Python Program to Find Product of List
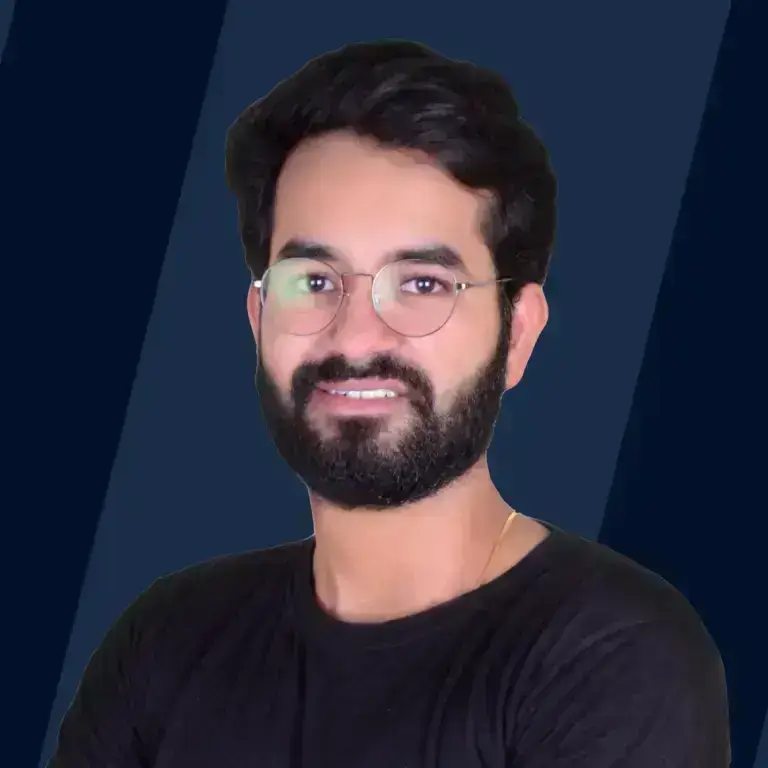
Overview
We can calculate the Python product of list in many ways. We can either create our function from scratch to calculate the Python product of list elements or we can use Python built-in methods to do the same.
How to Find Product of List in Python?
Without wasting any further time, let's dive right into the different ways to find the Python product of list. Below is an example to depict what we have to do.
Example
a. The Traversal Method
In this method, we follow a straightforward approach. We iterate over the input list and multiply its elements and save the result in a variable.
We set the product's value to 1 at the beginning (not 0 as 0 multiplied with anything returns zero). Continue through the list to the end, multiplying each integer by the product. Your ultimate answer will be determined by the value that is retained in the finished product.
Below is the implementation of the discussed viewpoint.
Code
Output
b. Using numpy.prod()
To multiply all the numbers in the list in Python, we can use the numpy.prod() function of numpy module. Depending on the outcome of the multiplication, either an integer or a float value is returned.
Below is the implementation of the discussed viewpoint.
Code
Output
c. Using reduce() and Lambda Function
Python's reduce() function accepts two arguments: a function and a list. A list and a lambda function are used to invoke the function reduce(), and a new reduced result is returned. This repeatedly operates on the list's pair elements.
Code
Output
d. Using math.prod
Earlier, we saw how to find the product of list elements using numpy.prod() and in this section, we'll see how to work with math.prod(). The math.prod() function doesn't require you to install any external module as we do in the case of numpy.prod().
Output
e. Using mul() Function of Operator Module
In this method, first, we have to import the operator module, and then we can use its mul() function to get the Python product of list.
Code
Output
f. Using Traversal by Index
Earlier we saw a traversal method in which we traverse the list using for each loop and we directly get the value of the list elements. However, in this section we'll see the use of range in for to iterate over the list, and using the index we'll get the Python product of list elements.
Output
g. Using itertools.accumulate
Output
Conclusion
In this article, we discussed seven different ways by which we can find the product of list elements. The methods are:
- Traversal Method: Using a for each loop to traverse over the list elements and find the product.
- numpy.prod(): Here, we utilize external Numpy library and its prod() method to find the product of list elements.
- reduce() and lambda: We use reduce() method to find the product of list elements. It takes two arguments: a list, and a lambda function.
- math.prod(): It's an in-built function in python, that we use to find the product of list elements.
- mul(): This method is present in operator module of python that we have to import to use mul() function.
- Traversal by Index: In this method we use a simple for loop to iterate over a list and use its index to access list elements and find the product.
- itertools.accumulate: This method takes in two parameters: a list and a lambda function to find the product of list elements.
Now, it's your turn to implement each one of these methods into practice. Knowing how to find the product of list elements can come in handy while problem solving.