Prepared Statement Java
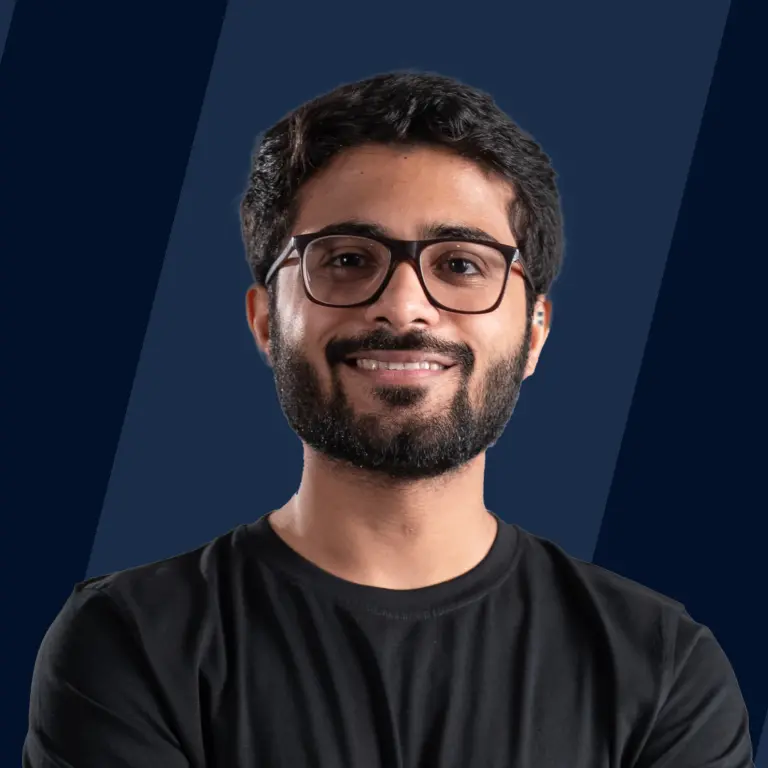
Overview
Prepared Statement is a SQL statement that is pre-compiled. It is used because it prevents SQL injection attacks. In this blog, we will be learning about PreparedStatement in Java, its use and methods, etc.
What is a Prepared Statement in Java ?
Prepared Statement is a pre-compiled SQL statement. It is a sub-interface of the statement in java. It has valuable features which are in addition to that of objects in a statement. The object of the Prepared Statement has the feature of executing parameterized queries instead of hard coding.
The example of a parameterized query :
Why do we Use Prepared Statements in Java ?
The main reason for using Prepared Statement is because it improves the performance of the application. This is because it uses an interface in which the query is compiled only once.
Here are the following advantages of using Prepared Statement :
- Prevent SQL injection attacks.
- When the Prepared Statement is created, the parameter that is passed is the SQL query. It contains pre-compiled SQL query.
- The same Prepared Statement can be used with different parameters while executing of the statement.
Methods of Prepared Statement Java
Following are the methods of PreparedStatement in java :
- setInt(int, int) :
it is used for setting the value of integer at a given index in the parameter. - setString(int, string) :
it is used to set the value of a string at a specified index given in the parameter. - setFloat(int, float) :
It is used to set a float value at a specified index - setDouble(int, double) :
It is used to set a double value at a specified value. - executeUpdate() :
It is used to create drop, update, insert and delete etc. The return type is int. - executeQuery() :
It is used for returning instance of ResultSet when a query is selected.
Steps to Use Prepared Statement
Here are the following steps to use PreparedSatement :
-
Creating a connection to the database :
-
Preparing the statement :
instead of hard coding the queries of SQL like : -
Select the parameters of placeholders, use ‘?’ for example :
-
The whole prepared statement looks like this :
-
Setting up the values of parameter :
-
Execution of the query :
Examples of Prepared Statements in Java
To understand this, create a table in SQL as given :
Now that the table is created, let's insert the values in the table using the code given :
Output :
Explanation :
In this example, all the main code is written in the try block. First, we initialize the class of oracle driver that we will be using in the code. Once this is done, a connection would be set up by using the oracle driver. The query of PreparedStatement is written and executed. Once executed successfully, the message will be printed, or else error.
Example of PreparedStatement for deleting a record :
Output :
Explanation :
Query of PreparedStatement is written for deleting a record from SQL in java. The query is executed and the message is printed successfully.
Advantages of Prepared Statement in Java
Here are the following advantages of using Prepared Statement :
- Prevent SQL injection attacks.
- When the PreparedStatement is created, the parameter that is passed is the SQL query. It contains pre-compiled SQL queries.
- The same PreparedStatement can be used with different parameters while executing of the statement.
Conclusion
Here are a few key takeaways from this blog :
- PreparedStatement is a SQL statement that is pre-compiled.
- It is a sub-interface of the statement in java.
- The main reason for using Prepared Statement is because it improves the performance of the application.
- Method for prepared statement :
- setInt(int, int)
- setString(int, string)
- setFloat(int, float)
- setDouble(int, double)
- executeUpdate()
- executeQuery()
- The example of a parameterized query :