Program to Find Area of Triangle
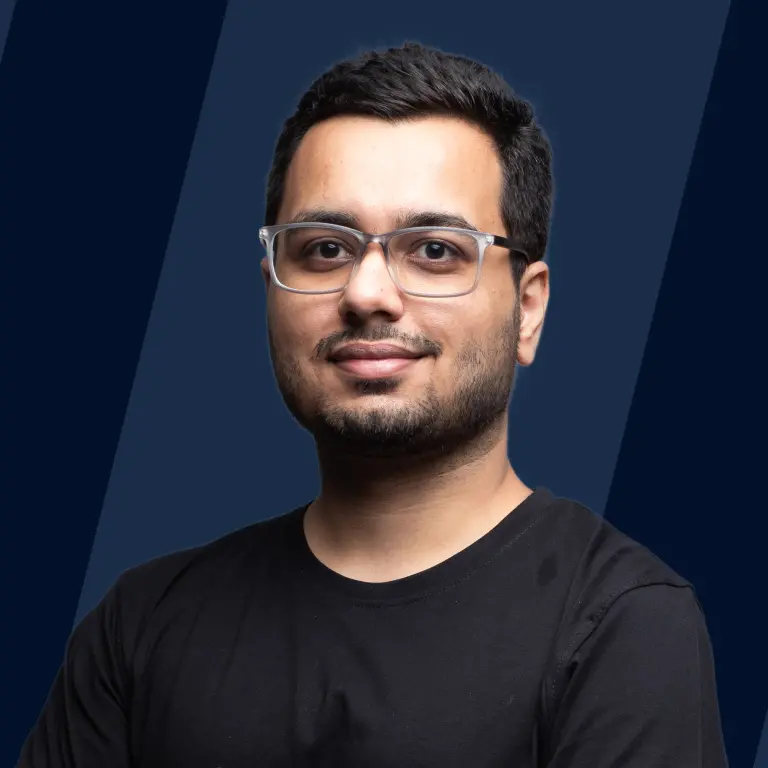
We have several methods to find the area of a triangle, whether it might be using the Heron formula, distance formula, or just a basic formula to find the area of a right-angled triangle. In this article, we will study the different methods by which we can find the triangle area.
Introduction to Area of Triangle
Have you ever wondered how we can find the area of a triangular object, whether physical or visualized on graph paper, by knowing only its dimensions or coordinates? Read the article to learn more about that problem and its solution.
There are several ways to calculate the area of a triangle. Here, we will discuss all possible methods for writing a program to calculate the area of a triangle.
Algorithm for Area of Triangle
Finding Area Of Triangle Using Given Sides
If we have 3 sides of the triangle and it is said that we have to find the area of the triangle by using giving sides.
First of all, we have to check whether these sides are correct to form a triangle or not. To check that, we have to add the condition, i.e., the sum of any of the 2 sides is greater than the third or not. If this condition is valid for all 3 conditions, constructing the triangle is possible. After that, we will use a formula famous for finding the area of a triangle using sides called Herons Formula.
Below is the equation of Heron's Formula:
where,
s: semi-perimeter
a: first side of triangle
b: second side of triangle
c: third side of triangle
How to find Semi-Perimeter(s)
Algorithm
We have created the function areaOfTriangle and checked a condition inside the function. These conditions are explained below in points:
- We have checked whether the sides we gave are valid for forming a triangle.
- If sides are not valid then we return that sides are not correct
- Else, we find the semi-perimeter and then calculate the area by using the Herons Formula.
Finding Area Of Triangle Using Given Coordinates
If we have 3 corner coordinates of a triangle, it is said that we have to find the area of the triangle using the given coordinates.
To approach that problem, first, we must compute each side of the triangle using distance-formula. After calculating all the sides of the triangle, we have to check whether these sides are correct to form a triangle. After that, we will use a formula that is famous for finding the area of a triangle using sides is termed as Herons Formula.
The Distance Formula is used to find the distance between only 2 given points. So, to calculate all sides of a triangle, we have to use the distance formula three times on three different pairs of coordinates.
How to find Semi-Perimeter(s)
Algorithm
We have created a function areaOfTriangle and checked a condition inside the function. These conditions are explained below in points:
- We have checked whether sides which are given to us are valid to form a triangle or not.
- First of all, we find all the sides of the triangle using the distance formula.
- If sides are not valid then we return that sides are not correct
- Else, we find the semi-perimeter and then calculate the area by using the Herons Formula.
Complexity for Area of Triangle
We have studied both algorithms in depth to understand how we can find the area of the triangle when we have different use cases. After understanding the algorithms (by using given sides and by using given coordinates), we can see that we are not using any loop for finding the area. So, it means that the complexity is not in the form of nSquare or nCube.
As we go deeper, we can analyze that we are just using square roots to calculate the triangle area. So we can conclude that the overall complexity depends only on the complexity of the square root method as all other operations are performed in constant time i.e. O(1).
Due to its internal implementation, the square root method is O(log n) complex. Similarly, the time complexity of Finding the Area of a Triangle by the above-mentioned algorithms is O(log n).
Finding Area Using Given Sides
As we understand, the algorithm for finding the area of a triangle uses giving sides. Let's write the code to find the area of a triangle in different languages.
Python Code
JavaScript Code
C++ Code
C Code
Output Of All Codes
Finding Area of Triangle Using Coordinates
As we have understood, the algorithm for finding the area of a triangle uses the coordinates of a triangle. Let’s write the code to find the area of a triangle in different languages.
Python Code
We have to import Python's math library so that we can use its built-in functions, such as pow(for the power function) and sqrt(for the square root function).
JavaScript Code
C++ Code
C Code
Output Of All Codes
Conclusion
- First of all, we understand the basics of Heron's formula and how we can use it to find the area of a triangle.
- Then, we understand the distance formula, which helps us find the distance between the two given points.
- After that, we understand the time complexity of both algorithms.