Pyramid Program in Java
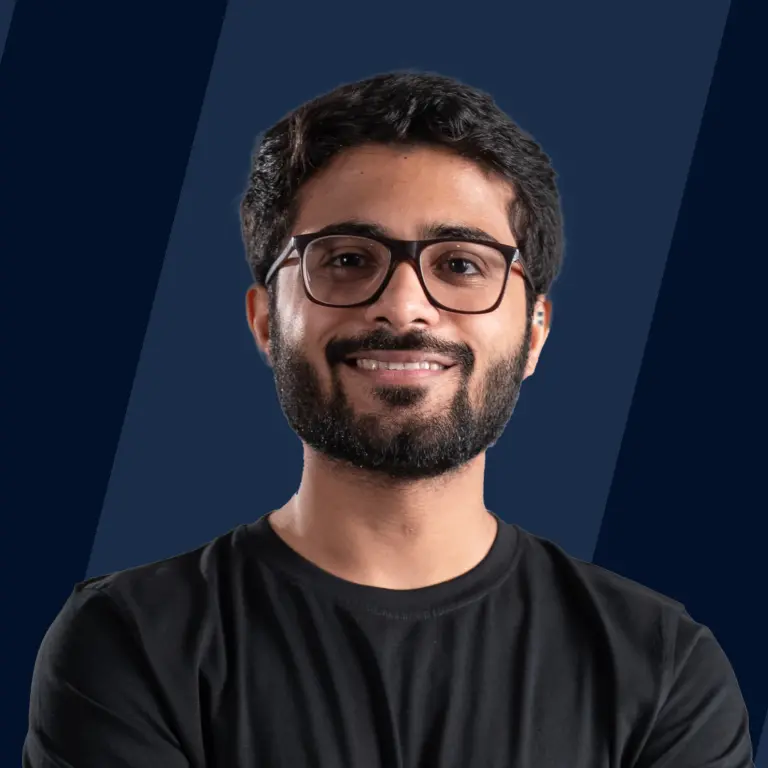
The Pyramid program is a pattern printing program. Pattern printing programs help the developer build the basic logic required in programming, and they also help the developer get acquainted with the loops in Java. There are different types of pattern printing programs, such as Star pattern printing programs, character pattern printing programs, and number pattern printing programs. This article covers many examples of such types of pattern printing programs.
Introduction
Pattern Printing programs can be very helpful in developing the logic required for programming. Various loops, like for loop, while loop, etc., can be used extensively in these programs, which results in better-looping concepts and enhanced logic. Printing programs also show the logical ability of a developer to solve particular programs; hence, these programs are widely asked in the programming interview questions. We will use the concept of sp and st; sp denotes number of spaces while st denotes the number of stars.
What is a Pyramid Program in Java?
A pattern program with a pyramid shape is called the pyramid program in Java.
We will discuss many such pattern programs. In most of the patterns given below, the first for loop is used to traverse the rows, while the second for loop is used to traverse the columns of those rows. There are four sections of each program:
- Explanation
- Code of the program.
- Given Input.
- Expected Output.
Further, all these pattern programs are categorised into three types:
- Star Patterns in Java
- Number Patterns in Java
- Alphabet/Character Patterns in Java
Note: We have taken \t as the standard space to avoid space differences in patterns in most of the programs in this article.
Star Patterns in Java
This section will show some popular star pattern programs in Java.
Pyramid Program
A star pattern program that looks like a pyramid is called a Pyramid program in Java. In this program, we set the initial value of st as 1 and sp as n - 1. How did we come to these values? We came to these values by observing the pyramid pattern.
Note: In the same way, we can easily get the initial values of st and sp for all the pattern programs if we observe the pattern carefully.
In the Pyramid program in Java, we first need to print some spaces followed by the odd number of stars. We can notice that in every row, the stars increase by 2 while the spaces decrease by 1.
In this pattern program, three for loops are used. The first for loop is for the number of rows, the second for loop is for printing stars, and the third for loop is for printing spaces.
Input:
Output:
Right Triangle Star Pattern
In the Right Triangle Star Pattern program, we set the initial value of st as 1 by observing the pattern. We can notice that, in this Pattern we do not need any spaces before the stars and in every row the stars increase by 1.
In this pattern program, two for loops are used. The first for loop is for the number of rows, and the second for loop is for printing stars.
Input:
Output:
Left Triangle Star Pattern
In the Left Triangle Star Pattern program, we observe the pattern and set the initial values of st as 1 and sp as n-1. We notice that in every row, the spaces decrease by 1 while the stars increase by 1.
In this pattern program, two for loops are used. The first for loop is for the number of rows the, second for loop is for printing spaces, and the third for loop is for printing stars.
Input:
Output:
Downward Triangle Star Pattern
In the Downward Triangle Star Pattern program, we set the initial value of st as 1 by observing the pattern, and we don't need sp in this case. We can notice that in every row, the stars decrease by 1.
In this pattern program, two for loops are used. The first for loop is for the number of rows, and the second for loop is for printing the stars.
Input:
Output:
Inverted/Reversed Pyramid Star Pattern
In the Reversed Pyramid Star Pattern program, we observe the pattern and set the initial values of st as n and sp as 0. We notice that in every row, the spaces increase by 1 while the stars decrease by 2.
In this pattern program, three for loops are used. The first for loop is for the number of rows, and the second for loop is for printing the spaces, while the third for loop is for printing the stars.
If you look closely, you can see that this is nothing but the inverted Pyramid program in Java.
Input:
Output:
Diamond Shape Pattern Program in Java
In the Diamond Shape Pattern Program, we set the initial value of st as 1 and sp as n / 2 by observing the pattern.
This program is different from the other programs we have seen so far because we have to add different conditions in different halves of the pattern. In the first half of this pattern, stars increase by 2, and spaces decrease by 1, while in the second half, stars decrease by 2, and spaces increase by 1.
In this pattern program, three for loops are used. The first for loop prints the number of rows, the second for loop prints the spaces, and the third for loop prints the stars.
If you look closely, you can see that this is nothing but the combination of a Pyramid program in Java and an inverted/reversed Pyramid program in Java.
Intput:
Output:
Right Down Mirror Star Pattern
In the Right-Down Mirror Star Pattern program, we observe the pattern and set the initial values of st as n and sp as 0. We notice that in every row, the spaces increase by 1 while the stars decrease by 2.
In this pattern program, three for loops are used. The first for loop is for the number of rows, and the second for loop is for printing the spaces, while the third for loop is for printing the stars.
Input:
Output:
Right Pascal’s Triangle
In Right Pascal's Triangle Star Pattern program, we observe the pattern and set the initial values of st as 1 and sp as 0. We notice that in the first half of the pattern, the stars increase by 1 while in the second half, they decrease by 1.
In this pattern program, two for loops are used. The first for loop is for the number of rows(here 2*n) and the second for loop is for printing the stars.
Input:
Output:
Left Pascal’s Triangle
In Left Pascal's Triangle Star Pattern program, we set the initial values of st as 1 and sp as n—1 by observing the pattern. We can notice that in the first half of the pattern, the stars increase by 1 and spaces decrease by 1, while in the second half, the stars decrease by 1 and spaces increase by 1.
In this pattern program, three for loops are used. The first for loop is for the number of rows(here 2*n), the second for loop is for printing the spaces, and the third for loop is for printing the stars.
Input:
Output:
Sandglass Star Pattern
In the Sandglass Star Pattern program, we set the initial values of st as n and sp as 0 by observing the pattern. We can notice that in the first half of the pattern, the stars decrease by 2 and spaces increase by 1, while in the second half, the stars increase by 2 and spaces decrease by 1.
In this pattern program, three for loops are used. The first for loop prints the number of rows, the second for loop prints the spaces, and the third for loop prints the stars.
Input:
Output:
Alphabet A Pattern
In the Alphabet A Star Pattern program, we do not use nst and nsp because, in this case, it's easier without using these two.
In this pattern program, two for loops are used. The first loop counts the number of rows, and the second loop prints the stars or spaces based on the given condition.
Input:
Output:
Triangle Star Pattern
In the Triangle Star Pattern program, we set the initial value of st as 1, sp1 as n - 2 and sp2 as 1 by observing the pattern. There can be three cases in this situation. If i == 1, else if i == n and an else case. The number of for loops vary depending on the cases.
This pattern is somewhat similar to that of the Pyramid program in java, the only difference is that the Pyramid program in java is filled with stars but this pattern is not filled with stars.
Input:
Output:
Down Triangle Star Pattern
In the Down Triangle Star Pattern program, we observe the pattern and set the initial values of st as 1, sp1 as 1andsp2as n. We notice that there can be three cases in this situation: ifi == 1, else if i == n`, and an else case. The number of for loops varies depending on the cases.
Input:
Output:
Diamond Star Pattern
In the Diamond Star Pattern program, we set the initial values of st as 1 and sp as n / 2 by observing the pattern. We can notice that in the first half of the pattern, the stars increase by 2 and the spaces decrease by 1; in the second half, the stars decrease by 2 and the spaces increase by 1.
In this pattern program, three for loops are used. The first for loop is for the number of rows, the second for loop is for printing the spaces, and the third for loop is for printing the stars(based on the condition).
Input:
Output:
Numeric Pattern in Java
Now, we will see some of the most famous numeric patterns in Java using this awesome technique of sp and st.
Simple Number Program
This is a simple number program in which we will print the number in each row three times. For example, if the row number is 3, then 3 will be printed three times.
In this pattern, two for loops are used. The first for loop is for the number of rows, and the second for loop is for printing the numbers.
Input:
Output:
Number Pattern Program in Java: 1
In this numeric pattern, numbers start from 1 in every row and are printed row times with an increment of 1 each time. For example, for the third row, the numbers are 1 2 3. We can easily do this by printing the counter variable j in the second for loop as it goes from 1 to the row times, that is' i' times.
In this pattern, two for loops are used. The first for loop is for the number of rows, and the second for loop is for printing the numbers.
Input:
Output:
Number Pattern Program in Java: 2
In this numeric pattern, the first number is 1, and each time, this number is incremented by 1. Here, the numbers are also printed row times. For example, for the third row, the numbers are 4 5 6. This can be done easily using a variable called num, which is initialized with 1 and incremented by 1 in the second for loop.
In this pattern, two for loops are used. The first loop calculates the number of rows, and the second loop prints the numbers.
Input:
Output:
Fibonacci Series Triangle Program in Java
In this numeric pattern, we must print the Fibonacci series in the triangular form. In the Fibonacci series, the first number is 0, the second is 1 and the third number is the sum of the last/previous two numbers.
In this pattern, two for loops are used. The first loop calculates the number of rows, and the second loop prints the numbers.
Input:
Output:
Pascal’s Triangle Program in Java
In this numeric pattern, we will use the factorial concept because Pascal's triangle problem can be easily solved using the factorial concept.
This pattern also looks like the Pyramid program in Java.
Input:
Output:
Diamond Pattern Program in Java(1-9)
For the Diamond Pattern Program we will go with the concept of sp and st as it will make it easier for us. Observing the given pattern, we set the initial values of st as 1, sp as n/2 and num as 0.
We have noticed that in the first half of the pattern, stars(here the number of nums) increase by 2, and spaces decrease by 1. In the second half, stars decrease by 2, and spaces increase by 1. The variable num increases by 1 throughout the pattern; hence, num++ is placed outside the if-else conditional block.
In this case, we have used three for loops: first for the number of rows, second for printing spaces, and third for printing stars.
Input:
Output:
Diamond Numeric Pattern Program in Java(1-5-1)
This pattern is the same as the previous pattern except for the variable num. Here, num is initialised with 0 and is placed inside the if-else conditional block as it increases by 1 in the first half and decreases by 1 in the second half of the given pattern.
Input:
Output:
Descending Order Pattern
As the name suggests, in the descending order pattern, we have to print the numbers in descending order and row times. For example, the numbers in the third row will be 5 4 3.
In this pattern, two for loops are used. The first is for loop for the rows, and the second is for loop for printing numbers.
Input:
Output:
Sandglass Numeric Pattern in Java
As the name suggests, in the sandglass numeric pattern, we have to print the numbers in the shape of a sandglass. Observing the pattern, we set the initial values of st as n, sp as 0, and num as 1.
We can notice that in the first half of the pattern, spaces increase by 1, stars decrease by 2, and num increase by 1, while in the second half of the pattern, spaces decrease by 1, stars increase by 2, and num decrease by 1.
We have used three for loops to print this pattern. The first for loop is for the number of spaces, the second for loop is for printing the spaces and the third for loop is for printing the stars.
Input:
Output:
Binary Number Pattern
In the binary number pattern, as the name suggests, we have to print the numbers in binary format, which is 0 and 1 only. On observing the pattern, we can see that there are four different conditions-
- Whenever the row number is even and the column number is also even, 1 is printed.
- Whenever the row number is even but the column number is odd, 0 is printed.
- Whenever the row number is odd, but the column number is even, 0 is printed.
- Whenever the row number is odd, as well as the column number is also odd, 1 is printed.
In simple words, whenever the row number and column number are even, or both are odd, 1 is printed; otherwise, 0 is printed. In this pattern, two for loops are used based on condition.
Input:
Output:
Zeros/ Ones Pattern Programs
In this pattern, we set the initial st values as 1 and increment by 1 for every row. If the column is even, 1 is printed; otherwise, 0 is printed. Here,
Input:
Output:
Alphabet/ Character Patterns in Java
All the alphabets have some ASCII value associated with them, which helps us in printing these alphabets using their respective ASCII values. Hence, we will use these ASCII values to print alphabets in our pattern. We need to convert the ASCII values into characters using the (char) when printing. The patterns mentioned below are already discussed in the Star Pattern section of this article. We need to add a new variable alphabet with the ASCII value of A and print that alphabet instead of the star. That's all.
Right Alphabetic Triangle
Input:
Output:
A Shape Character Pattern Program
Input:
Output:
K Shape Character Pattern Program
Input:
Output:
Triangle Character Pattern Program in Java
Input:
Output:
Diamond Pattern in Java
Input:
Output:
Conclusion
- Various pattern programs in Java are discussed in this article.
- Star pattern programs in Java are discussed.
- Numeric pattern programs in Java are also discussed.
- Character pattern programs in Java are also discussed.
- We observed that the logic almost remains the same in all of these categories.