Python Getpass Module
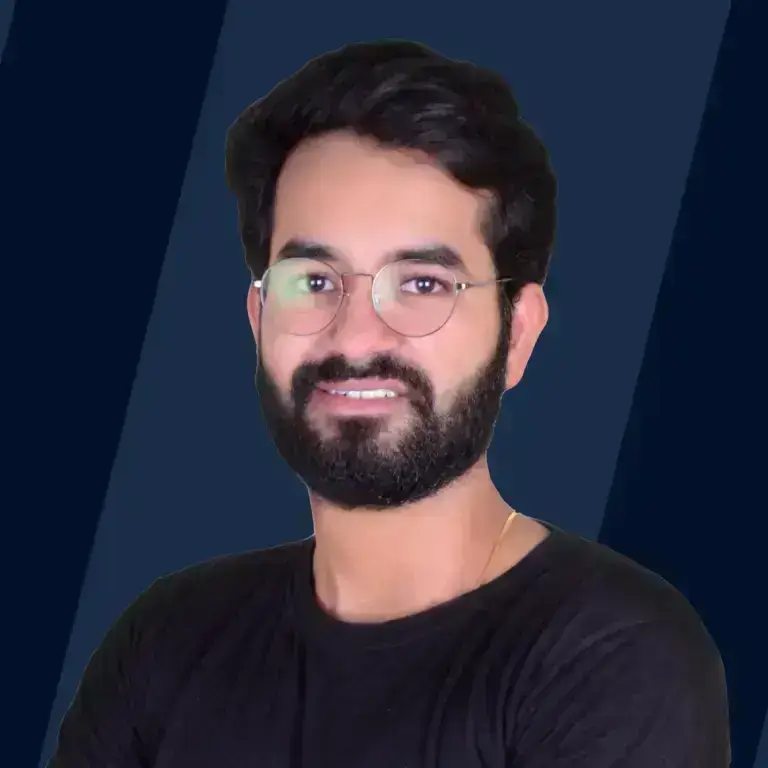
Overview
When we make an application in Python where we have to deal with confidential data that should not be displayed on the screen or made accessible to unauthorized people, we use the Python Getpass module.
The confidential data can be anything from secret keys to the user's login credentials. In this article, we'll see how we can use the Python Getpass module to hide such confidential data.
Introduction
The getpass module in python is an external package that we need to install using the pip command. The getpass module in Python prompts the user to enter the password without echoing.
The Python getpass module is used whenever we use a terminal-based application with various security credentials that require passwords before the execution of the application.
There are mainly two functions of the Getpass module that are required for a terminal-based application in Python. These functions are :
- getpass() function
- getuser() function
Functions in Getpass Module
As said earlier, the Getpass module has mainly two functions - getpass() and getuser().
These two functions are enough for validating a user and running the Python application only if a valid user login.
Let's discuss each one of these functions in detail.
getpass()
Utilizing the getpass() function, we can accept the password from a user in a Python program. When our application contains confidential data, we need to validate users before giving them access to the confidential data. The validation is done by the Python Getpass module.
Syntax :
The first parameter of the getpass() method is the prompt string, which defaults to 'Password: '. The second parameter is for UNIX-based OS and is ignored in windows, so we set it to none. Getpass() defaults to writing a warning message to stream, reading from sys.stdin, and issuing a GetPassWarning if echo-free input is not available.
Code :
Input - 1 :
Output - 1 :
Input - 2 :
Output - 2 :
Explaination :
The getpass() function of the getpass module is used to create a variable in the above section of code that stores the value of the key. Then we use an if-else statement to determine whether the input value matches the value in the variable and if so, unlock it for the user. Otherwise, run the code snippet written inside the else block.
Let's see different ways to use the getpass() method with examples.
Getpass without Prompt
In this section, we'll see how to get a password from users and return the same password without a prompt.
Code :
Input :
Output :
Explaination :
In the above example, we have imported the getpass module and declared a parameter that uses the getpass() method. However, we have not specified any parameter. We don't give any prompts in this method. We are just printing the password entered by the user in the next line.
Getpass with Prompt
The getpass() method with prompt, takes one parameter and that is the prompt. The prompt inside the getpass() method takes a message we want to be displayed on the screen when we take the user's input. The rest of the code is the same as in the case of getpass() without a prompt.
Code :
Input :
Output :
Explanation :
The working of this code is the same as explained earlier in the introduction of the getpass() method.
Getpass with Other Streams
Here, along with the Getpass module, we also use the sys module to access its stream keyword. Instead of prompt, we use the stream command. And it enables us to stream the password entered by the user.
Code :
Input :
Output :
getuser()
The getuser() method of the Getpass module is used to return the user's system login name. The function scans the user's system environment variables and the user name information is accessed. If the getuser() method is unable to access the environment variable, an exception is produced.
Let's see an example to implement the getuser() method.
Syntax :
The getuser() method doesn't take any argument. It returns the user login information from the environment. The getuser() function checks the environment variables LOGNAME, USER, LNAME, and USERNAME, in this particular order, and returns the value of the first non-empty string.
Code :
Output :
The output is your system's username. Like, my system's username is 'mav' so the getuser() method returned 'mav' in the above example.
Advantages of Using Getpass
There are several examples of using the Getpass module. Let's see some of them here :
- It takes user input without echoing it on the screen.
- We can also take the user's input using the input() method. However, that's not a secure method as your data will be displayed on the screen. The Getpass module hides the information you input.
- Can be used to deal with confidential information.
- Used in the validation of users.
Conclusion
This article discussed the Python Getpass module and its use cases. Let's take a quick recap of the article.
-
The Getpass module has two main methods :
- getpass()
- getuser()
-
getpass() :
The getpass() method is used to take user input without echoing it on the display. -
getuser() :
The getuser() method is used to get the system's user information. -
The python Getpass module is used in applications that deal with data that is confidential. Access to this data can only be granted after validating the user.