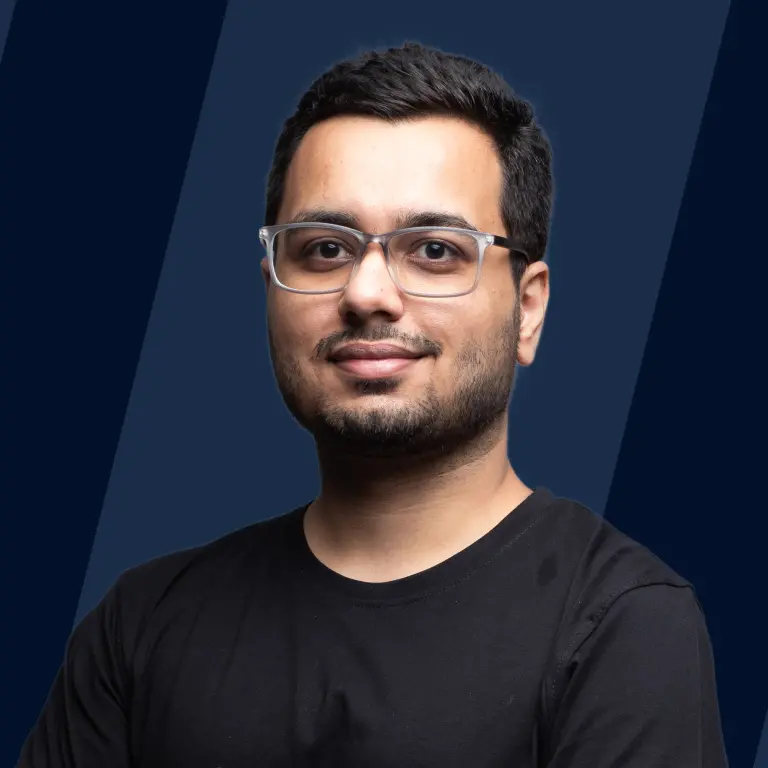
Overview
As we know Pointer is a variable that stores the address of another variable of data types like int or float. Similarly, we can have a Pointer to Structures, In which the pointer variable point to the address of the user-defined data types i.e. Structures. Structure in C is a user-defined data type which is used to store heterogeneous data in a contiguous manner.
Introduction
Before Declaration of Pointer to Structures, let's First define what is pointer and structure in C language.
What is Pointer in C ?
The Pointer is a variable which points to the address of another variable having the same data type as that of the pointer variable. The image completely explains the basic pointer which is required for this article.
In this image, we have a variable named a that currently stores an int type value which is equal to 44. Now we are curious to know what is the address of variable a ? To find out the address of variable a, we defined a pointer variable named b which is the same type as of a. To store the address of a in b, we use AMPERSAND OPERATOR (&) i.e. b=&a And by DEREFERENCE OPERATOR (*), we can easily find out the value stored at an address as shown in the image.
What is Structure in C ?
The Structure is a User-defined data type which is a collection of heterogeneous data which means structure allows us to group different data types and store them in a contiguous manner.
Example
What is Structure Pointer in C ?
We have seen how to create structure in C language using struct keyword. So creating a pointer to a structure in C is known as Structure Pointer.
This is our structure for a student in which id is the student roll number, name is the student name and age is the student's age.
So how to create a structure pointer to student ?
Syntax :
Note: No parenthesis in the above syntax. These are just added for better understanding.
Example :
However, the declaration for a pointer to structure only allocates memory for pointer NOT for structure. So to access the member of structure, we must allocate memory for structure using malloc() function and we could also give the address of the already declared structure variable to the structure pointer.
Memory allocation using malloc() function in C
malloc() function is the core function in C for memory allocation in C that takes a single integer argument defining the number of bytes to be allocated and returns the pointer to the first byte of allocated space. To allocate the memory for the struct object, we should call the sizeof() operator.
See in example for better understanding.
So, here malloc allocates memory dynamically, which means we can add struct blocks as many as we want. And it's the better way of allocating memory to user-defined data structures.
Initialization of The Structure Pointer
Initialization during the time of the creation of pointer
The above piece of code is declared a pointer to structure as well as initialised it.
Initialization after creating Pointer :
This is how we initialize a pointer to structure either time of creation or after creation. Both ways are considered correct.
Accessing Pointers to Structures (With Examples)
To access members of the structure using a pointer, we have two methods :
- Using Indirection Operator(*) and Dot (.) operator
- Using Arrow Operator (->)
Using Indirection Operator (*) and Dot Operator (.)
Indirection Operator is used to dereference the value present at a particular memory address. And the dot operator is used to access the data members of a structure.
Let's take an example for better understanding
Let's understand the above piece of code. Here we create a data type that stores information of an Employee. We create an object of Employee employee1 and a pointer ptr which points to the address of employee1 (according to line 14). So, according to the indirection operator, *ptr is same as employee1. which means *ptr can access the member of structure Employee followed by Dot operator.
Therefore,
(*ptr).name refers to the name of employee1. (*ptr).id refers to the id of employee1.
Note: The brackets are important before the Dot operator with the ptr variable because the dot operator has high precedence than the indirection operator.
Using Arrow Operator (->)
To access the members of the structure, we have another method ARROW OPERATOR METHOD. And using this operator, we could directly access the data members of struct and its don't need dereferencing (see example 2).
Syntax : pointer_name -> member_of_structure.
Example :
This method is more intuitive and easy to use as well as understand. With this method, code is more readable and understandable.
Dynamic memory allocation of Structures
Let us understand how to store the information entered by the user Dynamically with the help of an example. Here dynamically means we can change the size of the data structure at runtime.
Example
This program Dynamically Stores the information of entered students. To create a dynamic structure, we use here malloc() function. The syntax is simple just pass the number of student records we want to store and sizeof the struct. It will create that much of the amount of memory in a heap for us.
Note: *(ptr+i) is just a way to access the ith element of dynamically structured blocks.
Note: Don't forget to free the ptr. If we don't do that it will lead us to memory leaks.
Output :
Why Use it ?
Because Dynamic allocation is better as we can decide the number of blocks at the run time. It allows us to create more complex data structures such as Binary Tree and LinkedList.
Drawback of Using malloc :
The major drawback of using the malloc() function is that when we allocate memory using the malloc function, it is initialized our structure with some garbage values and if we forget to initialise the structure it will lead us to some undefined behaviour.
Solution to Drawback :
Instead of using malloc(), we can use calloc() function in C which is another core function for memory allocation in C. The main advantage of calloc() over malloc() is that calloc() initialized our structure with zero instead of some random garbage value.
Examples
Now it's time to write some neat code. Here we gonna discuss two examples one of them is related to how to access the structure pointer using INDIRECTION OPERATOR (*) AND DOT OPERATOR (.) and the other one consists of How to access structure pointer using ARROW OPERATOR (->).
Example 1
In this example, we are going to learn how to use the indirection operator (*) and dot operator (.) to access the pointer to structure in C.
This is the simplest program that shows how to use the indirection operator and dot operator to access the member of structure in C.
Output :
Example 2
The given example teaches us how to use Arrow operator (->) to access the pointer to structure in C.
The above program illustrates how Arrow Operator is used to access the member of structure. Like above said please don't forget to free the pointer after use otherwise it leads us to a memory leak of the program. Also, free the pointer after their use is good programming style.
Output :
Summary
- Structure enables us to group different data types and a pointer is used to store or to point to the address of variables. Creating a pointer to structure in C is known as Structure to pointer in C.
- With arrow operator (->) and indirection(*) & dot operator(.), we can access the members of the structure using the structure pointer.
- Initialization of a pointer is like initialization of a variable.
- Using the malloc() function, we can create dynamic structures with the help of pointers.