What is Python Metaclass?
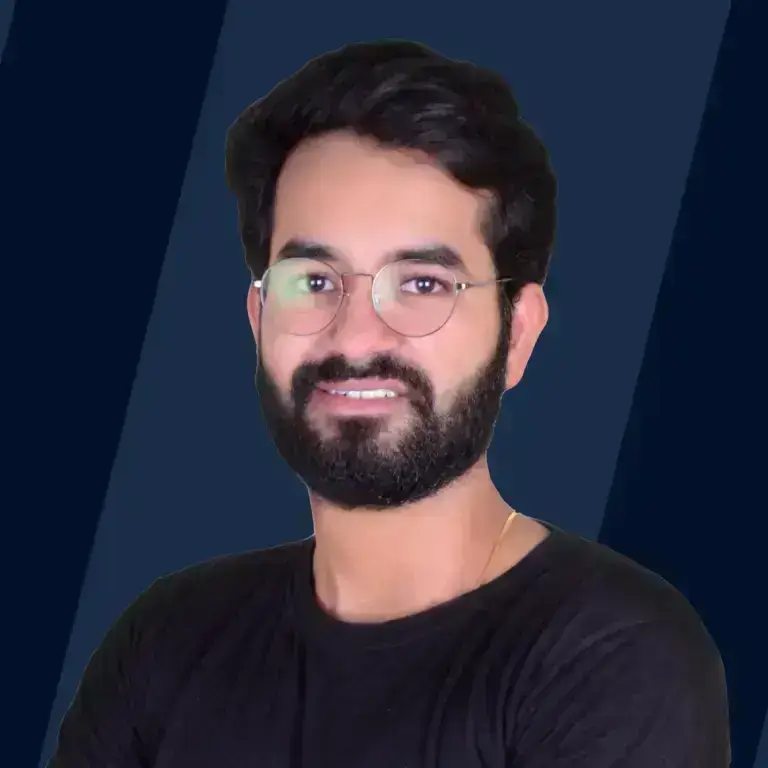
What is Python Metaclass?
Python metaclasses are a powerful tool for metaprogramming, which allows a program to modify its own structure and behavior. With this in mind, Python provides a feature called metaprogramming to support this capability through its Python metaclass classes.
Despite being an OOP concept, Python metaclasses find extensive use in the backend of almost all Python programs. They can be implemented either explicitly or implicitly, without the programmer being aware of it. You can instantiate a Python metaclass when you need to create classes that instantiate objects, and they create classes under the hood. This ability to create custom Python metaclasses enables you to manipulate the class creation process and instantiate classes differently than how the default "type" metaclass does it.
The process of changing the behavior of a program by purposely manipulating the code before execution starts is a well-known concept in Python called method decorators. Although decorators can manipulate Python functions before they are called, and metaclasses can modify classes before they are instantiated, both concepts share a similar idea of customizing program behavior through code manipulation.
Python metaclasses are a powerful tool for metaprogramming, which enables code to manipulate itself. They provide flexibility and customization to the class creation process, and their usage shares similarities with method decorators.
Let us start coding and understand the Python metaclass in detail.
Code:
Output:
Explanation: As seen above, we implemented the type() function to validate the type of the variable. As the type in Python is also specified by Class. Hence, then we create a new type by defining a class to it. As a Class is also an object we can call it an instance of Python metaclass. We then. find the type of Teacher class, and gets type as the type of it. As stated earlier a Class is also an object, we can modify them as well as we added to add, mul, div, or subtract fields.
By the above code example, we can come to a conclusion that Metaclass creates Classes and Classes create objects, they are all an instance of the other.
Pre-requisites for Python Metaclass
Listed below are the topics that must be revised before we can start learning about the Python Metaclass:
- Classes in Python
- Objects in Python
- Class instantiations in Python
- UDF in Python.
How to Create Custom Metaclass in Python?
Let us learn with this section of the article how we can create custom Python metaclass. We know that the custom Python metaclass inherits the type metaclass along with overriding it –
- init(): Should be implemented when you need to create an object and returns it in response. This method can easily override this method for controlling how the objects are getting created. Hence, you should be using the init() for initialize the already created object which could eventually be passed as a parameter.
- new(): Methods that are always called before.
Classes can be created by implementing the type() function directly. Listed below are the different ways in which these classes can be called:
- Scenario when you call the classes with only one argument, it shall then return the type.
- Scenario when you call the classes with three parameters, it then eventually creates a class. The arguments that can be passed here are:
- Class name.
- Base classes as a tuple inherited by class
- Class Dictionary: The class dict is treated as a local namespace to the class, where class methods and variables are populated.
Let us dive into an example to understand how we can Create Custom Python Metaclass:
Code:
Output:
Explanation: As seen above, we create a custom Python metaclass using the type() directly. We also created an instance for the sample class as shown above.
Now let us check another scenario where we shall again create a Python metaclass without implementing the type() directly.
Code:
Output:
Explanation: As seen above, we created a Python metaclass called 'SampleMulBases' that helps to validate the scenarios where when a class is created does it contain the inherited from more than one base class or not? If the scenario statement holds true, an error is raised as shown above.
When Should Python Metaclass be Used?
You might be thinking, when can we start using the Python Metaclasses with many scenarios stated as studied above? Indeed, Python metaclass is not implemented that rapidly in practice. It is so, as many other ways of finding solutions to the problems as compared to implementing the use of Python metaclass. As with decorators, we get the ability to find the solution as a generally powerful tool that satisfies the criteria easily and is enough.
But it is good that we came to this question, where we need to understand where can we implement the python metaclass and reap its benefits as an understanding of Python programming and the majority of the backend workings is important to make the right decisions on how and when to implement the right tools.
For scenarios where you already have to deal with many classes having a common base class, then implementing the Python metaclass is the right way to permeate through inheritance. Hence, for use cases where you need to change how a class instantiates, Python metaclasses are the right tool to implement for this job. Also, in this scenario decorators won't give you the same expectations as Python metaclass as the class is already instantiated before anything can be used with a decorator.
Using Python Metaclass to Solve Problems
A wide variety of problems can be resolved easily with decorators (easily) and by implementing Python metaclass. While certain specific problems can only be solved by Python metaclass.
Listed below are a few use cases of Python metaclass:
- Python metaclass finds a lot of inspiration during API development.
- Python metaclasses drill down to the inheritance hierarchies as well where it highly influences all the subclasses too. hence, for similar use cases, always opt for metaclasses as your solution.
- If at the time of creation there is a requirement to switch classes automatically, it is recommended to implement the python metaclass.
- Widely used for creating a registry for classes with Python metaclass.
- Implemented for logging and profiling, interface checking as well as automatically adding new methods.
Below is the code example where we work towards the easy problem of code repetition as shown below. We shall be defining class methods where our main goal would be to print its fully qualified name while the class method is running before executing the body.
Code:
Output:
Explanation:
As seen above, the purpose of the problem statement is to print its fully qualified name while the class method is running before executing the body. The way we executed it with decorators is fine but can we apply this same decorator method to all subclasses by inheriting the Operation class.
Let us find out with the below-given code:
Code:
Output:
Explanation: As seen above, we separately implemented the decorator method for each sub-class as we did for the Operations class. When we have various subclasses, then we should always refer to Pythonmetaclass over the decorator's method for every class separately. when we already know that each of the subclasses needs to have to debug features, then it recommended opting for a metaclass-based solution. As from the above code example also, every class is normally carried as before but each is immediately wrapped up by debugging decorator method.
Learn More
You could also learn more about Python programming by visiting a few of the below-listed topics:
- Python pygame.time.Clock() function
- Python random.randrange(start, stop, step) method
- Pygame .blit() method
- time.sleep() in Python
- pygame .midtop() function
- for loop in Python
- if statements in Python.
- if- else loop in Python
- .insert() in Pygame
- .fill() in Pygame
Conclusion
-
When we have various subclasses, then we should always refer to Python metaclass over the decorator's method for every class separately. when we already know that each of the subclasses needs to have to debug features, then it is recommended to opt for a metaclass-based solution.
-
If at the time of creation there is a requirement to switch classes automatically, it is recommended to implement the Python metaclass.
-
init()Should be implemented when you need to create an object and returns it in response. This method can easily override this method for controlling how the objects are getting created. Hence, you should be using the init() for initialize the already created object which could eventually be passed as a parameter.
-
new(): Methods that are always called before.