Python Pprint Module
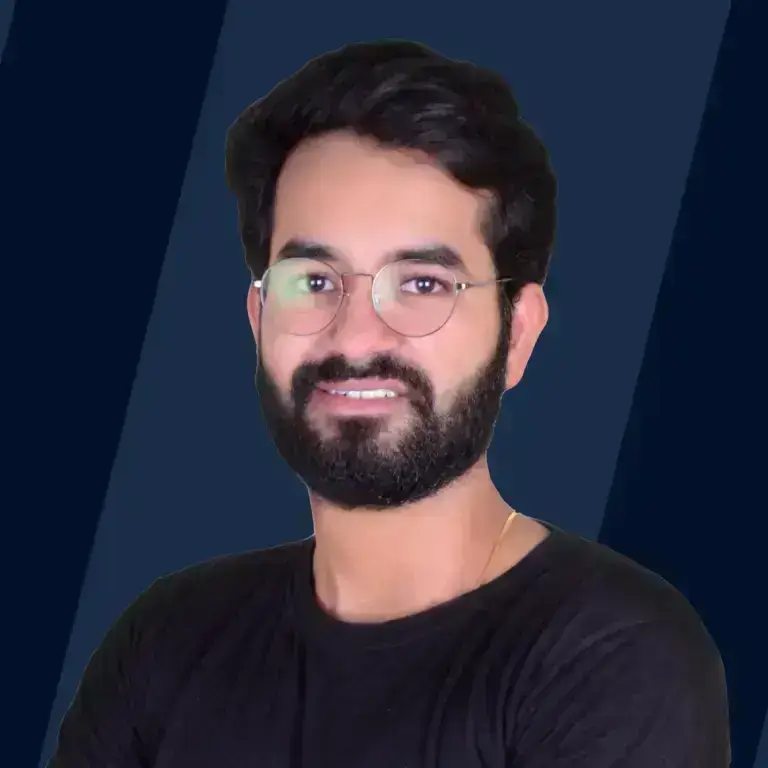
Overview
Python’s print() method is used by programmers to see the output of variables. However, if you use this method to print a variable containing a large amount of data, like JSON dictionary, nested lists, data from API requests, etc, this method will print everything in the same line. Developers need to indent the output so that they can understand the result. This can be done manually, or using the Python way, which is using the Python pprint from the module pprint. The Pprint module provides a way to use the Python pprint() that “pretty-prints” your variables.
Introduction to Python Pprint Module
Python Pprint stands for Pretty Printer. It is a module provided by Python to format your output. It provides multiple properties to customize your output.
To understand the need for this function, consider a response from a URL request. The URL will fetch the details of 10 users.
Output
When the above Python program is executed, all the data is printed onto a single line. Now computers have no problem with this format. However, for humans, this representation of data is messy and not readable.
To make this readable so that humans can understand, we use the Python pprint() function from the module pprint.
Output
Note that the keys of the dictionaries are sorted alphabetically. This is not the property of a Python dictionary. This is done by the Python pprint() function. Using Python pprint(), you can see that the output has been formatted using the default settings. The output is printed cleanly and is human-readable. Developers use this function to print the data structures like dictionaries, strings, lists, etc, as it makes debugging easier.
Working with Python Pprint Module
To start using this module, you need to import the module pprint using the following line.
Now, you can use the function pprint.pprint() to “pretty-print” your data. You can also create an object of the class PrettyPrinter() and customize it according to your requirements.
Output
The advantage of creating your object using the class Pretty Printer is that you can provide custom arguments for formatting the output while creating the object, which will be used throughout the code.
These arguments can also be provided inside the pprint.pprint() functions. However, the settings are not stored, so each function call is independent of another.
Optional Parameters within Python Pprint Module
Python allows various optional parameters that can be passed to the function pprint.pprint() or to the class PrettyPrinter on creation. These parameters allow you to format the output according to your own needs.
Depth
Python pprint() provides a parameter Depth that determines the number of depth levels (for nested data types) to be displayed. The default is to show all the data. When a value is provided, the data beyond the limit is displayed as ....
Example
Output
In the output, the dictionary is printed to depth 1, which represents only the keys. Since the value of the keys is a list, which is another level of nesting, it is represented as ....
Indent
Python pprint() provides a parameter Indent that is used to provide the number of spacing for each line. This is used when a specific type of formatting or linting rules are implemented in your code.
Example
Output
In the output, each new line has an indentation of 4 spaces.
Width
Python pprint() provides a parameter Width that is used to limit the maximum number of characters in each line. If the line exceeds this value, then the remaining characters will be wrapped in the lines below.
Example
Output
In the output, we see that the words “Data” and “science” are printed on different lines.
Compact
Python pprint() provides a parameter compact that takes a Boolean value True or False. When set to True, this property makes the output wrap on the next line, if it exceeds the width limit. This property can only be applied to lists, sets, and tuples. It cannot be applied to dictionaries. This property is useful for long sequences with short elements. It makes the output more readable.
Example
Output
In the first output, every name is printed on a different line. The output uses unnecessary space on the terminal.
When compact is set to True, the names are printed on the same line until it does not exceed the width specified in the argument.
Stream
The stream parameter, provided by Python pprint(), is used to redirect the output of pprint() to a file object or any other descriptor. It can act as a logging feature that sends pretty logs to a file.
Example
The output is redirected to the file “logs.txt” as shown below.
Output
Sort_dicts
When a dictionary is printed using the pprint() function, the keys are sorted alphabetically. In Python, dictionaries are unordered. To prevent the function pprint() from sorting the keys, we can set this parameter to False.
Example
Output
pprint.pprint() Object in Python
The function pprint.pprint() is the most used function from the module pprint. It can be used to print dictionaries, nested lists, and JSON data in a clean and readable manner.
Example
Output
pprint.pformat() Object in Python
The function pprint.pformat() is similar to the function pprint.pprint(). The main differences are:
- pprint() function sends the formatted data to the stream (stdin, stdout, etc) while the pformat() function returns the string with formatted data.
- pformat() function does not accept stream as a parameter.
Example
Output
In the above example, the string representation of the first user is returned by the function pformat() which can be printed by the print() function.
pprint.pp() Object in Python
The function pprint() from the module pprint has an alias pp. For developers who prefer less code, this can be used. This alias is available from Python3.8 and above.
Example
Output
pprint.isreadable() Object in Python
The function pprint.isreadable() is used to check whether an object can be pretty-printed by the function pprint.pprint() or not. If an object is readable, it can be pretty printed.
Example
Output
In the above example, the object person_obj is not readable by the pprint() function, hence we get the value False. The dictionary is readable by the pprint() function, so we get the value as True.
pprint.isrecursive() Object in Python
The function pprint.isrecursive() returns true if the object has a recursive structure.
Example
Output
Conclusion
- Python provides the module pprint that contains the Python pprint() to format your output in a human-readable manner.
- Many arguments like width, depth, indent, etc can be used to specify the rules of formatting using the Python pprint().
- A custom object can be created with the linting rules using the class PrettyPrinter. These rules will be applied automatically whenever the pprint() method is called using the object created.
- Python pprint.pformat() can be used to return the string representation of the formatted output.