pow() Function in C++
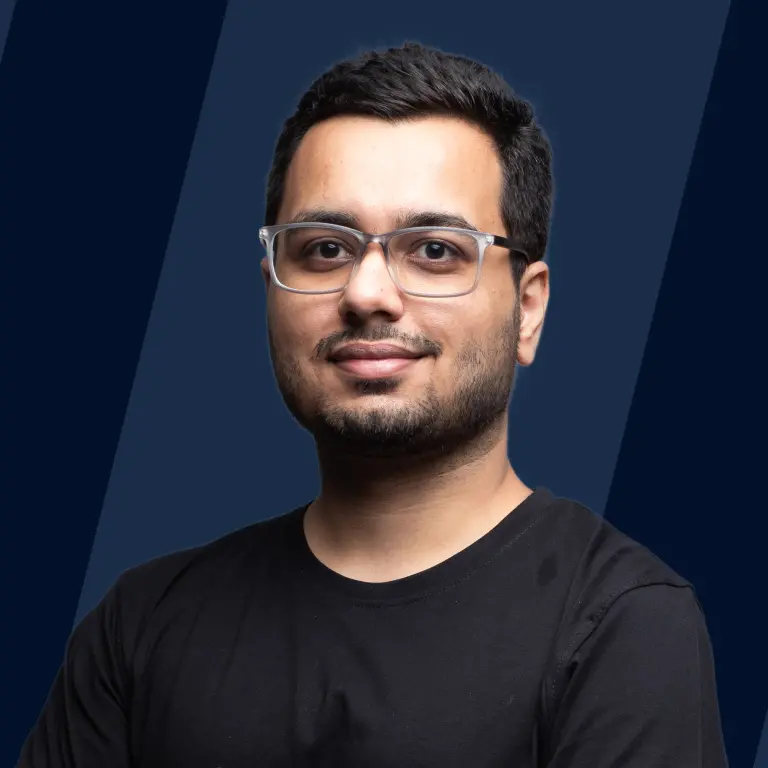
The pow() function in C++ is a mathematical function used to calculate one number raised to the power of another. It is part of the <cmath> header file, which is the C++ standard library’s math header. The pow() function is defined in the std namespace.
pow() Function Syntax
Syntax of pow() in C++ is
pow() Function Parameters
pow() accepts 2 parameters: base and exponent
Base: The base number indicates the number being multiplied.
Exponent: The exponent indicates the number of times the base number is multiplied.
pow() Function Return Value
The return type of pow() in C++ is double, and we get as a result.
pow() Function Prototypes
The pow() function in C++ is overloaded to handle different types of arguments. This means that there are multiple prototypes available for this function, allowing it to work with various data types. Here are some of the commonly used prototypes for the pow() function:
1. Standard Prototype
This is the standard and most commonly used prototype of the pow() function. Both the base and the exponent are of type double, and the function returns a result of type double.
2. Float Prototype
In this prototype, both the base and the exponent are of type float, and the function returns a result of type float.
3. Long Double Prototype
This prototype is used when the base and the exponent are of type long double, and it returns a result of type long double.
4. Integer Exponent Prototype
This prototype is particularly useful when you want to raise a number of type double to an integer power. The function returns a result of type double.
5. Float Base and Integer Exponent Prototype
This prototype allows you to raise a number of type float to an integer power. The function returns a result of type float.
6. Long Double Base and Integer Exponent Prototype
This prototype is used when you have a base of type long double and you want to raise it to an integer power. The function returns a result of type long double.
pow() Function Examples
If Base=0, We Get the Result as 0.0
This is because if we multiply 0 any number of times, it will remain 0 only. (any number of times), our result remains zero.
Output:
If Exponent=0, We Get the Result as 1
In mathematics, any number raised to the power 0 is 1.
Output:
If Both Base and Exponent are Non-zero, the Result is Also Non-zero
Here both base and exponent are of type double, and we get base raised to the power exponent as our result.
Output:
pow() in C++ with Different Arguments
First, we have the base as a long double and the exponent as an integer. And in the next case, we have both base and exponent as float.
Output:
Working of pow() Function with Integers
The pow() function in C++, primarily designed to work with floating-point numbers, also provides flexibility to work with integers. This is particularly useful for scenarios where you need to raise an integer to a power. Here’s how it works:
1. Integer Base and Exponent
When both the base and the exponent are integers, the pow() function will implicitly convert them to double types to perform the operation. The result will be of type double, which can be then explicitly cast back to an integer if required.
2. Integer Exponent with Floating-Point Base
You can also use the pow() function with an integer exponent and a floating-point base. This is particularly useful when you need to perform exponential calculations with a fixed exponent.
3. Precision Considerations
While working with integers and the pow() function, it’s important to be mindful of precision. The result of the pow() function is a floating-point number, and converting it back to an integer type might lead to loss of precision.
4. Performance Aspects
Using integers with the pow() function can sometimes be more performant, especially when dealing with large exponent values, as integer arithmetic is generally faster than floating-point arithmetic.
5. Handling Large Results
When raising an integer to a high power, the result can quickly become very large, potentially leading to overflow. It’s crucial to ensure that the result can be safely stored in the chosen data type.
Conclusion
-
The pow() function in C++ offers extensive functionality, allowing developers to perform exponential calculations with ease. Its multiple prototypes cater to different data types, ensuring flexibility across various applications.
-
With specific prototypes for float, double, and long double, the pow() function ensures that calculations are carried out with the appropriate precision, catering to the needs of different numerical computations.
-
The function integrates seamlessly into C++ programs, providing a straightforward syntax and predictable behavior that benefits both novice and experienced programmers.
-
It’s crucial for users to be aware of and handle special cases, such as negative bases with fractional exponents, to ensure accurate and reliable results.
-
While pow() is designed for performance, selecting the correct prototype and understanding its behavior are vital for optimizing computations, particularly in performance-critical scenarios.