File Handling in Python
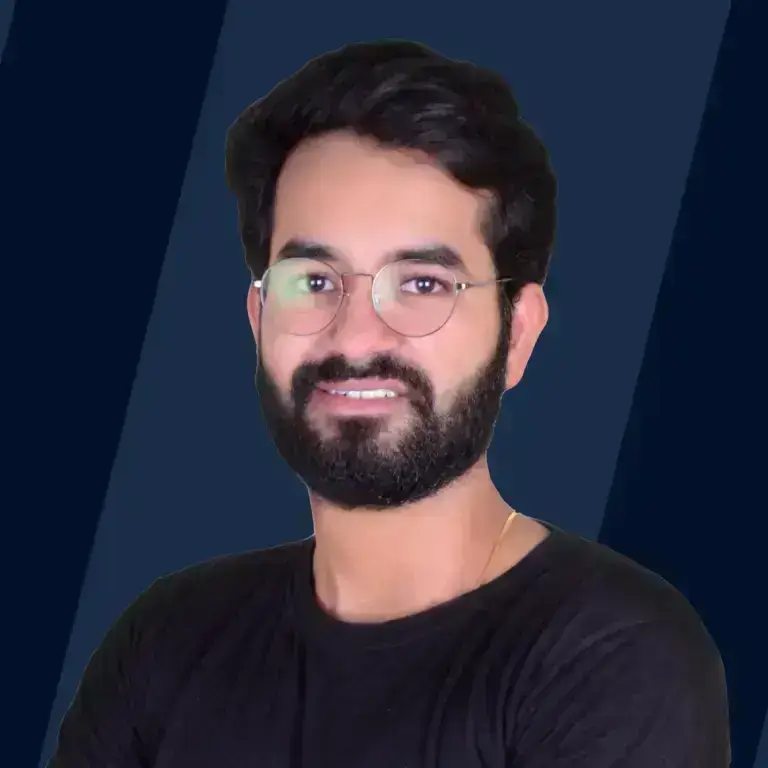
Python file handling is a versatile and powerful mechanism for performing operations on file. Python file handling allows users to work on different types of files and allows them to perform different operations on files. File handling is important as it allows us to store data in file after runing the program.
Advantages of File Handling in Python
Python file handling provides us following advantages:
- Versatility: Python file handling allows users to perform a variety of operations on files like creating, writing, reading, and deleting files.
- Flexibility: Python file handling provides flexibility as it allows to work with different types of files like binary, text, CSV Files, etc. and allows to perform a variety of operations.
- User–friendly: Python provides a user-friendly, simple and short way for file handling.
- Cross-platform: Python file handling can be performed on different platforms such as Linux, Mac, Windows, etc.
Disadvantages of File Handling in Python
Python file handling has the following disadvantages:
- Error-prone: Python file handling sometimes throws an error when the code is not written properly or if there is some issue in the file system.
- Security risks: Sometimes there is a security risk in python file handling especially when the input is taken from the user and it tries to modify and access the sensitive files of the system.
- Complexity: Python file handling becomes complex while working with advanced file types and operations.
- Performance: File handling in Python is slow in comparison to file handling of other programming languages, especially at the time of working with complex operations and large files.
Opening Files in Python
The open() method is used in Python for opening the file.
Example:
Let us create a file with the name example.txt, and insert the following data into it.
Now we will open the file in Python using the open() method.
In the above code, we have created the object of the file with the name f. This name will be used further for performing operations on file.
Files will be opened in read mode by default. f = open("example.txt") is equivalent to the code f = open("example.txt", "r"), in this we have explicitly specified the read mode for opening the file.
Various file opening modes are given below:
Mode | Description |
---|---|
r | read mode(default) |
w | write mode, if the file already exists erase its whole data then insert data, create a new file if the file does not exist then insert data. |
x | exclusive creation mode, this method fails, if the file already exists. |
a | append mode, inserts data at the end of the file without erasing the previous data of the file and creates a new file if the file does not exist. |
t | text mode (default) |
b | binary mode |
Writing to Files in Python
The write() method is used for writing data into the file, we need to open the file in write mode for writing data into it, and we need to pass w as the second of the open() method while writing to the file two things can happen:
- If the file does not exist, then a new file will be created and data will be written into it.
- If the file already exists, then the whole data of the file will be deleted, and new content will be inserted into it.
Example: Let us assume example1.txt does not exist, now we try to write content into it.
As example1.txt does not exist, so it will be created and the content passed in write() will be added to it.
Reading Files in Python
The read() method is used in Python for reading the file data. To read the file, we need to open it in read mode.
Output:
In the above code, we are reading the data of a file named example.txt which already exists in our system, and we are storing file data in the variable file_data, and then printing it.
Closing Files in Python
The close() method is used in Python for file closing, it is good practice to close after performing all operations on it, as it will release all the resources that are bound with the file.
Example:
Output:
In the above code we are reading file data, after that we close the file
Exception Handling in Files
If an exception occurs while working with the files, then the program will exit automatically without closing the file, so we should use try...finally block for the safer side.
Example:
Output:
Finally block will always be executed in both cases whether an error occurs or not, so we have closed the file finally block, if the code terminates due to the error then also file will also be closed.
Creating and Writing to Files
The write() method is used for writing the content inside the file, if the file does not exist then it will create a new file and insert content inside it., and if the file exists then it will erase all its data and then insert new content inside it.
Example: Let us assume the example2.txt file does not exist in our system.
The above code will create the file with the name example2.txt and insert Hey!!! Welcome inside it.
Append Mode in Python File Handling
If the file exists then append mode inserts the data at the end of the file, without erasing the existing data of the file, it will create a new file if the file does not exist.
Example:
As the example.txt already exists, so the data will inserted at the end of the example.txt file.
Implementing all the functions in File Handling
Below is an implementation of the Python file handling functions we have studied above, we have also used the remove() function of the os module which is used for the file deletion.
Output:
Python File Handling Methods
Various file handling methods are given below:
Method | Description |
---|---|
close() | close the file, if the file is already closed then it will do nothing |
detach() | It returns by separating the underlying binary buffer from the TextIOBase |
fileno() | file integer number (file descriptor) is returned by it |
flush() | it flushes the file stream write buffer |
isatty() | true is returned by it if the file stream is interactive |
read(n) | It reads the at most n characters of the data of the file, and it will read the whole file content if the n value is none or negative |
readable() | true is returned by it if the file stream can be read from |
readline(n=-1) | reads one line of the file and returns it. Reads in at most n bytes if mentioned |
readlines(n=-1) | reads a list of lines of the file and returns it. Reads in at most n bytes if mentioned |
seek(offset,from=SEEK_SET) | file position is changed by it to offset bytes, in reference to from (start, current, end). |
seekable() | It returns true if random access is supported by the file stream. |
tell() | returns an integer value which points to the current position of the file object. |
writable() | true is returned by it if the file stream can be written to. |
write(s) | string s is written to the file and it returns a number of characters written inside the file. |
writelines(lines) | A List of lines written by it inside the file. |
Conclusion
- Python file handling is a versatile and powerful mechanism for performing operations on the file.
- The open() method is used in Python for opening the file.
- The read() method is used in Python for reading the file data.
- The write() method is used for writing data into the file.
- The close() method is used in Python for file closing.