Python Logging
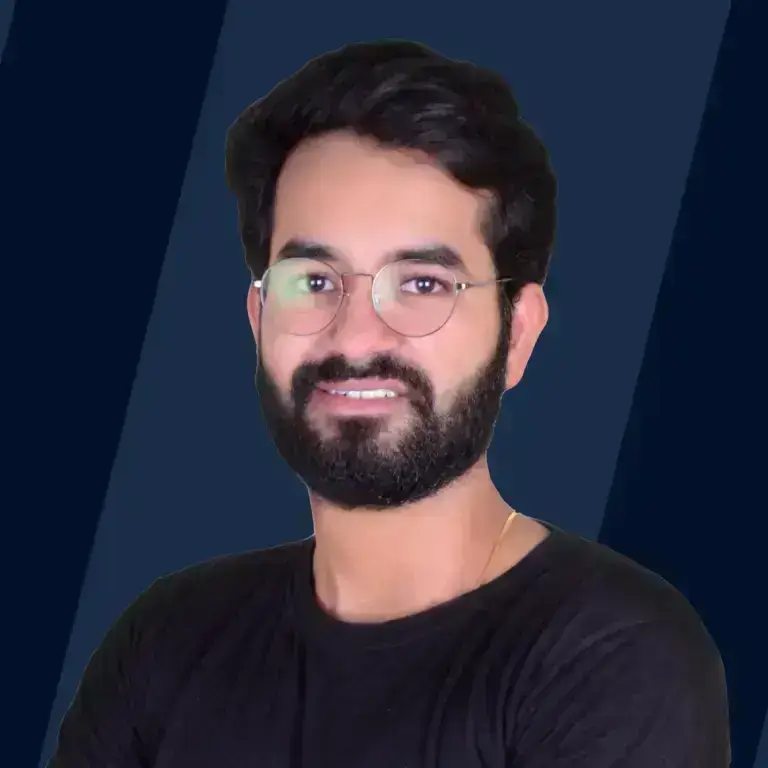
Overview
Logging is widely used in the software development process and software testing for debugging and future testing. Python logging is a built-in module that is used to store the log messages generated by Python programs into a file. The Python logging module contains several functions and methods that are used to log several events. We can use various methods to detect the error causing part in program execution and the exact problem. For logging a program, we first import the module, and then the logger must be configured. Finally, we can create an object of the logger and start using various methods.
What is Logging in Python?
Logging refers to tracking the events that occur when we run a particular program or software so that we can use the data for further improvement or error checking. Let us learn about Python logging.
Introduction
Python logging is a built-in module that comes with a Python interpreter. We can use the Python logging module to store the log messages generated by Python programs (or software working with Python frameworks) into a file.
Logging is widely used in the software development process and software testing process where developers log the running process of the program for debugging and future testing. We can store the log results in a file and can use it as a reference for other programs as well.
To understand the need for logging, let us suppose a situation where there is nothing like logging. We have developed a program having a large code base but it is crashing due to some reason that we need to find. Since the program is pretty large, we need to check every step of the program and this can be pretty hectic. We may not even get the exact reason for the problem.
In such scenarios, we use something like a logging tool that will trace the entire program execution and store it in a file so that we can refer to the file and get the exact problem. Logging saves us time as well because debugging is a time-consuming process.
How Logging in Python Works
Let us now see how Python logging works. Since it is a built-in Python module, we do not need to install it. We can start using it by importing it.
The Python logging module contains several functions and methods that are used to log several events. We can use various methods to detect which part of our code is causing the error in program execution and what is the exact problem. Let us look at the various logger objects present in the Python logging module.
- Logger.info(msg)- .info() is used with the INFO level to print log messages.
- Logger.warning(msg)- .warning() is used with the WARNING level to print log messages.
- Logger.error(msg)- .error() is used with the ERROR level to print log messages.
- Logger.critical(msg)- .critical() is used with the CRITICAL level to print log messages.
- Logger.log(lvl,msg)- It is used with the integer level lvl to print log messages.
- Logger.exception(msg)- .exception() is used with the ERROR level to print log messages.
- Logger.setLevel(lvl)- .setLevel() is used to set the current logger's level to the beginning. It ignores all the log messages that are logged below.
- Logger.addFilter(filt)- .addFilter() is used to add a filter filt to the current logger.
- Logger.removeFilter(filt)- .removeFilter() is used to eliminate a filter filt from the current logger.
- Logger.filter(record)- .filter() is used to put on the filter to the logger record. It returns True if the record is available and it can be handled else it returns False.
- Logger.addHandler(hdlr)- .addHandler() is used to add a picture handler hdlr to the current logger.
- Logger.removeHandler(hdlr)- .removeHandler() is used eliminate the handler hdlr from the current logger.
- Logger.hasHandlers()- .hasHandlers() is used to detect if the current logger has been configured or not.
The default logger is known as root. We can define our logger objects using the Logger class. Refer to the section Python Logging Classes and Function for more details.
Basics of Python Logging
As we have previously discussed that the Python logging module is used to log the events of execution of a program into a file. Let us now learn the basic configurations of the Python logging module.
We use basicConfig(kwarg) to configure the Python logging module. Let us look at the arguments of the basicConfig() method.
Syntax:
- level (optional parameter): It is used to specify the severity level which is set by the root level.
- filename (non-optional parameter): It is used to specify the file name.
- filemode (non-optional parameter): It is used to specify the opening mode of the file (i.e. read mode, append mode, or write mode). The default file mode is appended mode (a), which means that we can append data to the file.
- format (optional parameter): It is used to specify the format of the log file.
Example:
Output:
Python Logging Levels
Let us see some of the important events.
-
Debug: Debug is used to get detailed information but we usually used it when we perform diagnosing of the problem.
-
Info: As the name suggests info is used to get information about a certain thing.
-
Warning: Warning is used for something that has happened unexpectedly. It is also used when we can face problems in the upcoming time.
-
Error: Error is used in some serious cases such as the program has not executed the program or there is a major issue in the code.
-
Critical: Critical is used when we are dealing with some errors (a situation in which the program cannot execute the remaining program).
The above-stated levels are used in handling all the logging situations. Let us look at the corresponding numeric values of these levels.
Level | Numeric Values |
---|---|
NOTSET | 0 |
DEBUG | 10 |
INFO | 20 |
WARNING | 30 |
ERROR | 40 |
CRITICAL | 50 |
Here, the severity level or the number increases as the risk increases. So, larger the number greater is the concern.
Python Logging Classes and Function
We have seen in the Introduction section that the default logger is the root logger. It provides us with numerous useful functions and classes that are used to handle log messages and errors.
The classes start with a capital letter (for example: Logger), constants start in all caps (all letters are capitalized), and the methods or functions start in lowercase.
Let us look at some of the useful functions of the logging module before getting into the examples.
- Logger: We use the Logger object to directly call the logging module functions.
- LogRecord: LogRecord is used to create log record files that contain information related to various events such as the name of the logger, function, line number (that we need to log), and the log message, etc.
- Handler: Handler is used to dispatching the LogRecord to the output. There are various subclasses of the Handler class such as FileHandler, StreamHandler, HTTPHandler, and SMTTPHandler.
- Formatters: Formatters are used to specify as well as define the structure of the output log. It uses Python string formatting methods (fstring) to specify the log message format.
Example
Let us take an example, to sum up, things and for better clarity.
Output:
In the above program, we have first imported the Python logging module. We then created and configured the logger using the basicConfig() method by passing various parameters. We need to pass the name of the file in which we want to save the record of the events. We can also set the format of the logger so that the output can be generated in the desired format. Similarly, we can set the file opening mode. Finally, we have created an object, and using that object, we have called the various methods of the logging module.
Conclusion
- Logging refers to tracking the events that occur when we run a particular program or software so that we can use the data for further improvement or error checking.
- Logging is widely used in the software development process and software testing for debugging and future testing.
- Python logging is a built-in module that is used to store the log messages generated by Python programs into a file.
- For logging a program, we first need to import the module, and then the logger needs to be configured. Finally, we can create an object of the logger and start using various methods.
- The Python logging module contains several functions and methods that are used to log several events.
- Methods help us to detect which part of our code is causing the error in program execution and what is the exact problem.
- We use the basicConfig(kwarg) to configure the Python logging module.
- The classes start with a capital letter (for example: Logger), constants start in all caps (all letters are capitalized), and the methods or functions start in lowercase.