Python Decode() Function
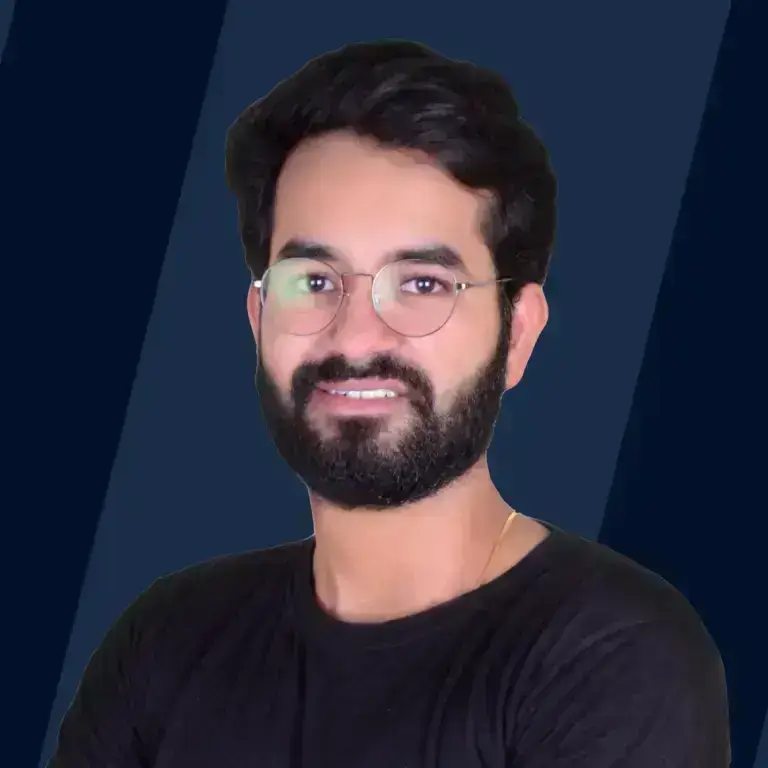
Overview
The python decode method is used to decode the encoded form of a string. The python decode uses the codecs that are registered for encoding. By default, the python decode uses the UTF-8 encoding value. It is used to convert bytes to string objects.
Syntax of Decode() Method
The following is the syntax of the python decode method:
- Here Str is the string that is being decoded.
Parameters of Python Decode() Method
The python decode method takes the following parameters:
- encoding: The encoding parameter refers to the type of encoding that is being used on the string. Some common types of encoding are UTF-8, cp866, iso2022_jp etc.
- errors: The errors parameter takes error handling methods as the value. This parameter decides how the error will be handled during the process of encoding or decoding. By default, it takes the value strict which means that the python decode method will raise a UnicodeError.
Note: Please visit python docs to learn more about different types of encoding values.
Return Value of Python Decode() Method
A string value.
The python decode method returns the decoded string.
Working of the Python Decode() Method?
The following flowchart shows the working of python decoding:
Uses of Python Decode() Method?
- The python decode method is used to decrypt messages.
- The python decode method is used for password matching.
- The python decode method is used to implement a safe message transmission over an open network.
Examples of Python Decode() Method
Decode a String
In this example, we will take the string python decode example and display the encoded and decoded strings using python decode.
Code:
Output:
Explanation of the example:
In the above example, we have taken the string python decode example, and then it is passed to example.encode(encoding='utf8') which returns an encoded string that is stored in encodedString. Then this encoded string is passed to python decode which returns the decoded string.
Encode-decode Implementation
In this example, we will create a small message verification system using the python encode and python decode functions.
The encode-decode in python can be used for message encryption. Message encryption is done when we want to send private messages that can't be snooped easily, thus we encode those messages and send that encoded form. These messages are then decoded and obtained in the original form.
Note: The given example represents the most basic case of message encoding-decoding in order to explain the implementation of encode and decode methods in python. The actual message encryption is a far more complex process that is outside the scope of the article.
Code:
Output:
Explanation of the example:
In the above example, we have two parts: the sender's end and the receiver's end. On the sender's end, we are taking an input message, then the inputMessage is encoded using the base64.b64encode(inputMessage.encode('utf-8')) and stored in the encodedMessage.
On the receiver's end, we are receiving the encodedMessage. Then the message is decoded using base64.b64decode(encodedMessage).decode('utf-8') and the decoded message is stored in receivedMessage. Thus, we obtain the output "Hello. This is a sample message to teach encoding and decoding in python" which is the same as the inputMessage.
Output:
Explanation of the example:
In the above example, we have a login_id and login_password. In order to make the system more secure, we are storing the password in an encoded form using the encodedPassword variable.
In the first case, the user is entering the login id as 'coder123' and the password as 'myPassword'. Now we will encode the stored password associated with this login id and compare. Since the input id and the original password did not match thus we will display sorry! You've entered the wrong password.
In the second case, the user is entering the login id as 'coder123' and the password as 'examplePassword'. Now we will encode the stored password associated with this login id and compare. Since the input id and the original password did not match thus we will display Welcome.
Related Functions in Python
Conclusion
- The python decode method is used to decode the encoded form of a string.
- The python decode makes use of codecs.
- The python decode converts bytes to string objects.
- The python decode by default uses UTF-8.