Parallel for Loop in Python
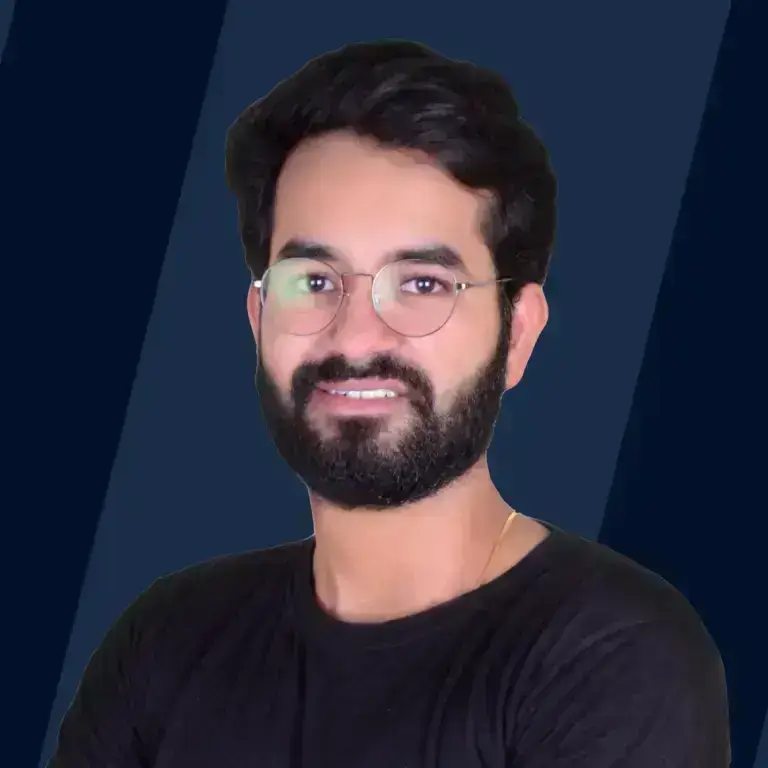
Overview
Python parallel for loops helps to spread processes in parallel using multiple cores. In presence of numerous jobs, parallel processing uses different processors without waiting for the completion of the previous job.
Introduction
The concept of Python parallel for loop is one of the most popular concepts to express parallelism in parallel languages and libraries. The Python Parallel For loop is similar to the for loop with the only exception that it allows the iterations to run in parallel across multiple threads.
What are Parallel Loops?
A loop whose iterations are executed at least partially concurrently by several threads or processes is called a parallel loop.
Need to Make For-Loop Parallel
A Python parallel for loop is a loop where the statements in the loop can be run in parallel: on separate cores, processors, or threads. Python parallel for loop is important as they
- Speed up the overall processing time
- Improve data processing performance
Method1: Use the Multiprocessing Module
multiprocessing. Pool().map() is a good choice for parallelizing simple loops. To parallelize the loop the multiprocessing package provides a process pool with helpful functions to automatically manage a pool of worker processes. By default, the created Pool class instance uses all available CPU cores. The parallel version of the built-in map() function takes a single argument. It calls the same function for every data in the provided iterable and returns an iterable of return values from the target function. Once all the work is done using the close() function the pool_obj is closed to release the worker processes.
Code
Output
Method 2: Use the Joblib Module
Joblib is a set of tools to provide lightweight pipelining in Python. It mainly aims to avoid computing the same thing twice. To perform parallel processing, we have to set the number of jobs, which is limited to the number of cores in the CPU. The delayed() function delays the mentioned method call for some time. The Parallel() function creates a parallel instance with the specified number of cores.
Code
Output
Method 3: Use the Asyncio Module
The asyncio module is single-threaded that runs loops by suspending the sequence temporarily using await methods. The main function and called functions run parallelly without affecting each other. The loop also runs in parallel with the main function.
Code
Output
Conclusion
- Python parallel for loop is a loop whose iterations are executed at least partially concurrently by several threads or processes.
- Using Python parallel for loop helps to spread processes in parallel using multiple cores. The For Loop in Python can be parallelized using the
- multiprocessing Module
- joblib Module
- asyncio Module