Python PyAutoGUI Library
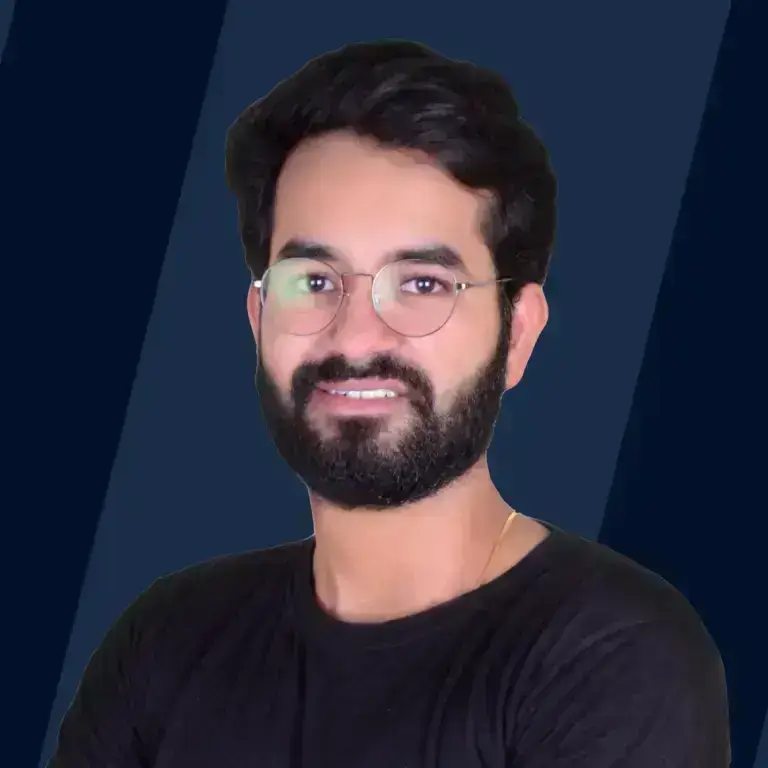
PyAutoGUI in Python allows you to explore the potential of automation in Python. This powerful library allows developers to easily handle the mouse and keyboard, making repetitious operations simple. PyAutoGUI's straightforward syntax makes it easy to automate GUI interactions and create efficient processes. PyAutoGUI can help you increase productivity and streamline your Python projects. With this user-friendly Python library, you can say goodbye to manual, time-consuming chores in favor of the efficiency of automated scripting. With PyAutoGUI, you can enjoy the convenience of automation while taking your coding to the next level.
What is the PyAutoGUILibrary?
In the broad field of Python libraries, PyAutoGUI stands out as a flexible module in Python that meets the automation demands of both developers and fans. As we explore deeper into this powerful library, we'll learn about its features, use cases, and the seamless automation experiences it provides.
PyAutoGUI in Python is a Python module that automates graphical user interface (GUI) interactions. This package allows developers to automate repetitive processes, replicate keyboard and mouse inputs, and seamlessly move around apps. PyAutoGUI provides something for everyone, whether you're an experienced programmer looking to streamline operations or a curious enthusiast new to automation.
Let us now look at some key features of PyAutoGUILibrary.
Cross-Platform Compatibility:
PyAutoGUI in Python is intended to be platform-independent, making it a good alternative for developers working with a variety of operating systems. Whether you're using Windows, macOS, or Linux, PyAutoGUI adjusts to your environment, delivering consistent performance across platforms.
Simple and Intuitive Syntax:
One of the standout features of PyAutoGUI in Python is its straightforward and easy-to-understand syntax. Developers can automate complicated activities with only a few lines of code, making it an excellent choice for both new and experienced programmers. PyAutoGUI's syntax is simple, which speeds up the automation process and allows developers to focus on their responsibilities without being distracted by complex code.
Mouse and Keyboard Control:
PyAutoGUI in Python excels at delivering precise control over mouse and keyboard input. Developers may manipulate the mouse pointer, click on particular coordinates, imitate keyboard inputs, and even take screenshots with ease. PyAutoGUI's degree of control makes it an excellent tool for automating a variety of activities, from data entry to GUI testing.
Let's have a look at some popular PyAutoGUILibrary usage cases.
Automating Repetitive Tasks:
PyAutoGUI in Python proves invaluable when it comes to automating repetitive tasks, such as data entry, file manipulation, or form submissions. By scripting the sequence of mouse and keyboard events, developers may save time and limit the possibility of human mistakes.
GUI Testing and Validation:
PyAutoGUI in Python makes quality assurance and testing more efficient. Developers may write automated test scripts to interact with graphical interfaces, verifying that programs work as intended in a variety of circumstances. This is especially useful in agile development contexts, where quick and frequent testing is required.
Screen Capture and Image Recognition:
Advanced automation scenarios are made possible by PyAutoGUI's ability to take screenshots and recognize images. Developers may write scripts that detect and interact with specific parts on the screen, making it an effective tool for automating operations in applications with dynamic or changing interfaces.
Let us now see some of the reasons why we should use PyAutoGUILibrary.
Cross-Platform Compatibility:
PyAutoGUI in Python is a cross-platform automation library that functions perfectly on Windows, macOS, and Linux. This adaptability enables developers to write automation scripts that are not dependent on a single operating system, increasing flexibility and portability.
Ease of Use:
PyAutoGUI's simplicity is one of its main features. Python developers, particularly those new to automation, may rapidly learn the language's straightforward syntax. PyAutoGUI simplifies the complexities of GUI automation, allowing users to focus on specific tasks rather than becoming bogged down in technical minutiae.
Extensive Documentation and Community Support:
PyAutoGUI in Python has extensive documentation that walks users through its features and functionality. Furthermore, the active community behind PyAutoGUI guarantees that any difficulties or questions are quickly resolved. This strong support system makes it an excellent alternative for individuals who appreciate collaboration and helpfulness.
Wide Range of Features:
PyAutoGUI in Python provides a wide range of functions, including mouse and keyboard control, screen capture, and image recognition. This toolkit's adaptability enables developers to automate a wide range of processes, from data entry to testing and beyond. The ability to operate the mouse and keyboard allows for the automation of interactions with graphical user interfaces.
In conclusion, the PyAutoGUI in Python package demonstrates the Python community's dedication to automating hard activities. Its flexibility, cross-platform interoperability, and straightforward syntax make it suitable for developers of all skill levels. Whether you're automating routine operations or improving your testing procedures, PyAutoGUI allows you to easily unleash the power of automation. As we continue to study the ever-changing ecosystem of Python packages, PyAutoGUI remains a useful asset in the arsenal of any developer seeking efficiency and productivity.
Installing PyAutoGUI
Now that we've covered the benefits of PyAutoGUI in Python, let's walk through the simple process of installing it on your machine. Follow these steps to get started:
1. Prerequisites
PyAutoGUI requires Python installation on your machine. You may get the most recent version of Python from the official website (https://www.python.org/).
2. Installing PyAutoGUI:
Open your terminal or command prompt and enter the following command:
This program will download and install PyAutoGUI and its dependencies. Once the installation is complete, you may begin automating tasks.
3. Verifying Installation:
To ensure that PyAutoGUI was successfully installed, construct a simple Python script with the following lines:
Run the script, and if you see the screen width and height printed, congratulations – you've successfully installed PyAutoGUI!
In conclusion, PyAutoGUI in Python is an appealing option for those wishing to simplify automation activities. Its cross-platform portability, user-friendliness, rich documentation, and community support make it an ideal tool for both new and seasoned developers. By following the simple installation steps indicated above, you can immediately leverage the power of PyAutoGUI and optimize your workflows. Begin automating with confidence, and let PyAutoGUI handle the tedious duties, freeing up your time to focus on what is most important: your creative and complicated projects.
Fundamentals of PyAutoGUI
In the world of automation, PyAutoGUI in Python stands out as a reliable and user-friendly Python package that allows developers to easily automate monotonous activities. Whether you're a seasoned programmer or a beginner discovering the marvels of automation, grasping the fundamentals of PyAutoGUI is important. Let's go on an exploration to discover its core functions, including syntax and descriptions.
Basic Functions
pyautogui.position() - Getting Mouse Coordinates:
-
Description: This function returns the current coordinates of the mouse cursor.
-
Syntax:
Consider a scenario where you want to automate a task by precisely clicking on certain elements. pyautogui.position() comes to the rescue, providing real-time coordinates to guide your virtual assistant.
pyautogui.moveTo(x, y, duration) - Moving the Mouse:
-
Description: Moves the mouse cursor to the specified coordinates over a defined duration.
-
Syntax:
Precision is a key in automation. Use pyautogui.moveTo() to smoothly navigate the mouse to a designated spot, creating a seamless and accurate automation experience.
pyautogui.click(x, y, clicks, interval) - Mouse Clicks:
-
Description: Simulates mouse clicks at the specified coordinates with customizable click count and intervals.
-
Syntax:
You are required to automate a repetitive clicking task? With pyautogui.click(), you can control the frequency and intensity of your clicks, adapting to the unique demands of your automation script.
pyautogui.typewrite('text', interval) - Keyboard Input:
-
Description: Types the provided text with an optional keypress interval.
-
Syntax:
Text input is a common requirement in automation. Leverage pyautogui.typewrite() to emulate keyboard input accurately, ensuring your automation script interacts seamlessly with various applications.
pyautogui.screenshot('filename') - Taking Screenshots:
-
Description: Captures the current screen and saves it as an image file.
-
Syntax:
Visual confirmation is crucial. Use pyautogui.screenshot() to capture screenshots during your automation process, providing a valuable reference for debugging and analysis.
Mouse Operations
PyAutoGUI in Python simplifies GUI automation by offering easy-to-use methods for a variety of mouse actions, allowing you to interface with programs and perform tasks effortlessly. This makes automation accessible even to novices.
-
Moving the Mouse:
The moveTo(x, y) function in PyAutoGUI in Python is employed to move the mouse cursor to a specific screen coordinate defined by the (x, y) parameters. Conversely, moveRel(dx, dy) moves the cursor relative to its current position, making it an excellent choice for dynamic interactions.Syntax Example:
-
Clicking the Mouse:
PyAutoGUI in Python facilitates both the single and the double clicks through the click() and doubleClick() functions, respectively. These actions emulate the left mouse button, providing an efficient way to interact with GUI elements.Syntax Example:
-
Dragging and Dropping:
For operations involving dragging and dropping, PyAutoGUI in Python offers the dragTo(x, y) and dragRel(dx, dy) functions. These routines imitate pressing the left mouse button, dragging it to the desired place, and then releasing the button.Syntax Example:
-
Other Useful Functions:
PyAutoGUI includes some additional functions to cater to diverse automation needs. The rightClick() function simulates a right mouse button click, while middleClick() emulates a click of the middle mouse button.Syntax Example:
Mastering the principles of PyAutoGUI's mouse actions allows for more effective GUI automation. PyAutoGUI's simplicity and adaptability make it a handy tool for automating repetitive processes and testing applications. With PyAutoGUI, you may experiment with these functions, discover new features, and improve your automation skills.
Keyboard Operations
PyAutoGUI in Python facilitates automation by offering a collection of routines that simulate human keyboard and mouse interactions. Today, let's look at the keyboard operations, where PyAutoGUI shines.
-
Simulating Key Presses:
PyAutoGUI in Python allows you to easily imitate key presses. The pyautogui.press() function takes care of this task, accepting a key as an argument and emulating a single key press. For example:In this example, the letter a is virtually pushed to simulate human contact with the keyboard.
-
Typing Strings:
PyAutoGUI allows you to automate full strings of keystrokes in addition to individual ones. The pyautogui.typewrite() method is your go-to tool for this.This snippet efficiently types out the specified string, character by character, just as if a user were typing manually.
-
Combining Key Presses:
For more sophisticated circumstances, PyAutoGUI in Python allows you to mix key presses. The pyautogui.hotkey() method is used for this reason. Assume you wish to replicate the shortcut Ctrl + C (copy). Here's how you can accomplish this:This succinctly triggers the Ctrl and C keys simultaneously, replicating the copy command.
-
Controlling Typing Speed:
PyAutoGUI in Python is realistic, enabling you to regulate your typing pace.The pyautogui.typewrite() function accepts an additional argument for the duration of the pause between key presses:
By setting the interval option, you may control the duration between each character written, resulting in a more natural pace.
In terms of automation, PyAutoGUI's keyboard operations offer a simple and powerful way to interact with programs programmatically. Whether it's a single key press, typing out strings, or performing complicated key combinations, PyAutoGUI gives developers the tools they need to automate operations effectively.
Understanding the principles of PyAutoGUI's keyboard operations allows developers to unleash the potential for increased productivity and optimized workflows, eliminating the need for time-consuming manual interactions. So, enter into the realm of PyAutoGUI in Python and let your code manage the keyboard with ease and precision!
Examples
PyAutoGUI in Python stands out in the automation world as a robust and user-friendly library for mouse and keyboard control. Among its many advantages is the ability to create message boxes, which is an effective tool for interacting with users during script execution. In this post, we'll look at real examples of how to use PyAutoGUI to create message boxes and add dynamic elements to your automation scripts.
Message Boxes Using PyAutoGUI
Message boxes let users get information, warnings, or prompts while a script is being executed. They are critical for improving the user experience and offering useful information regarding the script's progress. PyAutoGUI makes creating these message boxes easier for both new and experienced developers.
Let's begin with a basic example. Suppose you want to notify users that a specific task has been completed successfully. Using PyAutoGUI, you can achieve this with just a few lines of code:
In this example, the alert function is used to generate a basic information box. The first input includes the message that you wish to communicate, and the second parameter specifies the title of the message box.
Sometimes, you may require the user to decide on the script execution. PyAutoGUI in Python provides the confirm function for this purpose:
In this example, the confirm function creates a message box with an OK and Cancel button. The user's choices are then captured in the result variable, allowing the developers to tailor the script's behavior accordingly.
PyAutoGUI in Python also supports message boxes for input gathering. Consider the following example:
Here, the prompt function prompts the user to input information, and the entered value is stored in the user_input variable. This input can then be utilized within your script as needed.
In this PyAutoGUI in Python message box tutorial, we covered basic information boxes, user confirmation prompts, and input collection. Including these message boxes in your scripts may significantly increase user engagement and give useful feedback. As you continue to explore the realm of automation, knowing PyAutoGUI's capabilities will improve the productivity and usability of your scripts.
Conclusion
- PyAutoGUI in Python stands noteworthy due to its simplicity. You may automate monotonous operations with only a few lines of code, making it an excellent solution for both new and experienced developers. Its user-friendly design means that you may get started with automation without having to learn too much.
- One of PyAutoGUI's most significant features is its cross-platform portability. PyAutoGUI adapts easily to Windows, macOS, and Linux, ensuring a consistent automation experience. This adaptability broadens its usefulness, making it a useful tool for a variety of development contexts.
- PyAutoGUI's pixel-based automation method provides exact control over GUI components. This pixel-perfect accuracy is extremely useful for dealing with specific UI components, ensuring that your automation scripts run consistently and precisely across several apps and interfaces.
- PyAutoGUI's picture recognition skills set it distinct from other automation tools. PyAutoGUI offers flexible automation by detecting and interacting with on-screen items using pictures rather than particular coordinates. This capability is very useful in situations where GUI components can alter dynamically.
- PyAutoGUI in Python fits easily with the Python Package Index (PyPI) environment. This includes access to a plethora of extra libraries and tools that enhance PyAutoGUI's capabilities. Whether you want complex image processing or improved keyboard and mouse control, PyAutoGUI's interoperability with PyPI allows you to easily expand its capabilities.
- PyAutoGUI has an active and friendly community. With active development and regular updates, the tool continues to progress, correcting errors, offering new features, and remaining aligned with the ever-changing environment of automation. The community-driven nature ensures that users have access to resources, forums, and documentation, fostering a collaborative environment.
- PyAutoGUI enables developers to streamline operations and increase productivity. Whether automating mundane tasks or orchestrating complex processes, PyAutoGUI's ease of use, cross-platform compatibility, pixel-perfect precision, image recognition, integration capabilities, and community support all help to achieve significant efficiency gains in automation.