Program to Print Array in C
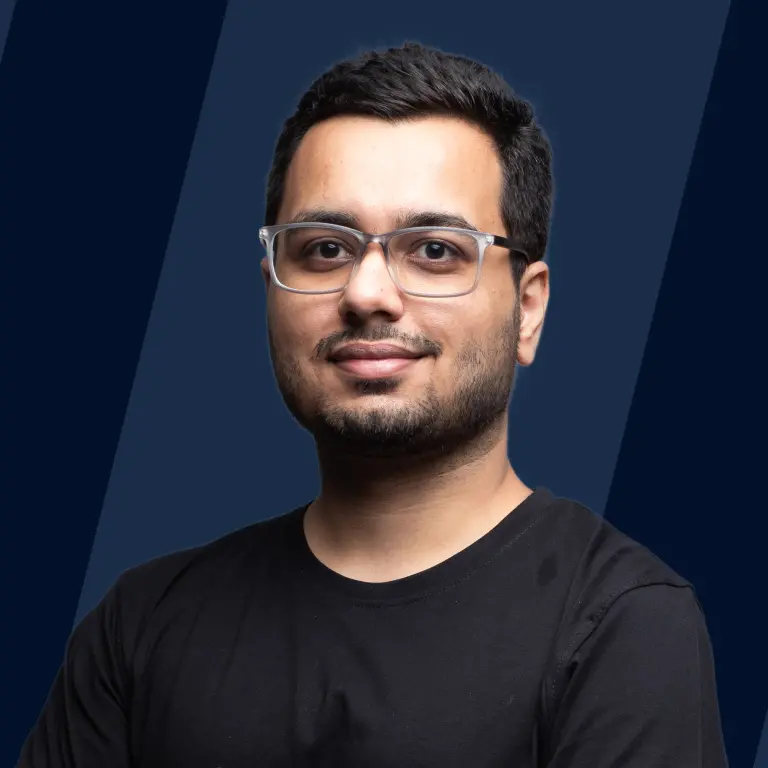
Overview
This article explores the different methods of printing an array in C. This article contains ways to print an array using for loop, while loop, do-while loop, etc. Apart from that, we will also take a look at printing an array using recursion and functions in C.
Introduction
Printing an array in C involves outputting the elements of an array in the console in order. Printing an array gives us a look into the contents of an array. This can be achieved in various ways, all of which we will discuss after taking a look at the algorithm.
Algorithm
Let us take a look at the low-level algorithm that we will be using to print array in C.
Assume we have an array arr[].
START
- Step 1 → Take the array arr[] and define its values, that is define the value for each element of the array arr[].
- Step 2 → Start a loop for each value of the array arr[].
- Step 3 → Print the value associated with arr[i] where i is the current iteration.
STOP
Pseudocode
Let us next look at the pseudocode to print an array in C concerning what we discussed in the above Algorithm section.
C program to Print the Elements of an Array
There are a lot of ways we can print the elements of an array in C. Let us take a look at some of the standard ways to print an array in C.
-
C Program to Print an Array Using For Loop
We can use for loop to print the elements of an array in C. Let us take a look at the code and then understand the logic behind it.
Output
Explanation
In the above code, we print an array in C with the help of for a loop. We create an array arr initially and then we iterate over all the elements of the array using the for loop and then print the ith element in the ith iteration.
-
C Program to Print an Array Using while loop
We can also use a while loop to print the elements of an array in C. Let us again take a look at the code and then understand the logic.
Output
Explanation
In the above code, we print an array in C with the help of a while loop. We create an array arr initially and then we use a while loop with the condition that the variable i which is initialized with 1 should be less than the size of the array. Every time the while loop runs we print the ith element and update the variable i by i+1.
-
C Program to Print an Array using a do-while loop
Similar to a while loop we can also use a do while loop to print the elements of an array in C. Let us again take a look at the code and then understand the logic behind it.
Output
Explanation
In the above code, we print an array in C with the help of a do while loop. The code is very similar to the code where we use a while loop. The only difference is that the condition for while is checked at exit and not at the entry.
-
Program to Print Elements in an Array using Functions
We can also create a function to print the elements of an array in C. Let us again take a look at the code and then understand the logic behind it.
- Using for loop with a function to print an array in C
Output
Explanation
In the above code, we declare a function by the name Print_array() which takes as input the array which is to be printed and the size of the array. The function internally uses a for loop to print an array as was discussed in the previous sections.
Note: If the array has no elements, that is if the length of the array is zero, we cannot print anything.
-
Using While Loop with a Function to Print Array in C
Output
Explanation
In the above code, we declared an array Print_array() which takes in the array which is to be printed and the size of the array. The function internally uses a while loop to print the array as was explained in the previous sections.
-
Program to Print Elements in an Array using Recursion
The last standard way to print the elements of an array in C is by using recursion. Let us look at the code and then understand the logic behind it.
Output
Explanation
In the above code, we use recursion to print an array in C. We pass three parameters to the recursive function Print_array() which are, the array whose elements are required to be printed, the position of the elements which need to be printed in the current recursive call and the length or the size of the array. We set a base condition that if the current element that we have to print is greater than the length or the size of the array then we stop the recursion, otherwise, we print the current element and recursively call the function with the parameter "cur" changed to "cur + 1" where cur stands for the current index that needs to be printed.
Conclusion
- Printing an array in C involves outputting the elements of the array to the console in order.
- We can print an array in C using the following methods:
- Using for loop
- Using while loop
- Using do-while loop
- Using recursion
- Using functions