Difference between Two Lists in Python
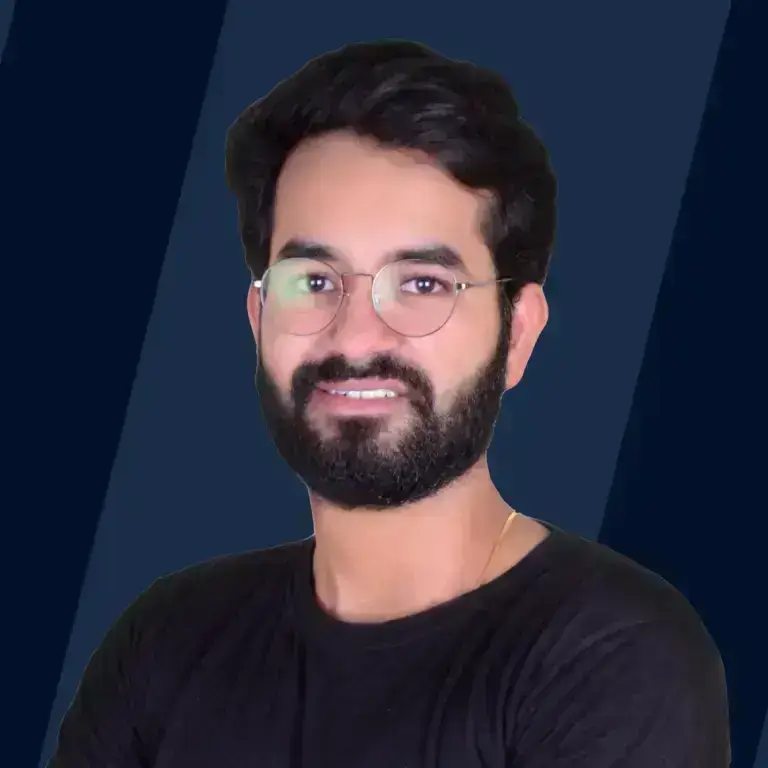
Overview
The difference between the two lists in Python (first list - second list ) results in items that are in the first list but not in the second list.
Similarly, the difference between the second list and the first list (second list - first list) results in the items that are in the second list but not in the first list.
Symmetric difference between the list gives the items which are either in the first list or the second list but not in both.
For example
First list = [1,2,9] second list = [8,7,9]
- difference between first list and second list = [1,2]
- difference between second list and first list = [8,7]
- symmetric difference between the lists = [1,2,8,7]
Method 1: Using “in” to Find the Difference Between Two Lists in Python
In this method to find the difference between two lists in Python, iterate over the first list using for loop, and for each item in the first list check if the item is present in the second list using the not in syntax of Python. If the item is not found in the second list then append the item to a new list.
Output
Method 2: Using set() to Find the Difference Between Two Lists in Python
We can use the set() method in Python to convert both into sets and then use the set. difference(s) to find the difference between two sets. Then to convert the set into a list we can use the list() method in Python.
This method cannot handle duplicate elements as the difference is calculated from the set of lists. The set allows unique elements so the duplicate elements in the list will not give the correct result.
Output
Method 3: Using List Comprehension to Find the Difference Between Two Lists in Python
A more compact implementation of finding the difference between two lists in Python can be done using the list comprehension syntax of python.
Output
Method 4: Finding the Difference Between Two Lists in Python Using Numpy
We can use the NumPy.concatenate() method to find the difference between two lists in python. The NumPy.concatenate() method concatenates a sequence of arrays along an existing axis.
Output
Method 5: Finding the Difference Between Two Lists in Python Using Symmetric_difference
The symmetric difference between two lists gives the elements which are either in the first list or the second but not in both. For example , the symmetric difference between [1,2,4] and [4,7,8] is [1,2,7,8].
symmetric_difference is used to get the elements that are either in the first set or in the second set. Firstly the list is converted to a set using the set() method in Python and then symmetric_difference is applied to the sets to find the resultant set. finally, the resultant set is converted back to a list using the list() method in Python.
This method cannot handle duplicate elements as the symmetric difference is calculated from the set of lists. The set allows unique elements so the duplicate elements in the list will not give the correct result.
Output
Conclusion
- Difference between two lists in Python gives the item which is in the first list but not in the second list.
- The difference between the two lists in Python can find out using the in syntax of Python.
- The list can be converted to a set and then the set difference can be used to find the difference between two sets and finally the resultant set can be converted into a list.
- List comprehension is a more compact syntax to find the difference between two lists in Python.
- Numpy can be used to find the difference between two lists in Python.
- symmetric_difference can also be used to find the difference between two lists in Python.
- Finding the difference using set and symmetric difference methods cannot handle duplicate elements in the list as these methods first convert the list to set which eliminates the duplicate elements.