Python Ctypes Module
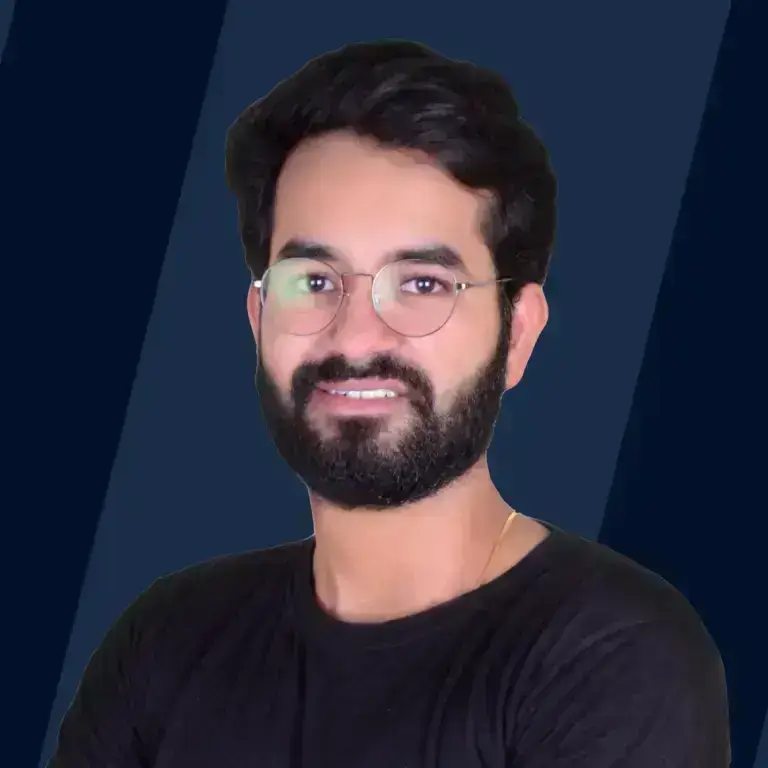
Overview
In this article, we will discuss the python Ctypes library. When programming in Python, we often have to use functions written in another language. Python is usually slower than languages such as C or C++ as it is mostly interpreted
The Python language offers a number of libraries that utilize C and C++ functions, such as NumPy and OpenCV. With the Python Ctypes library, you can import libraries like this and use their functions.
Let's take a look at how you, too, can do this in this Python Ctypes tutorial!
About Python Ctype Module
Python Ctypes modules provide C-compatible data types and allow calling functions to be located in dynamic link libraries or shared libraries. Python Ctypes is one of the most useful features of Python, as it allows you to leverage existing libraries in other languages by simple Python wrappers.
Python developers have access to the most powerful Python libraries through the Ctypes modules. By calling the python Ctypes functions in dynamically linked libraries, you are able to manipulate memory at a low level.
Importing a C Library with Ctypes
We will now try importing a basic library with only one function into our Python program by using the python Ctypes library. However, you cannot link your regular .c file. A shared library must be generated, which can be done with the following command.
Our C file is called "clibraryexample". Any name can be used, just keep the .so extensions in mind.
First, let's create our .c file.
In our Python file, we now need to import the Ctypes library and then load the shared library using the CDILL function. If the shared library is not located within the Python file, include the full path.
As a result of calling CDILL, we receive a library object, and we can use that object to access functions within the library.
Calling C Function from Python
Here are the steps to call a C function using Python Ctypes
- Creating a C file (with a .c extension) with all the necessary functions
- Using the C compiler, create a shared library file (.so extension).
- Using the shared file, create an instance of ctypes.CDLL in Python.
- The C function should then be called with the format <CDLL_instance>.<function_name>(<function_parameters>).
Step 1: Create a C file with some functions
There is a simple C function that returns an integer's cube. This function code has been saved as functions.c.
Step 2: Setting up the shared library
From the C source file, we can create the shared library file using the following command.
$ cc -fPIC -shared -o functions.so functions.c
Step 3: Invoking a C function from a Python program
Output:
The shared library file must be regenerated if the C program file is changed.
Using Function Signatures to Call Functions
Throughout this tutorial on Python Ctypes, we will discuss alternative methods of calling functions.
The following function will multiply two numbers together, and return the result in our C Library. This is a very basic multiplication function
Next, let's look at the Python file. Your first step should be to obtain the C function's signature. Like so.
multiplyTwoNumbers = clibrary.mul
The next step is to define the function parameters and the type of return from the function.
Parameter types are argtypes, while return types are restypes. The argtypes parameter takes a list since multiple parameters can be specified. However, restype only accepts a single value, since there can be only one return type.
The complete code:
Output:
When we try to add arguments that are against the predefined argtypes we will get the following error. For example, if we input 15.2 and 25.0 as input
Supported or Unsupported Data Types
C functions now allow you to modify the string. The C function will increments a number for each item in a string. In accordance with the alphabet codes, a character A will become B. This function takes a Python string:
Output:
C strings are not compatible with Python strings, so the output remains the same. The problem can be solved by using some Ctypes data types. Use a character pointer instead of a Python string. Using Ctypes data types, you can return a string from the char pointer using a Python native object.
Example
Output
Mutable Memory Creation with String buffers
It is important to understand that char pointers do not work with mutable memory, but with immutable memory. It will be mapped to a newly allocated memory location when a new value is assigned to it. Functions expecting mutable memory might encounter problems because of this.
Here’s an example that explains the same:
Output:
In the output above, you can see that each assignment results in a different address. Create string buffers using Ctypes to resolve this issue. In line five, replace "cstring = types.c_char_p" with "cstring = ctypes.create_string_buffer" and clibrary.increment(cstring).
The string has now been modified properly. By using Ctypes to create a string buffer, you will be able to create mutable memories for use with C functions. To create an empty string buffer of that size, you can also pass an integer instead of a string.
Example:
Output:
Using Pointer for Memory Management
It is also important to work on managing memory in Ctypes using pointers. Python does not support pointers as a data type. C and C++ both use pointers, but Python does not support pointers themselves. Using the Ctypes library, you can create a pointer in Python using Ctypes.POINTER.
Here are some examples of Ctypes pointer:
The first thing you need to do is declare two functions in your library, one for allocating memory and one for freeing it. To accomplish this, allocated_memory() and free_memory_available() are created.
Allocating memory and freeing it up when needed can be done using the allocating memory function.
Output
Examples of Understanding
Let us now look at an example of Python Ctypes to understand it even better. The following function will subtract two numbers together, and return the result in our C Library.
The following function will multiply two numbers together, and return the result in our C Library. This is a very basic multiplication function
C-types code to implement in python
Output:
Why You Should Use Ctypes in Python
Let us try to understand the performance boost of Python Ctypes with an example
Normal python code
The following results are obtained after execution:
Using Cytpes we run the same code
The Output would be
There is a 100-fold speed improvement over the original Python code using the C function. Obviously, this depends on the compiler optimization options we have used. The execution time is reduced by about half when I compile the library with the -O2 flag.
Conclusion
- Python Ctypes used to wrap C code in Python
- With Python Ctypes, you can call any shared or dynamic library written in C or C++ since it is already included with your Python installation.
- Python Ctypes also have the advantage of not requiring recompilation in order to be usable from Python.
- You must, however, be cautious with python Ctypes when it comes to memory management and ownership.