Program to Find Average of List in Python
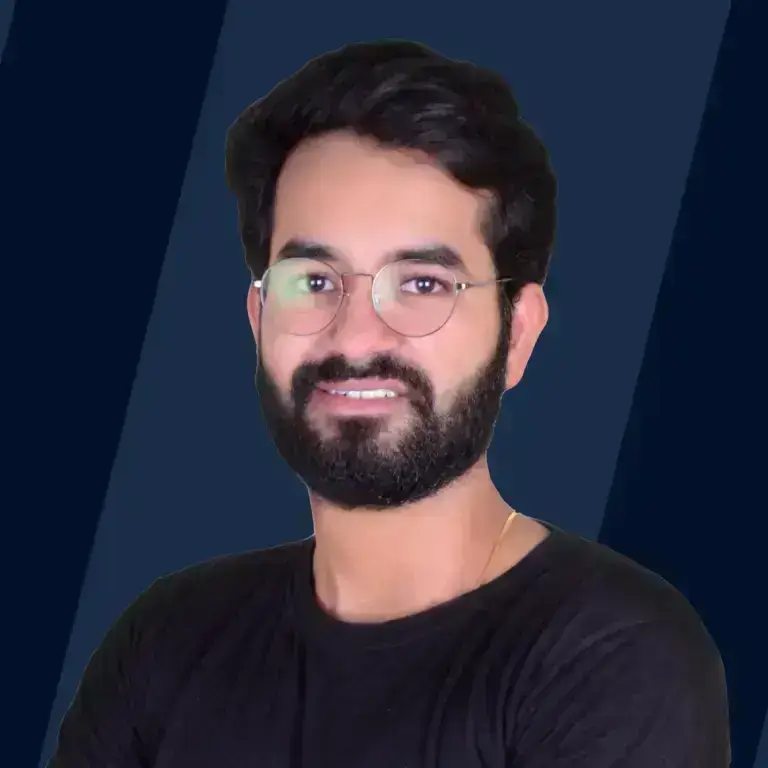
Overview
Python average of list can be calculated in multiple ways. Python provides many different ways to find the average of a list. However, out of these, there are four most commonly used methods. Using sum(), or reduce() with lambda, or mean() function. All these functions are in-built into python. We can also use a simple for loop to find the average of a list in python.
Introduction
The average or Arithmetic Mean of a list in python is the sum of all the elements in the list divided by the length of the list. Finding average is a fundamental concept, and everyone should know it.Even though python provides many in-built functions to find average, we can create our own from scratch using loops.
Before diving deep into finding the average using in-built functions of python. There are a few prerequisites that you know.
Explanation
Prerequisites:
- Basic python
- sum() method
- len() function
- reduce() method
- Lambda expression
- mean() method
Now, let's discuss these methods in detail.
Average of List in Python Using sum()
We can find the average of a list using sum() with len(). We'll use sum() to find the length of the list, and len() to find the length(number of elements) of the list.
Code:
Output:
Explanation:
In the example above, we are using the sum() and len() methods inside the Find_Average() function which we have created. The Find_Average() function takes a list as a parameter.
To find the average we divide the sum returned by the sum() method, by the length of the list that we got using the len() method.
Average of List in Python Using reduce() and Lambda
The reduce() function is used to apply a specific function passed into its argument to apply to all the list elements. We can use this to reduce the loop and to compute the sum of the list elements we'll use the lambda.
Output:
Explanation:
Here, we have used reduce() and lambda to find the average of a list. First, we need to import the reduce() function from functools, and then we can use it in our code.
Average of List in Python Using mean()
This is the most straightforward way. Python has an in-built module called statistics in which it has a method called mean. The mean() method can be used to directly calculate the average of a list without using any other function.
Output:
Explanation:
Here, we have made use of the in-built mean() function. This function does all the work for us, we just have to pass the list. This method will calculate the sum of all the elements of the list and divide it by the length of the list.
Average of List in Python by Iterating List
If we don't want to make use of any in-built python function, we can use loops to iterate over a list and find the Python average of list.
Output:
Explanation:
In this example, we are using for loop to iterate over the list and find the average of the list elements. We can also use a while loop to do the same thing. By iterating over the list elements, we first find the sum of all the list elements and store it in the variable sum. Then to get the average we divide the sum by the length of the list. To get the length of the list we have used a variable to keep the count of the elements of the list, by incrementing the variable by one everytime the for loop iterate.
Conclusion
Average is a basic concept that everyone should know.
- In this article, we discussed different ways to find the Python average of list.
- Using loops
- Using mean() method
- Using reduce() and lambda
- Using sum() and len() methods
- We can use any method depending on the situation, but it's good to know all the methods.