Python hasattr() Function
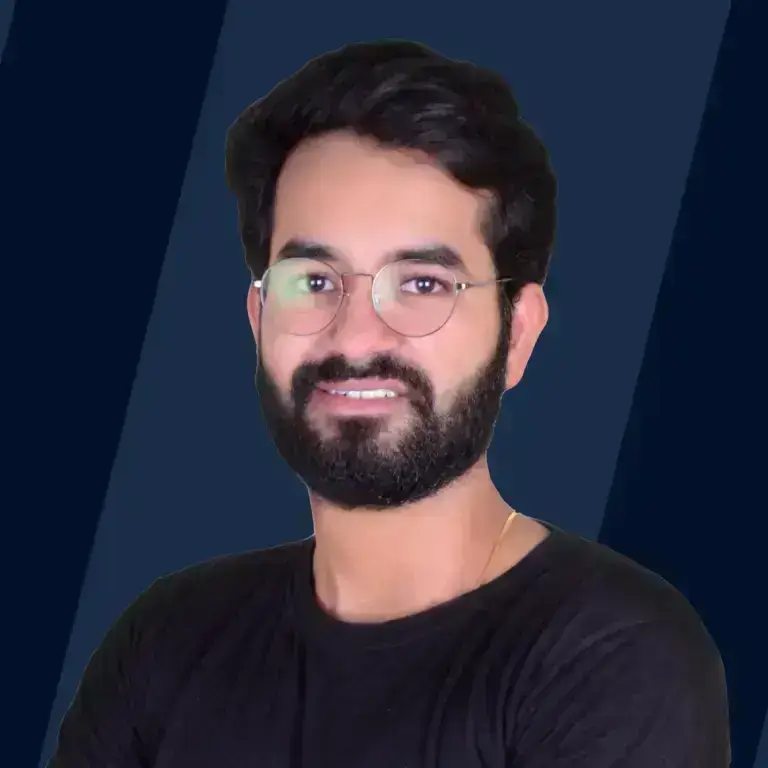
Overview
We deal with the representation of or mapping of real-world scenarios to Classes and Objects in the world of object-oriented programming. The objects can be viewed as a class's blueprint, showing both its characteristics and behavior.
Sometimes, we could get across situations where we need to verify the existence of an attribute that a Class is either holding or including. The Python hasattr() method can be used to do the same thing. It helps to determine whether an attribute is present in a Class or not.
In this article, we will discuss the operations of the Python hasattr() Function.
Python hasattr() Function
Python objects have identity, behavior, and state. The values of an object's attributes determine its state. The behavior is determined by the purpose of the object, and the identity is determined by its reference(s).
The Python hasattr() Function is used to check for the presence of an attribute within a Class. In other words, the hasattr() method returns a True if an object has the given named attribute and a False if it does not. The hasattr() function works for checking the presence of a method having a specific name on any given object.
When we are writing code that involves duck typing, the function hasattr() is useful.
FYI: Duck typing is a related concept to dynamic typing, where an object's type or class is not as significant as the methods it defines. Duck typing eliminates the need for any type of check. Instead, you verify that a specific method or attribute is present.
Syntax of the Python hasattr() Function
The hasattr() function is inbuilt in Python, hence does not requires any imports or installation. Below given is the syntax for the hasattr() Function.
The Python hasattr() Function takes two parameters, object and attribute name. Let us discuss in brief about them.
Parameters of the Python hasattr() Function
The Python hasattr() Function takes two required parameters. They are as follows:
- object : It is a mandatory parameter, and it is the object whose attribute is to be checked by using the Python hasattr() Function.
- name : The name is also a mandatory parameter, and it is the name of the attribute that is to be searched using the Python hasattr() Function. It must be of type string.
Return Value of Python hasattr() Function
The Python hasattr() Function returns boolean values (True or False), depending upon whether a certain condition is met or not. Let us discuss them below:
- True: The Python hasattr() Function returns a True if the object passed as parameter contains the name passed to the hasattr() function. In simple words, it returns True if the class contains the given named attribute.
- False: The Python hasattr() Function returns a False if the object passed as parameter does not contains the name passed to the hasattr() function. In simple words, it returns False if the class does not contain the given named attribute.
Exceptions of Python hasattr() Function
In general, the Python hasattr() Function does not raise any exception if we handle all the edge cases clearly and check whether or not the string (or name) passed to the hasattr() Function in Python exists in our code.
However, sometimes, we might pass something else to our hasattr() function instead of a string, that is the attribute name (suppose any attribute itself or something else), in those cases it can raise an AttributeError. This is because the hasattr() Function in Python expects a string as its second parameter.
Let us look at the below example to demonstrate this.
Code:
Output:
Explanation: In the above code, we have a class named class Student:. Inside that class, we have defined a method - def name(self, name). Now, using that hasattr() function, we try to check whether the attribute 'name' is present in our class or not. Our hasattr() function returns a True in this case because 'name' attribute is present in our class. Now, similarly, we check for 'age', and it returns a False because no attribute named 'age' is present in our class Student.
After that, we try to pass a non-string value job to our class, and our hasattr function returns a NameError: name 'job' is not defined because the 'job' we have passed to it is not a string. Hence, in this case, we can fall into the trap of exceptions in the hasattr() function. And eventually, get an AttributeError.
Python hasattr() Function Examples
Now that we have covered the Python hasattr() Function in detail, it is time for us to look into some code and get our concepts more concrete. So, let us cover all the code examples of the hasattr() Function in Python.
Example 1: When the Object of the Class has the Attribute
Let us take a scenario where our class has the attribute present and our Python hasattr() Function returns a True when the object of the class and attribute name is passed to the function. Let us look into the code for the same.
Code:
Output:
Explanation:
In the above code, we have a class named Book, class Book:. Inside the class, we have defined two methods, namely title and numPages. After that, we wrote the hasattr() function which checks whether the passed attribute is present or not in the object of the given class. Since, the attribute names(string) - 'title' and 'numPages' were present in our object, the hasattr function returned a True. However, since 'author' was not present in the given object, our hasattr() function returned a False.
Example 2: When the Object of the Class does not have the Attribute
Let us take a scenario where the class does not have the attribute present and our Python hasattr() Function returns a False when the object of the class and attribute name are passed to the function. Let us look into the code for the same.
Code:
Output:
Explanation: In the above code, we have a class named Hello. Inside the class, we have defined two methods, namely name and age. After that, we wrote the hasattr() function which checks whether the passed attribute is present or not in the object of the given class. Since the attribute named 'height' is not present in the given class Hello, our hasattr() function returned a False.
Example 3: Check the Class Attribute of the Class with getattr
Let us now take an example, where we will check whether any class attribute is present or not, by using the getattr method. Let us look into the code for the same.
Code:
Output:
Explanation: In the above code, we have a class named School. Inside the class, we have defined three class attributes, namely name, location, and website. After that, we used the hasattr() function which checks whether the passed attribute is present or not in the object of the given class. Since, the attribute names(string) - 'name' and 'location', and 'website' were present in our class, the hasattr() function returned a True.
However, since board was not present in the given class, our hasattr() function returned a False.
Applications of Using the hasattr Function in Python
The Python hasattr() function can be used to verify the keys, in order to prevent mistakes while trying to access keys that are missing. The hasattr() is also sometimes chained to prevent entering one associated attribute if the other attribute is absent.
Related Functions in Python
Now that you have got a clear and crisp idea about “Python hasattr() function”, I encourage you to go ahead and pick any of the below scaler topic articles to further enhance your knowledge in Python –
Conclusion
In this article, we learned about the " Python hasattr() function ". Now we have a better understanding of the requirements of the Python hasattr() Function, let us now go through the topics we covered and summarise them.
- The Python hasattr() Function is used to check for the presence of an attribute within a Class. The hasattr() works for checking the presence of a method having a specific name on any given object.
- The Python hasattr() Function takes two required parameters, object and attribute name.
- The Python hasattr() Function returns a True if the object has the given named attribute.
- The Python hasattr() Function returns a False if the object does not have the given named attribute.
- The Python hasattr() Function can sometimes raise an AttributeError if we do not pass a string as the attribute name to it. Or, if there are other bugs underlying in our code.
- The Python hasattr() function can be used to verify the keys, in order to prevent mistakes while we are trying to access the keys that are missing.