Python Programs to Create Dictionary From Lists
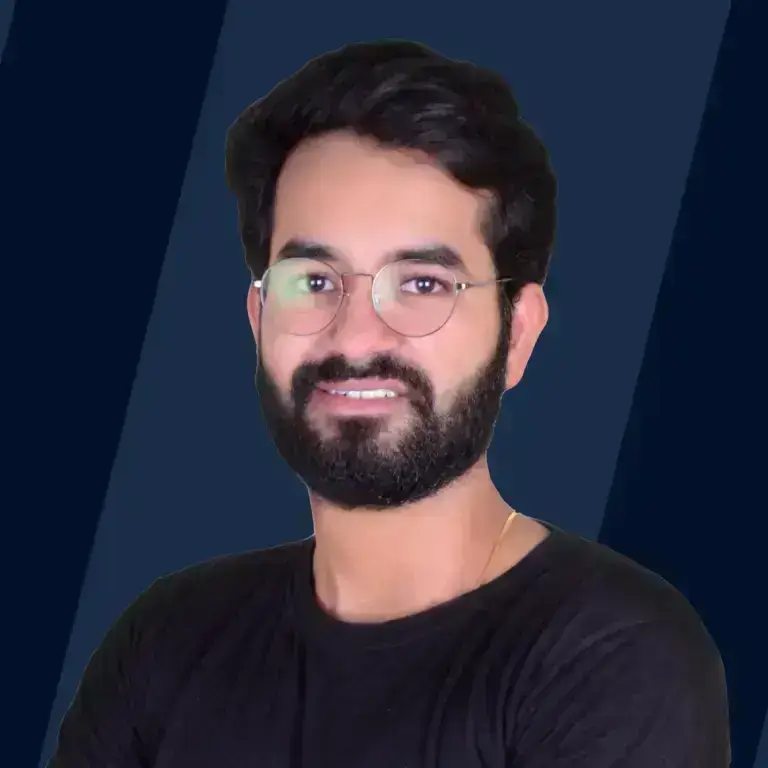
Overview
Interconversion between different data types is essential in real-time applications. Dictionary in programming is very useful as it is a collection of key-value pairs, unlike other data types which hold only a single value. Interconversion between different data types is essential in real-time applications. Lists are the most commonly used datatypes and dictionaries are the most applicable utility.
Converting Lists into Dictionary in Python
Interconversion of datatypes has very useful applications in computer programming and different systems support and require input and outputs in specific datatypes. Dictionaries find their application in almost every complex program written. Lists in Python are the simplest data type.
Lists in Python can have any type of data, supports duplicates, and are mutable i.e. elements can be added, deleted, or modified.
Dictionary in Python contains key-value pairs. These can be of any data. All the keys must be unique, but values for two distinct keys can be the same. Keys in the dictionary are immutable.
Different Methods in Python Create Dictionary From Lists
Let us now look at various ways to python create dictionary from lists.
Using For Loop
The brute force approach to python create dictionary from lists is to create a dictionary and use the for loop to add elements one by one into the dictionary. Let us take two lists containing roll numbers and names of the students. We assume that the data in the list are sorted and the index of students and index are the same.
Output:
In the above example, we are using for loop. In each iteration, we are inserting a new key for the dictionary from the rollNo list and for each key, we are giving the value from the names list.
Using Dictionary Comprehension
Dictionary comprehension is a concise way to python create dictionary from lists. It is a faster way to provide clean code by reducing the lines of code. We loop over the lists ranging from 0 to the length of the lists and in each iteration we assign the dictionary key and dictionary value from the lists respectively.
Let us create two lists consisting of product keys and products.
Output:
The for loop inside the dictionary's initialization goes from 0 to the length of the list. The range() function helps us iterate through the list along with the for a loop. The productKeys[i] is the key and the value after the colon : represents the value for the given key. We assign the keys and values using the direct assignment.
Using zip()
The above method discussed is still complicated and a little tricky to decode. Python provides another simpler and faster way to python create dictionary from lists.
The zip() function in Python pairs the list element with another list's elements at the corresponding index in the form of a tuple also called a zip object. This tuple is further converted into key-value pairs using the dict() function i.e. the tuples are mapped as keys and values.
Let us take an example of two lists one containing the list of countries and the other containing its capitals. We will convert these lists into a dictionary taking countries as keys and their capitals as values.
Output:
The zip() function combines the list of items corresponding to the respective index and adds it to the dictionary.
Using map()
The Lambda function can also be used to python create dictionary from lists i.e. to combine two lists. The map() function generates the tuple list, and this tuple list is cast into a dictionary using the dict() function.
Let us create a dictionary that contains the morning, afternoon, evening, and night and the respective greeting.
Output:
Lambdas are one-line anonymous functions. Here lambda simply takes two values x and y from the same index of time and dictionary lists and returns a pair. The map() function iteratively calls the lambda function for each value in the corresponding lists and pairs are created. Now, these pairs are then converted into keys and values using the dict() function.
Note: In the lambda function, the first variable denotes the key i.e x is the key and the next variable i.e. y denotes the value for the respective key.
Which is the best way to Create a Dictionary From Lists in Python?
The zip() function is the most efficient way to python create dictionary from lists from the pair of lists.
The first method is the brute force approach using only the for loop. It iterates over the entire list and one by one creates a key-value pair, which is then inserted into the dictionary.
The next method that we discussed was using the comprehension method which follows the same process except that the lines of codes are reduced in this process.
The third method was using the zip function. It is relatively less time-consuming as it does not iterate for each element, it simply converts all the items into the zip object which is then converted into the dictionary.
The last method we used was using lambda function. This method not only calls the lambda function for each iteration but also calls the dict function which adds to the time complexity of the function.
Thus, the zip() function takes a minimal amount of time and is the fastest of all the methods to convert lists into the dictionary.
FAQs
Q: What is one practical example of the list-to-dictionary conversion?
A: A practical example of conversion of a list to the dictionary is while fetching part of the data from the database. In case some entries are used, again and again, then the list is separated from the database and is stored in the cache memory in the form of the dictionary as it allows faster access to the data.
Q: Are there any other ways apart from these to generate a dictionary?
A: There are many other ways to convert lists to the dictionary like using a dict.fromkeys() function, using tuples, using enumerate() function, etc. Refer to the article to know more about them.
Q: Can we also create lists from the dictionary?
A: Yes, we can convert a dictionary into a list or tuple. Python provides various in-built methods like keys() which returns the list of keys, values() which returns the list of values, etc.
Conclusion
- Conversion from one data type to another is very useful, especially for commonly used data types.
- Multiple methods help to python create dictionary from lists
- One such way is to use a naive method i.e. using for loop.
- We can also use the comprehension method i.e. a concise one-line code to iterate through the entire list.
- We can also use the lambda function along with map() and dict() functions to achieve the functionality.
- Lastly, the most efficient way to convert a list into a dictionary is to use the zip() function that creates zip objects or tuples which can be further reduced to a dictionary using the dict() function.