Python Program to Solve Quadratic Equation
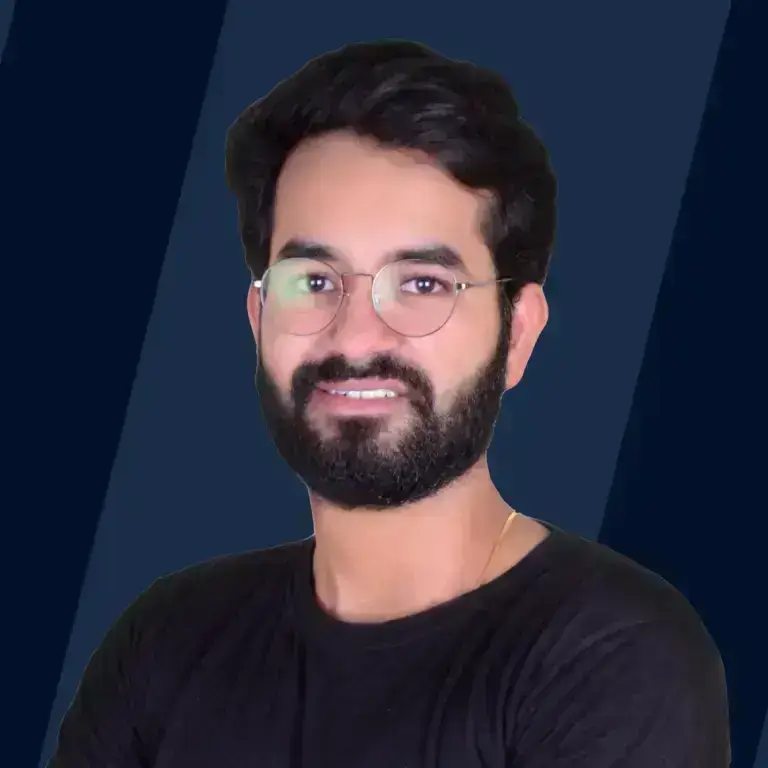
Overview
A Quadratic Equation is of the form a x ^ 2 + b x + c = 0 where, , , and are coefficients and . To solve a quadratic equation means to find the values of , which can be done using the quadratic formula.
We can write Python program to solve quadratic equation using the sqrt() function in math and cmath module.
What is a Quadratic Equation? How to Solve it?
A quadratic equation is a polynomial of degree 2 in one variable (generally ), it is generally represented as , where is the leading coefficient, is the coefficient of , and is the absolute term of .
In this equation, cannot be , because then the degree will no longer be and it won't be a quadratic equation.
A quadratic equation will always have two roots either both real or both complex ( and ) assuming its coefficients are real.
When a quadratic polynomial is equated to , it is called a quadratic equation.
The general form of a quadratic equation is:
Here are some examples of quadratic equations:
- , here is , is , and is .
- , here is , is , and is .
- , here is , is , and is .
A quadratic equation can be solved using the quadratic formula, which is,
This formula can also be written as,
Where, .
is called the discriminant, as it determines the nature of the roots, here are the cases:
- If : The roots are real and different.
For example, of the quadratic equation is and its roots are and . - If : The roots are real and the same.
For example, of the quadratic equation is and its roots are and . - If : The roots are complex and are conjugate pairs.
For example, of the quadratic equation is and its roots are and .
The first root of a quadratic equation is called alpha () and the second root is called beta ().
Note: In complex numbers, a conjugate pair is a pair of numbers whose product is a real number.
For example, and are conjugate pairs as their product is a real number.
Python Program to Solve Quadratic Equation
We will now implement the quadratic formula in Python to solve a quadratic equation of user input.
In the Python program, we will ask the user to input , , and , as we can build a quadratic equation using these coefficients.
Note: A complex number is of the form , but in Python a complex number is represented as ( represents in Python).
Method 1: Using Quadratic Formula Directly
In this method, we will use the sqrt() function from the math module to calculate the discriminant .
There is a problem, the math.sqrt() function cannot calculate complex numbers which is necessary to find out complex roots of a quadratic equation. A workaround for this will be to find the square root of abs(D) and then multiply it with if .
Code
Output 1
Output 2
In the above example, we are taking the quadratic equation from the user as input and then calling the QuadRoots() function to return the roots of the quadratic equation. Inside the function, we are calculating the value of the discriminant to determine the nature of the roots. We have then used the sqrt() function from the math module to calculate the roots of the quadratic equation.
Method 2: Using The Complex Math Module
In this method, we will use the cmath.sqrt() function from the cmath module to calculate the discriminant .
The cmath module is used for complex maths, using this, we can find the square root of even if it is negative.
Code
Output 1
Output 2
In the above example, we are taking the quadratic equation from the user as input and then calling the QuadRoots() function to return the roots of the quadratic equation. Inside the function, we are calculating the value of the discriminant to determine the nature of the roots. We have then used the cmath.sqrt() function from cmath module to calculate the roots of the quadratic equation.
Conclusion
- A quadratic equation is of the form , where .
- We can solve a quadratic equation using the quadratic formula, which is , where is the discriminant and its value is .
- We can determine the nature of the roots by calculating the .
- If , the roots are real and different, if , the roots are real and same, and if , the roots are complex and a conjugative pair.
- A Python program to solve quadratic equation can be written using the sqrt() function in math and cmath module.
- The sqrt() function from math module can only find the square root of positive numbers.
- The cmath.sqrt() function from cmath module can find square root of any number.