Pyramid Patterns in C
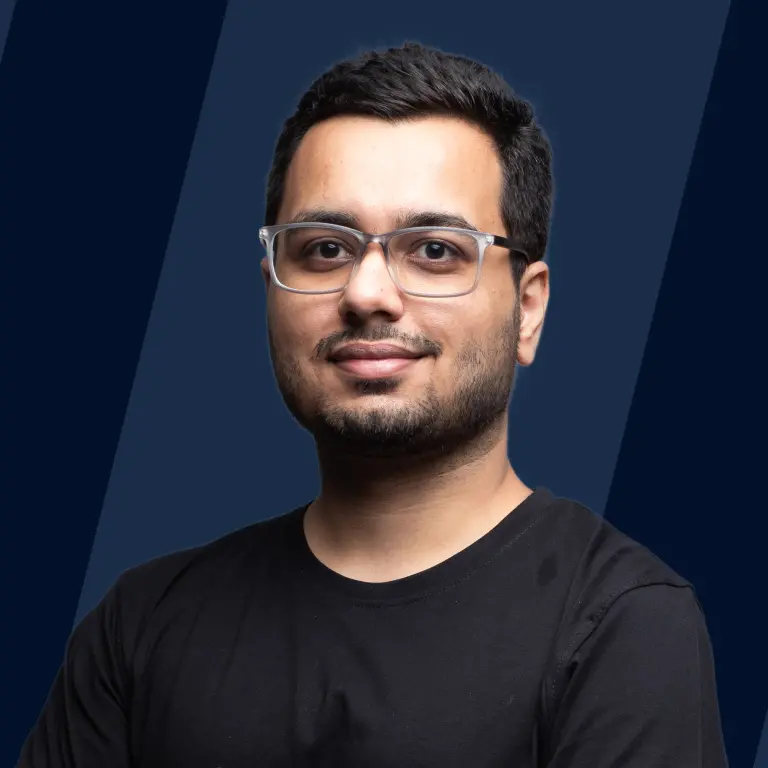
Overview
In this article, we are going to discuss the pyramid pattern in C. The Pyramid pattern in C consists of the pyramid pattern of numbers, pyramid pattern of stars, and pyramid pattern of alphabets. Pyramid designs are all made up of polygons. Each pattern program contains two or more loops.
Pattern program can also contain if-else statements.
The complexity of the pattern or logic determines the number of loops. The first for loop represents the row, whereas the second loop represents the column. Solving pattern printing problems is the simplest approach to understanding how a nested loop works.
In C, there are three types of pyramid patterns:
- Star Pyramid Patterns
- Number Pyramid Patterns
- Alphabets Pyramid Patterns
Star Pyramid Patterns
The Star pyramid contains half pyramids, inverted half pyramids, inverted right half pyramids, right half pyramids, full pyramids in 180 degrees, full pyramids in the opposite direction, full pyramid, zig-zag pattern, rectangle pattern, rhombus pattern, butterfly pattern, diamond-shaped pyramids.
Program to Print the Half Pyramid
Approach: To print a half pyramid of size N:
- First, we traversed over a for loop from i to numbers of rows (i <= rows) to create N rows.
- At ith row, using for loop we printed whitespaces in first rows-i columns and stars in remaining i columns.
Output:
Program to Print an Inverted Half Pyramid Pattern
Approach: To print an inverted half pyramid of size N:
- First, we traversed over a for loop from number of rows to 1 (i >= 1) to create N rows.
- At ith row, using for loop we printed whitespaces in the first rows-i columns and starts in the remaining i columns.
Output:
Program to Print an Inverted Right-half Pyramid
Approach: To print an inverted right-half pyramid of size N:
- First, we traversed over a for loop from number of rows to 1 (i >= 1) to create N rows.
- At ith row, using for loop we printed starts in i columns.
Output:
Program to Print the Right Half Pyramid
Approach: To print the right half pyramid of size N:
- First, we traversed over a for loop from 1 to number of rows (i <= rows) to create N rows.
- At ith row, using for loop we printed starts in i columns.
Output:
Program to Print the Full Pyramid of Star in 180 Degree
Approach: To print the full pyramid of the star in 180 degrees of size N:
- We printed first half pyramid of size N and then inverted half pyramid of size N-1 (here; N is row number)
Output:
Program to Print the Full Pyramid of Star In the Opposite Direction
Approach: To print the the full pyramid of star in the opposite direction of size N:
- We printed first half pyramid of size N and then inverted half pyramid of size N-1 (here; N is row number).
Output:
Program to Print the Full Pyramid of Star
Approach: To print the full pyramid of star of size N:
- First, we traversed over a for loop from 1 to number of rows to create N rows.
- At ith row, using for loop we printed whitespaces in first rows-i columns and stars in remaining 2*i-1 columns.
Output:
Program to Print the Inverted Full Pyramid of Star
Approach: To print the inverted full pyramid of star of size N:
- First, we traversed over a for loop from number of rows to 1 to create N rows.
- At ith row, using for loop we printed whitespaces in first rows-i columns and starts in remaining 2*i-1 columns.
Output:
Program to Print Zig-Zag Pattern Using Star
Approach: To print the zig-zag pattern using star of size N:
- First, we traversed over a for loop from 1 to 3 to create 3 rows.
- At ith row, using for loop we printed stars by following this condition (((i + j) % 4 == 0) || (i == 2) && j % 4 == 0) and whitespaces in remaining columns.
Output:
Explanation: Let's understand it by the below figure.
If we take the indices of every star
And we add add all corresponding indices. then we get a number which is divisble by 4 and we will print stars here. and when our row number is equal to 2 and column number is divisble by 4 then we will print star. so, the condition will be followed here (((i + j) % 4 == 0) || (i == 2) && j % 4 == 0) and we will print spaces in remaining space.
Program to print solid rectangle pattern using star
Approach: To print solid rectangle pattern using star of size N:
- First, we traversed over a for loop from number of rows and number of columns to create N rows and N columns.
- At jth column, using for loop we printed stars according to number of columns.
Output:
Program to Print Hollow Rectangle Pattern Using Star
Approach: To print hollow rectangle pattern using star of size N:
- First, we traversed over a for loop from number of rows and number of columns to create N rows and N columns.
- At jth column, using for loop we printed stars by following this condition (i == 1 || i == rows || j == 1 || j == columns) and whitspaces in remaining column.
Output:
Program to Print Solid Rhombus Pattern Using Star
Approach: To print solid rhombus pattern using star of size N:
- First, we traversed over a for loop from 1 to number of rows (i >= 1) to create N rows.
- At ith row, using for loop we printed whitespaces in first rows-i columns and stars in remaining 1 to numbers of row.
Output:
Program to print hollow rhombus pattern using star
Approach: To print hollow rhombus pattern using star of size N:
- First, we traversed over a for loop from number of rows to 1 to create N rows.
- At ith row, using for loop we printed stars by following this condition i == 1 || i == rows and j == 1 || j == rows and whitespaces in remaining columns.
Output:
Program to Print Solid Butterfly Pattern Using Star
Approach: To print solid butterfly pattern using star of size N:
- First, we traversed over a for loop from number of rows to 1 to create 2*N rows. For Upper part
- For row, loop will be traversed from 1 to number of rows.
- For stars, we are printing stars equal to the number of row.
- For space, we are printing space in terms of 2 * rows - 2 * i For lower part
- For row, loop will be traversed from number of rows to 1.
- The remaining part will be same according to upper part.
Output:
Program to Print Hollow Butterfly Pattern Using Star
Output:
Program to Print Solid Diamond Pattern Using Star
Output:
Program to Print Hollow Diamond Pattern Using Star
Output:
Number Pyramid Patterns
The Number pyramid contains half pyramids, inverted half pyramids, inverted right half pyramids, right half pyramids, full pyramids in 180 degrees, full pyramids in the opposite direction, full pyramids, floyd triangles, diamond-shaped pyramids, pascal triangles, 0-1 pyramid pattern, and palindrome pyramid pattern.
Program to Print the Half Pyramid of Number
Approach: To print a half pyramid of number of size N:
- First, we traversed over a for loop from i to numbers of rows to create N rows.
- At ith row, using for loop we printed whitespaces in first rows-i columns.
- At last we traversed over a loop we from 1 to ith row to printing a number.
Output:
Program to Print an Inverted Half Pyramid Pattern
Approach: To print an inverted half pyramid of number of size N:
- First, we traversed over a for loop from i to numbers of rows to create N rows.
- At ith row, using for loop we printed whitespaces in first rows-i columns.
- At last we traversed over a loop we from i to number row to printing a number.
Output:
Program to Print the Right Half Pyramid of Number
Approach: To print the right half pyramid of number of size N:
- First, we traversed over a for loop from i to numbers of rows to create N rows.
- At last we traversed over a loop we from 1 to ith row to printing a number.
**Output: **
Program to Print an Inverted Right Half Pyramid of Number
Approach: To print an inverted right half pyramid of number of size N:
- First, we traversed over a for loop from numbers of rows to 1 create N rows.
- At last we traversed over a loop we from 1 to ith row to printing a number.
Output:
Program to Print the Floyd Triangle
Approach: To print the Floyd triangle of size N:
- We are printing a number which is increasing by 1. Here rows is equal to size of pyramid and column number is from 1 to row number.
- We will make a variable called count which will be intialized from 1.
- We will be updating our count variable after printing current value of count variable.
Output:
Program To Print The Diamond-Shaped Pyramid Of Number
Output:
Program To Print The Full Pyramid Of Number In 180 Degree
Approach: To print the the full pyramid of number in the 180 degree of size N:
- We printed first half pyramid of size N and then inverted half pyramid of size N-1 (here; N is row number).
Output:
Program To Print The Full Pyramid Of Number In The Opposite Direction
Approach: To print the the full pyramid of number in the opposite direction of size N:
- We printed first half pyramid of size N and then inverted half pyramid of size N-1 (here; N is row number).
Output:
Program to Print the Full Pyramid of Number
Approach: To print the full pyramid of number of size N:
- First, we traversed over a for loop from 1 to number of rows to create N rows.
- Secondly, we traversed over a for loop from 1 to number of rows - 1 to printing spaces.
- At last, we traversed over a for loop from 1 to ith row to printing a number.
Output:
Program To Print The Inverted Full Pyramid Of Number
Approach: To print the inverted full pyramid of number of size N:
- First, we traversed over a for loop from number of rows to 1 to create N rows.
- Secondly, we traversed over a for loop from 1 to 2 * i - 1 to printing numbers in columns.
Output:
Program to Print the Pascal Triangle
Approach: To print the pascal triangle of size N:
- First, we traversed over a for loop from 0 to number of rows to create N rows.
- We will make a variable called coef which will be intialized with 1.
- If row and column is equal to 0 then we will print 1 Otherwise we will assign coef * (i - j + 1) / j to the coef variable and print the coef's value.
Output:
Program to Print 0-1 Pyramid Pattern
Approach: To print 0-1 pyramid pattern of size N:
- First, we traversed over a for loop from number of rows to 1 to create N rows.
- Secondly, we traversed over a for loop from 1 to number of rows to create N columns.
- Finally, we'll add the index numbers of all elements' rows and columns (row + column) and print 1 if the result is an even number; else, we'll print 0.
Consider the below diagram:
Output:
Program to Print the Palindromic Pattern
Output:
Alphabets Pyramid Patterns
The Alphabet pyramid contains half pyramids, inverted half pyramids, inverted right half pyramids, right half pyramids, full pyramids, and diamond-shaped pyramids.
Program to Print the Half Pyramid of Alphabets
Approach: To print the half pyramid of alphabets of size N:
- First, we traversed over a for loop from 1 to number of rows to create N rows.
- Secondly, we traversed over a for loop from ith row to number of rows to printing spaces.
- At last, we traversed over a for loop from 1 to ith row to printing a character.
Output:
Program to Print the Inverted Half Pyramid of Alphabets
Approach: To print the inverted half pyramid of alphabets of size N:
- First, we traversed over a for loop from 1 to number of rows to create N rows.
- Secondly, we traversed over a for loop from ith row to number of rows to printing spaces.
- At last, we traversed over a for loop from ith row to number of rows printing a character.
Output:
Program to Print the Right Half Pyramid of Alphabets
Output:
Program to Print the Inverted Right Half Pyramid of Alphabets
Output:
Program to Print the Full Pyramid of Alphabets
Output:
Program to Print the Inverted Full Pyramid of Alphabets
Output:
Program to Print the Diamond-Shaped Pyramid of Alphabets
Output:
Conclusion
- Pattern programs are simply patterns made up of numbers, alphabets, or symbols in a specific order.
- Pyramid pattern in C have the advantage of learning how to use loops and if-else statements.
- These types of pattern programs are simple to solve utilizing the for loop condition.
- Beginners can use pattern programs to visualize how each iteration of a loop works.
- By practicing pyramid pattern we can build our logical thinking of problem solving.