Python Choice
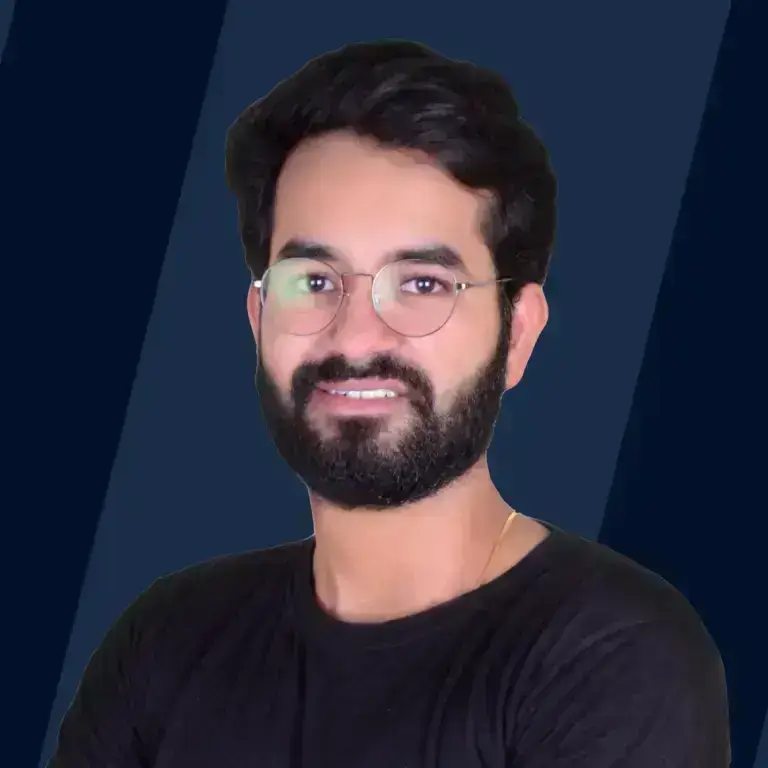
Overview
Some websites have a captcha verification when we log in to any website. The captcha is a randomly generated sequence of letters and/or numbers that appear as a distorted image. In the below image, you can see that there is a reset icon in the green box that will change the text of the captcha every time. Similarly, the Python choice() function randomly selects elements from a specified sequence.
Python choice() Function
The choice() function is an inbuilt function in Python. To use the choice() function in our program, we have to import the random module. The choice() function selects a random item from a specified sequence, and the sequence could be a list, a tuple, a range, a string, etc.
Let's understand the Python choice() function with a simple example:
In the below code, first you have to import the random module and then created a list of strings. Every time you run the code, the choice() function will randomly select a string from the list. The string would be the same or different every time.
Code
Output:
Explanation: In the above output, when I run the code for the first time, the Python choice() function produces an output InterviewBit. The second time, it produces Scaler. The third time it produces the same output as the first one, which means the choice() function selects elements randomly from the sequence.
Syntax of Python choice() Function
The following is the syntax for the choice() function:
First, we have to import the random module, and then we can access the choice() function.
Parameters of Python choice() Function
The choice() function takes only one parameter. It takes a non-empty sequence, which is a required parameter, and the sequence could be a list, a tuple, a string, a range of numbers, etc.
Return Value of Python choice() Function
The choice() function returns a random element from the given sequence. If the sequence is empty, it will raise an IndexError.
Exceptions of Python choice() Function
The choice() function also has some exceptions, that everyone should know. Let's discuss what are the exception of that Python choice() function.
TypeError
When we pass any number to the Python choice() function, it will throw a TypeError.
Code:
Output:
Explanation: In the above code, we are passing a number to the choice() function, so it will throw a TypeError.
IndexError
When we pass any empty sequence to the choice() function, it will throw an IndexError. An empty sequence means it could be an empty list, an empty tuple, an empty string, etc.
Code:
Output:
Explanation: In the above code, we have taken an empty list, so it will produce an IndexError.
Working of Python choice() Function
Say we have various foods in our bucket and we want to select two foods randomly to eat.
So, we will be using the choice() function to select the random foods from our bucket.
Code:
Output:
Explanation: As you can see, we executed the random.choice() function two times, and we got different foods as a result.
Tip: We can also use the random.choices() function to select one or more items from a list.
Let's see how we can use the random.choices() function to select one or more items from the list.
Code:
Output:
Explanation: As you can see, we have used the random.choices() function to select three items from the list at a time. Here, we have run the code twice. The first time we got different items, and the second time we got the same items, so it depends on whether it will select the same items or different ones, but it will select randomly.
Python choice() Function Examples
Example 1
In the below code, we have given a string, and now we will be using the choice() function.
Code:
Output:
Explanation: In the above output, we have run the above code thrice, and every time we are getting different outputs.
Example 2
In the below code, we have used the range() function to generate a list of numbers. The range() function takes the start and end points and generates the list of numbers between them.
Code:
Output:
Explanation: We can see that, first, the range() function would have generated a list of numbers between 19 and 99, and then, using the Python choice() function, we will select the random numbers from the list.
Example 3
In the below code, we have taken the boolean values. The boolean values are either true or false. Let's see how to choose a random boolean value using the choice() function.
Code:
Output:
Explanation: The result will either be false or true, as you can see above. The first time, the result is true, then false, and then true.
Example 4
In the below code, we have taken a dictionary. We will see how to use the choice() function to select random key-value pairs from the Python dictionary.
Tip: The Python choice() function doesn't accept a dictionary. We have to convert the dictionary into a list before passing it to the choice() function.
Code:
Output:
Explanation: First, we created a dictionary of employees, selected a random key from the dictionary using the choice() function, and last, we printed the key-value pair using the key name.
More: We can also select random elements from the tuple. Similar to the dictionary, we have to convert the tuple into a list before passing it to the choice() function.
Output:
Related Functions in Python
Read about a random module and its function from the below links:
Conclusion
Let's summarise our topic Python choice() function by discussing some important points.
- The Python choice() function randomly selects elements from a specified sequence. To use the choice() function in our program, we have to import the random module.
- Every time you run the code, the choice() function will randomly select a string from the list. The string could be the same or different every time.
- The following is the syntax for the choice() function: random.choice(sequence). The sequence could be a list, a tuple, a string, a range of numbers, etc.
- We can also use the random.choices() function to select one or more items from a list.
- The Python choice() function doesn’t accept a dictionary, tuple, or set. We have to convert the dictionary into a list before passing it to the choice() function.