Python Program to Compare Dictionary
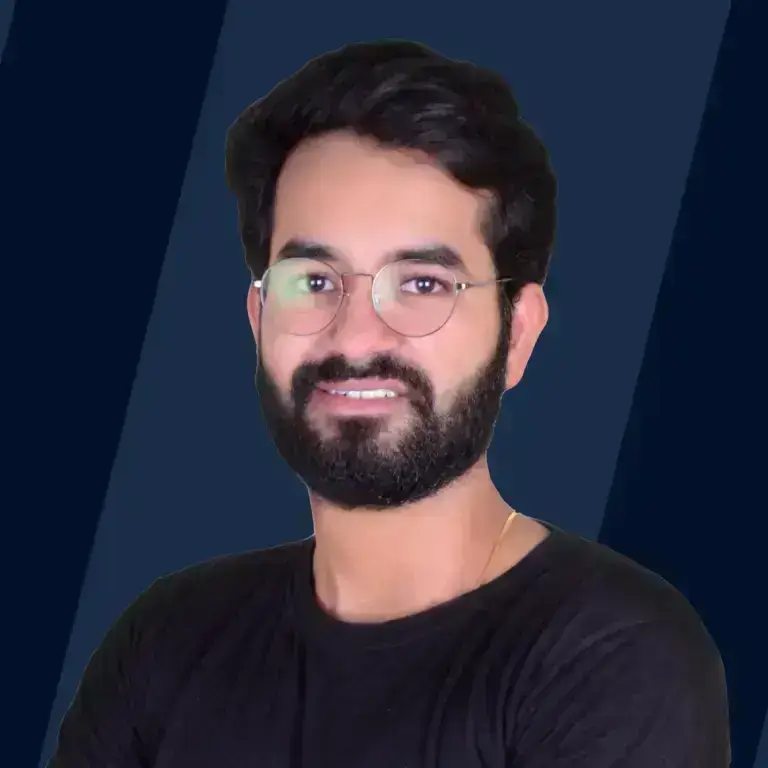
Overview
In this article, we'll see Python dictionary compare. However, before discussing that, let's recap dictionaries in Python. Dictionary is a data structure in Python that store data in key-value pairs. A dictionary is a collection that is, ordered (in and after Python version 3.7), changeable, and does not allow duplicate keys.
Now, we'll discuss different methods we can use to do Python dictionarie compare.
Python Dictionary Compare
Assuming the reader has basic knowledge of the dictionary data structure in Python, it is recommended to read the following Dictionary in Python. So, let's discuss different methods to perform Python dictionary compare.
1. Using == Operator
In this method, we'll use the equality (==) compare operator to compare two dictionaries. The equality operator checks whether both dictionaries contain the same key-value pair.
Code:
Output:
2. Using Loops
Here, we'll use for loop to iterate over the keys of a dictionary and check whether the same key-value pair exists in the other dictionary.
Code:
Output:
The first step is to check whether the lengths of both dictionaries match or not. Because if the dictionaries are of different lengths, then it's obvious that both are not equal.
After that, we compare the key-value pairs of both dictionaries. The dictionaries will be equal only if both contain the same key and values.
3. Using List Comprehension
In this method, we'll be using the concept of list comprehension. If you don't know about this concept, read this article, List Comprehension in Python. The basic idea of list comprehension is that it provides a short syntax for creating a new list based on the values of an existing list.
Code:
Output:
4. Using DeepDiff Module
The DeepDiff module is used to find the differences in dictionaries, iterables, strings, and other objects. Here, we'll use this module to do a Python dictionary compare.
However, before we can use the DeepDiff module, we need to install it. Type the below command in your terminal window.
Code
Output
The output is an object that shows the difference in the values of a key. If both dictionaries are the same the output will be an empty object.
Conclusion
In this article, we learned various ways in which we can do Python dictionary compare. The method we discussed:
- Using == operator: The equality operator can be used to compare two dictionaries. This is the most straightforward method.
- Using loops: We can use a for loop to compare two dictionaries by iterating over one and comparing it with the other.
- Using list comprehension: We can use this method to compare dictionaries if we want a very short code.
- Using DeepDiff module: Using the external DeepDiff module we can find the deep differences between many different kinds of data structures.
Now, it's your turn to apply these methods to your projects.