Pretty Print Dict Python
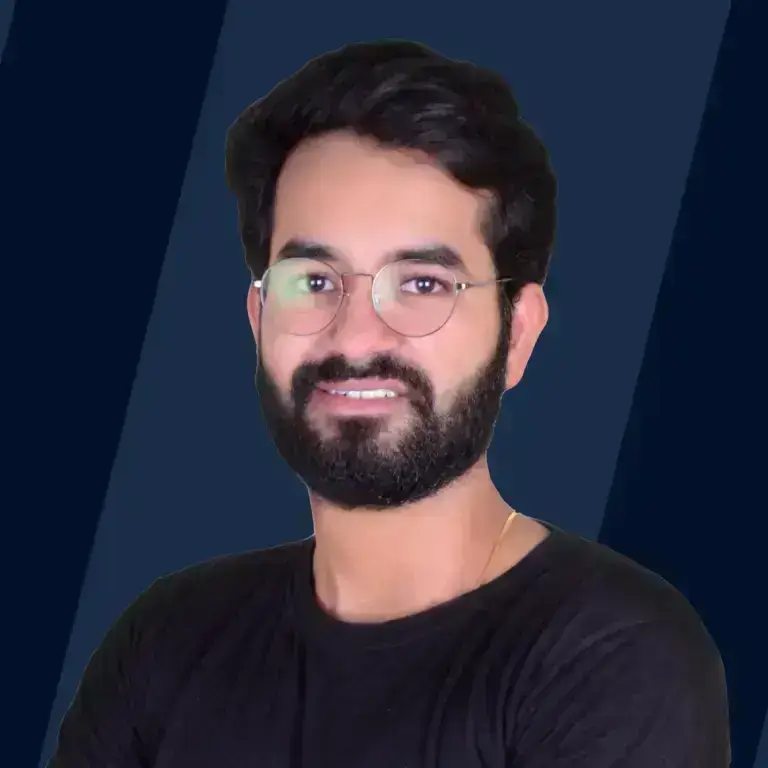
Overview
"Prettyprint” is defined as a process where the source code or any other item is displayed in an attractively presentable way. In the below article, we will learn about the concepts of pretty print dict Python with three different methods to implement it. We can pretty print dict Python by importing the 'pprint' module in Python which gives the capability to “pretty-print” random data structures in a much more presentable form that can also be used as input to the interpreter.
Introduction
One of the most frequently used data structures is the Dictionary in Python. The Dictionary can be defined as a collection of key-value pairs that hold and retrieve data. This dictionary stores data values such as maps. The keys are unique, immutable data types (like strings or integers), whereas values are made up of anything even additional dictionaries.
A simple example of how a dictionary in Python looks like given below:
To retrieve a value, we need to call the value by specifying the unique key of the dictionary. Suppose we want to retrieve the value for the name, we can simply call it as below:
Output:
As seen above, we called the unique key 'name' for which we got the output as 'harry' by implementing the get method.
Dictionaries in Python play a key part when working with data, such as data loaded from the internet. The JSON response from the APIs is very similar to the formatting of dictionaries in Python. We can easily store and retrieve data from dictionaries whereas sometimes it can get a bit tricky to read them. And with this problem statement in mind, Python introduced its "pprint" module which is helpful to print dictionaries in a human-readable, attractive, and presentable format.
Pretty Printing a Python Dictionary
After understanding dictionaries in Python and the problem statement where we identified that reading dictionaries can sometimes get very tricky, let us understand the concepts around pretty print dict Python and how it can help solve the problem of making the dictionaries much easier to read and understand.
We have an in-built library in Python called pprint widely known as pretty-print module. As the name suggests, this module is used whenever we want to print out the data structures dictionaries in a more formatted and presentable format. In this article, we are focusing on printing dictionaries with pretty print dict Python whereas many other data structures can be used to print them as well in a much more human-readable format.
Syntax:
The syntax for pretty print dict Python is as below:
Parameters: We have various parameters while we implement the pprint library that we can use for specifying various parameters such as:
indent: It is defined as the number of whitespaces that indents each line. The default value for the indent parameter is 1.
width: It is defined as the maximum number of characters allowed on a single line. The default value for the width parameter is None which means maximum.
depth: It is defined as the number of levels that shows when we use any nested data types. The default value for the width parameter is None which means we allow the program to show all levels of depth.
stream: It is defined as an output stream that is specified and is used to save a file.
compact: This refers to the values that shall be printed on single lines for a certain width when it's set to TRUE, else not.
sort_dicts: This refers to printing the key-value pairs in alphabetical order of the keys when set to TRUE which is the default value.
underscore_numbers: It is defined as a feature where long numbers can be segmented via an underscore symbol to make the. much more readable.
Example:
Code:
Output:
As seen above, we see that the output has the key-value pairs but reversed, it is so as the default value of sort_dicts is TRUE. This means that the key-value pairs are printed in the alphabetical order of the keys.
Hence we can say that the pprint module in Python is an extremely helpful utility module used to print data structures(in this case dictionaries) in a much more human-readable, presentable, and pretty way. This is a part of the standard library specifically used for debugging code where you need to work with API requests and collect large JSON files and data.
Methods of Pretty Print a Dictionary in Python
Below are the three methods through which we can pretty print dict Python where we shall see the working code example along with the algorithm, output, and elaborative explanation.
The three methods are:
- Using pprint()
- Using json.dumps()
- Using yaml.dump()
Let us dive into each of them:
Using pprint()
Intro:
The pprint() function of the pprint module in Python gives its users the ability to pretty print dict Python data types in a much more human-readable and presentable manner. Here we are dealing with a pretty-printing dictionary. We use the pprint() function to pretty-print any given string or object.
The pprint() output is much more human-readable as it breaks each element of the nested dictionary in the array format. It also sorts the dictionary's values with respect to the dictionary’s key.
QuickNote: To avoid sorting the key-value pairs of your dictionary, you simply need to set the sort_dicts parameter explicitly as False as the default value of the sort_dicts parameter is TRUE.
Algorithm:
- We start by importing the pprint module in Python.
- We create an array of dictionaries where we have various sections like Serial_No, Gender, Class_section, and Class_Attended for different serial numbers.
- We compare the print and pprint() functions by printing the array of dictionaries using both functions in Case 1 and Case 2 respectively.
Code:
Output:
Explanation:
As seen above, we implemented both the print() and pprint() functions by printing the array of dictionaries using both functions. We see that the output for the pprint() function is much more human-readable and easier to understand whereas the output for the print() function is not that clear to read. For implementing the pprint(), you need to import the pprint module first.
Using json.dumps()
Intro:
By importing the json module, we can make use of the dumps() function which helps to pretty much print dict Python. With the json.dumps() function we can convert the Python object into a JSON string. It also formats the dictionary into a much more human-readable and pretty JSON format. This is one of the most viable ways to pretty print dict Python as we first convert the dictionary into JSON and then sort the dictionary.
The json.dumps() function allows three parameters for pretty print dict Python, that are: the object(dictionary in this case) for conversion to Json, sort_keys with a boolean value (helps to specify that entries must be sorted by key) in addition to indent that helps to add these whitespaces into the formatting making the output look much more human-reading and presentable.
Algorithm:
- We start by importing the json module in Python.
- We create an array of dictionaries where we have various sections like Serial_No, Gender, and Class_Attended for different serial numbers.
- We compare the print and json.dumps() functions by printing the array of dictionaries using both functions in Case 1 and Case 2 respectively.
Code:
Output:
Explanation:
As seen above, we implemented both the print and dumps() functions by printing the array of dictionaries using both functions. We see that the output for the dumps() function is much more human-readable and easier to understand whereas the output for the print function is not that clear to read. For implementing the json.dumps() method, you need to import the JSON module first. We also specified the sort_keys as 'TRUE' which sorted the dictionary as well as specified the indent as 2 which made the output appears so human-readable and easier to understand.
Using yaml.dump()
Intro:
Lastly, we will implement the dump() function by first importing the YAML module. This yaml.dump function is similar to json.dumps() function where in YAML.dump() convert the dictionary in YAML format. And then. formatting it when its various parameters are switched to pretty print dict Python.
The yaml.dump() function allows three parameters for pretty print dict Python, which are: the object(dictionary in this case) for conversion to Yaml, sort_keys with a boolean value (helps to specify that entries must be sorted by key) in addition to the default_flow_style which is used to specify if the dump’s output style must be inline or in a block. Here the block style is considered to be much more readable and presentable. Hence, we always see this parameter as False.
Algorithm:
- We start by importing the YAML module in Python.
- We create an array of dictionaries where we have various sections like Serial_No, Gender, Class_section, and Class_Attended for different serial numbers.
- We compare the print and yaml.dump() function by printing the array of dictionaries using both functions in Case 1 and Case 2 respectively.
Code:
Output:
Explanation:
As seen above, we imported the yaml module and implemented the yaml.dump() function to pretty print dict Python along with printing the dictionary using the print function. The output for the dump() function is much more human-readable and easier to understand. We specified the sort_keys as False which is why the dictionary gets printed the same way and set the default_flow_style as False too which formatted it into readable and presentable YAML format.
Uses of Pretty Print a Dictionary in Python
The most predominant use of the pprint function of the pprint module is to make the data structure (here dictionaries) much more human-readable, presentable, and pretty way. This helps the user of the module to easily do his/her job when the work revolves around gathering data through API responses in long json formats. Storing and retrieving data in dictionaries is easier whereas sometimes it can get a bit tricky to read them. And with this problem statement in mind, Pytho introduced its "pprint" module which is helpful to print dictionaries in a human-readable, attractive, and presentable format.
FAQs
Below are some of the frequently asked questions about pretty print dict Python:
Q1: How do you pretty print dictionary values using which modules and functions?
A: The module used is the pprint module within which we have the pprint function of the same name. The function can be implemented to pretty-print the specific string or object. You first need to declare an array of dictionaries after which you can implement the pprint function to pretty print that string or object.
Q2: What is the difference between print and Pprint in Python?
A: We use the print function to simply print anything in Python in a single line. Whereas we implement the pprint() function in Python to print the data which are nested in a much more human-readable format.
Q3: Can we pretty print nested dictionaries using the pprint() function?
A: No, we can't. Although the pprint() function of the pprnt module print the dictionary in a much more human-readable, presentable, and pretty way. This is not the solution to pretty print any nested objects, such as nested dictionaries. Hence, when your values are nested, then the pprint() function is not the solution for it.
Conclusion
- The pprint module in Python is an extremely helpful utility module used to print data structures(in this case dictionaries) in a much more human-readable, presentable, and pretty way. This is a part of the standard library specifically used for debugging code where you need to work with API requests and collect large JSON files and data.
- The output from the YAML dump() function is much more readable and presentable than from the JSON dumps() function. Whereas, it's correct to say that both functions beat the output from the pprint function. This is so because the pprint function is not good enough to handle complex and nested data structures.