What are Partial Functions in Python?
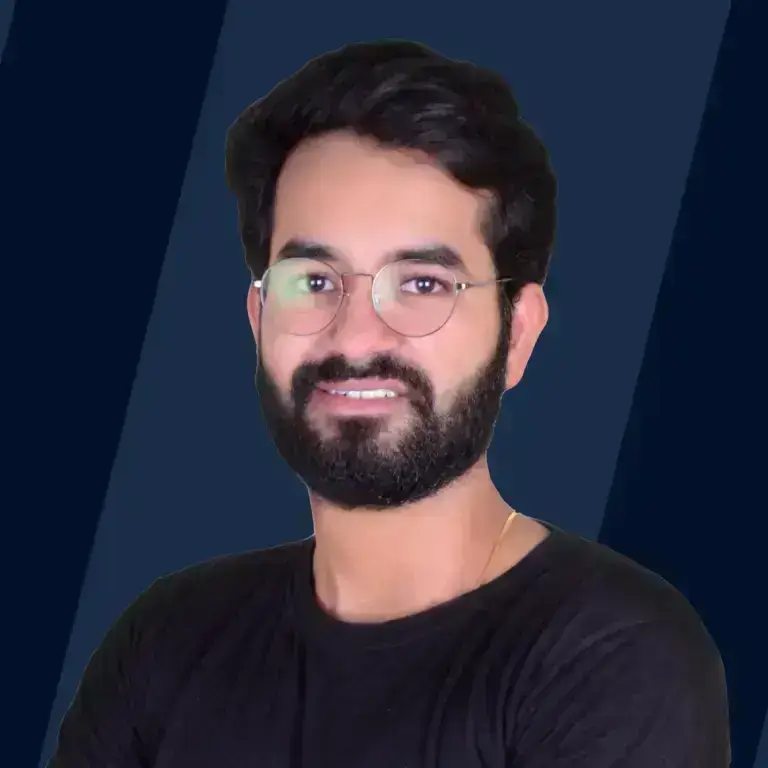
Partial functions are used to derive a specialized function with fewer parameters from the original function by setting constant values to a certain number of arguments. Python Partial functions make code more reusable without altering the original function. Partial functions in Python are created by importing the functools library.
Python Partial is a higher-order function that takes a function as its first input and returns a function that can be used as any other function in the program.
Partial functions support both positional and keyword arguments to be used as fixed arguments. The default values start replacing variables from the left.
Examples of Partial Functions
Example 1
Code
Output
Explanation
In the above example, since the default values start replacing variables from the left, the exponent value is pre-filled as 2 for the square function and 3 for the cube function. Functions square() and cube() take the base value as the only argument.
Example 2
Code
Output:
Explanation
In the above example, the keyword argument is used as the fixed argument. Here, in functions welcome() and holidays(), the message argument is frozen by setting values. Though the original function accepts two arguments, the two Partial ones accept a single argument, the name.
FAQs
Q. What is a Python Partial function?
A. A function that derives a specialized function with fewer parameters from the original function by setting constant values to a certain number of arguments is called a Partial function.
Q. Which Python module do you need to import to use Partial?
A. Python Partial functions can be easily created by importing the functools needs module.
Learn more
Conclusion
- Partial functions help in generating specialized functions from general functions. It increases code reusability.
- The Partial function in the functools library is used to create Partial functions.
- Python Partial is a higher-order function that takes a function as input and returns a function.
- Partial functions are very useful tools in situations where one of the arguments of the original function needs to be constant.