Python callable()
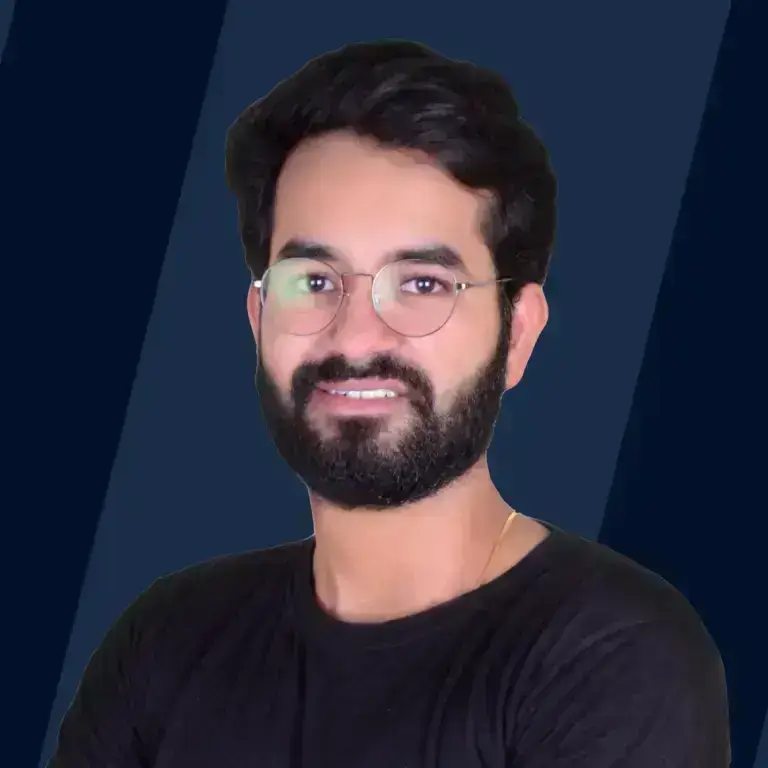
Overview
The Python callable() function takes an object as a parameter and returns True if the object is callable and returns False if the object is not callable.
Syntax of Python callable() Function
The syntax of Python callable() function is :
Syntax:
Parameters of Python callable() Function
The callable() function takes an object as a parameter, which can be a class, module, or another object.
The object passed as a parameter can be a callable or non-callable object.
Return Value of Python callable() Function
The return value of the Python callable() function is :
- True: If the object is callable
- False: If the object is not callable
Even if the callable() function returns True, the object call might fail. If the callable() function returns false then the object calling certainly fails.
:::
Python callable() Function Examples
Some Examples of Python callable() functions are :
Example 1: When an object is callable
Output:
In the above program, the __call__ method enables Python programmers to write classes where the instances behave like functions and can be called like a function.
The instance of the Demo class is callable, so the callable() function returns True when Class Demo is passed to it.
Code:
Output:
Example 2: When Object is not Callable but appears to be Callable
Output:
In the above program, we are not using any in-build Python function like __call__. So even if the callable() function returns True for the Demo class the instance of the Demo class is not callable.
We can see the error returned when the instance of the Demo class is created and called.
Code:
Output:
Example 3: When Object is not Callable
Code:
Output:
In the above program, the integer and string objects are not callable. Therefore, the callable() function returns False for them.
Related Functions in Python
You can read more about other Python functions from here
Conclusion
- The Python callable() function returns true or false depending on whether the object passed is callable or not.
- Python callable() function takes only an object as a parameter.
- If the callable() function returns true then also object calling might fail.
- If the Python callable() function returns false then the object is certainly not callable.