C++ Program to Find the Product of Two Numbers
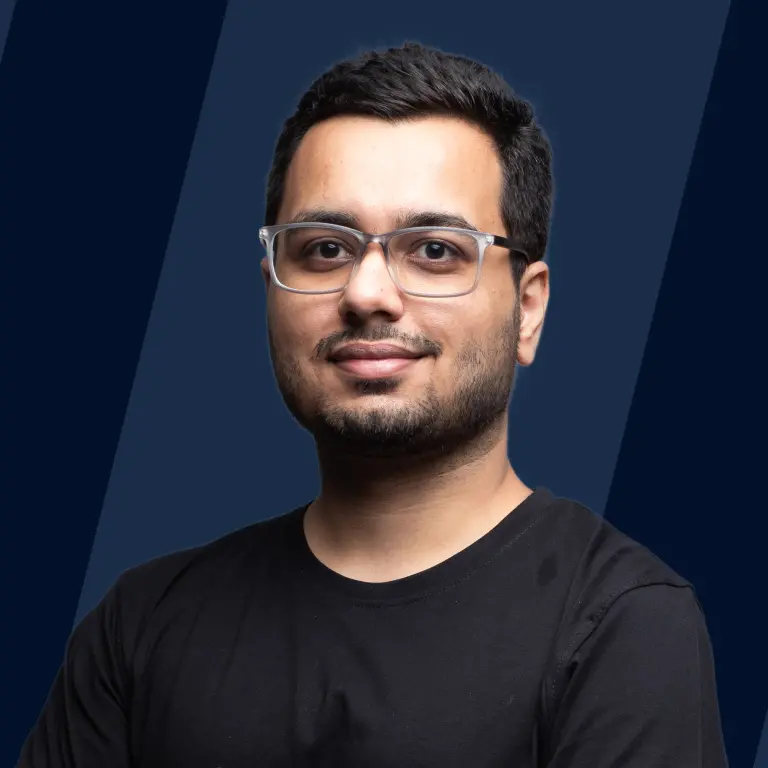
In C++, arithmetic operations like addition, subtraction, multiplication, and division can be executed. Specifically, the * operator calculates the product of two numbers. This article demonstrates writing a C++ program to find two numbers' product, highlighting the direct use of * and the alternative method of repeated addition with a for loop.
Program to Find the Product of Two Numbers Using * Operator
The product of two numbers can be evaluated easily using the * operator. The * operator is used to multiply two numbers. Let us look at the code.
Example
Below is the code to multiply two numbers using the * operator in C ++.
Output
The output of the above code is as follows.
How Does this Program Work?
Let us break down the code and understand what each line in the above program does.
- In the above line of code, we declared three variables, num_1, num_2, and product of double data type. The variables num_1 and num_2 are used to store the numbers to be multiplied, and the variable product will store the product of the two numbers.
- With the help of the above lines of code, we will ask the user to enter the numbers using the cout function and store them in the variables num_1 and num_2 using the cin function.
- This is the important line of code responsible for computing the product of two numbers. The above line uses the * operator to find the product of the two numbers.
- This line of code is used to print the product's value stored in the product variable.
Program to Find the Product of Two Numbers Without Using * Operator
Multiplication is also known as repeated addition. We can find the product without using the * operator. Let us take a simple example of , i.e., two fours are eight. This means that we are adding 2's for 4 times. For example, the product of can be calculated using the repeated addition as . We can use the same logic and find the product of two numbers without using the * operator.
Example
The following code is used to calculate the product of two numbers without using the * operator.
Output
The output of the above code is as follows.
How Does this Program Work?
Let us break down the code and understand what each line in the above program does.
- In the above lines of code, we will declare the variables num_1 and num_2 to store the input numbers and the product variable to store the product of two numbers. Using the function cin and cout, we will ask the user to enter the numbers and store them in the variables num_1 and num_2. We will also initialize the value of the product variable to 0.
- We will use the for loop to perform the repeated addition of the number. We will add num_1 to the product variable num_2 times to get the product of two numbers.
- This line of code is used to print the product value stored in the product variable.
Conclusion
- Multiplication is the repeated addition of a number.
- The product of two numbers can be easily calculated using the * operator.
- The product of two numbers can be calculated without using the * operator by repeated addition of the number.