Python Random Module
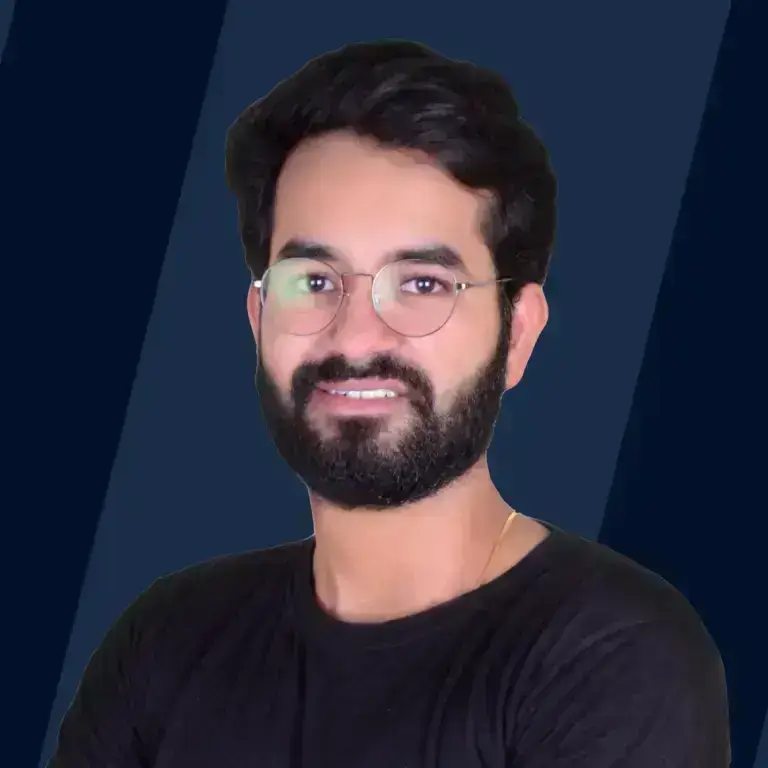
The Random module in python is a popular module in Python which is associated with generating random data. There are multiple other use cases of the Python Random Module. Python offers us a variety of random number generation modules.
Also, we do not need to install the Python Random Module, because it comes built-in and we can directly use it by importing the required module (random) at the beginning of our code. In this article, we are going to cover in-depth the Python Random Module.
Various Functions of Python Random Module
We have discussed some of the widely used functions of the Python Random Module. But, those are not the only functions that the Python Random Module supports. There are many other functions that are supported by the Python Random Module.
For example, a few are listed below:
- random sample() to choose multiple items from any sequence.
- random.seed() function to initialize the pseudo-random number generator.
Let us look at the below-listed table which consists of the functions of the Python Random Module with a summary of their purposes.
Function | Purpose |
---|---|
random() | It returns a number between 0 and 1 |
seed(x) | It sets the generator to a new sequence of x |
randint(a, b) | It returns an integer in the range of a and b |
randrange(a, b, c) | It returns an integer in the range of a to b, with increments of c steps |
uniform(a, b) | It returns a floating point number in the range of a to b |
shuffle(x) | It shuffles the values in some given list x |
choice(x) | It returns some random element from the list x |
sample(a, b) | It returns a sequence (list) of length "b" from any given to set a |
getstate() | It returns the internal state of a generator |
setstate(x) | It restores the internal state of any generator x |
getrandbits(x) | It returns a randomly generated "x" bits |
triangular(a, b, c) | It returns a real number from a to b with a distribution of c |
betavariate() | It returns a random floating point number with beta distribution |
gammavariate() | It returns a random floating point number with gamma distribution |
expovariate() | It return a random floating point number with exponential distribution |
lognormvariate() | It return a random floating point number with log-normal distribution |
gauss() | It returns a random floating point number with Gaussian distribution |
vonmisesvariate() | It returns a random floating point number with von Mises distribution or circular normal distribution |
normalvariate() | It returns a random floating point number with normal distribution |
weibullvariate() | It returns a random floating point number with Weibull distribution |
paretovariate() | It return a random floating point number with Pareto distribution |
Examples of Random Module in Python
Let us now start with some basic examples of the Python Random Module with their syntax.
1. Creating Random Integers with Python Random Module
Generating the random integers is one of the most popular use cases of the Python Random Module.
The randint function is primarily used for the generation of the random integers, within a specified range. It takes two arguments - a minimum value and a maximum value. It generates the random integers within that given range.
Let us look into its syntax below for a better understanding:
Syntax:
- First we need to import the random module.
- Then we will provide the start and end values, which must be integers
- It will return a random integer in the range [start, end] including the endpoints.
Having understood the syntax, let us now look into a code example to understand its usage.
Code 1:
Output:
Explanation: The randint() function in python generated a random number between the given positive range.
Generating random numbers between negative ranges:
Code 2:
Output:
Explanation: The randint() function in python generated a random number between the given negative range.
Generating random numbers between negative and positive ranges:
Code 3:
Output:
Explanation: The randint() function in python generated a random number between the given negative and positive range.
2. Creating Random Floats with Python Random Module
A Random float number can be generated between a given range or between the range 0 to 1. Please note that the random number generated is between or equal to the given range's endpoint(maximum value).
There are multiple ways to generate Random Floats numbers with Python Random Module. Here we will learn about the two most important ways:
- Using random.random()
- Using random.uniform(start, end)
Difference between the random.random() and random.uniform()
The very basic distinction between these functions is that, the random.random() generates random numbers between 0 to 1 (exclusive). And the random.uniform(start, end) generates a random number between the given start and end value (both inclusive). The range specified here can be floating point values or even integer. Also, both positive and negative ranges are allowed.
Using random.random()
The random.random() function is used to generate a random float number in a uniform way in the range [0.0, 1.0). Please note that the range is exclusive or semi-open. That means, the last number is not included in the random number generated.
Having understood that, let us now look into a code example to understand its usage.
Code 1:
Output:
Explanation: Here the random.random() function generated the floating random numbers in the range [0.0, 1.0), with every iteration of our loop.
Using random.uniform(start, stop) As already explained, the random.uniform() function is used to return a random floating point number within a given range in Python. The specified range can either be integer values or floating point values. That means the range can be from [5, 10] or [5.5, 10.5] (suppose).
Syntax:
The random.uniform() function returns a random floating-point number such that , that is, the range of the random.uniform() is inclusive.
In simple terms, random.uniform(5.5, 10.5) will generate any random float number greater than or equal to 5.5 and less than or equal to 10.5.
Code 1:
Output:
Explanation: In the above example, we have covered most of the use cases of the random uniform function to generate floating point random numbers in Python. We have tried to generate the numbers between positive, negative, floating point and integer ranges. Hence, you can conclude that passing any valid range is possible in the uniform function.
Exceptions in random.uniform() function
Both the arguments of the random.uniform() function are mandatory and if we skip any of them then we will encounter the TypeError: uniform() missing 1 required positional argument: 'b'
Code:
Output:
3. Selecting Random Elements with Python Random Module
Selecting random elements from a list or any iterable is another popular use case of the Python Random Module.
In Python, to select any random element from any list(or iterable), we can make use of the choice() function defined in the Random Module of Python.
To select a random element from a list in Python, we can use the choice() function defined in the random module. The choice() function takes a list as input and returns a random element from the list every time it is executed.
Let us look into its syntax below for a better understanding:
Syntax:
The choice function takes a sequence as its input and it returns any random element from the sequence, whenever it is called or executed. The sequence we pass as the argument is a mandatory parameter. The sequence can be anything like a list, tuple, etc.
Having understood the syntax, let us now look into a code example to understand its usage.
Code 1:
Output:
Explanation: In the above example, we have generated random elements from the given list. Please note, that you can have any iterable like the tuple apart from the list.
Exceptions in random.choice() function
The parameter sequence of the random.choice() function is mandatory and if we skip it then we will encounter the choice() missing 1 required positional argument: 'seq' error.
Code:
Output:
4. Shuffling list with Python Random Module
Shuffling a list basically means shuffling the order of the items in the list. When we shuffle a list, all the items are rearranged in some random order. For example, just like we shuffle a list of cards.
Shuffling a list can be done using the random.shuffle() function in the Python random module.
Let us look into its syntax below for a better understanding:
Syntax:
That means, shuffling a sequence(such as a list), using any specified random function.
Parameters: The random.shuffle() function takes two parameters. Let us discuss them below:
- sequence: It is the sequence (such as a list) that we want to shuffle. It is a mandatory parameter
- random: It can be used as an optional argument, and it returns a random float number between 0.1 and 1.0. How a sequence is shuffled is determined by this function. Python uses the random.random() function by default if it is not otherwise specified.
Important Note: This parameter (random) is deprecated since version 3.9, and will be removed in version 3.11
Return Value: random.shuffle() shuffles the given sequence in place and doesn’t return anything, or returns None. This function just changes the position of the items in any mutable sequence such as a list. Having understood the syntax, let us now look into a code example to understand its usage.
Code 1:
Output:
Explanation:
In the above example, you could see how using the random.shuffle() function we were able to shuffle the positions of the items in the list.
Exceptions in random.shuffle() Function
An important thing to note here in the random.shuffle() function is that it only takes mutable sequences as arguments. Because we cannot change the order or the content of immutable sequences (such as tuples). So, if we try to pass immutable sequences like tuples or strings to the random.shuffle()function then we will encounter an errorTypeError: 'tuple' object does not support item assignment
Code:
Output:
Explanation:
We passed the tuple as the argument and hence we encountered that error because we cannot support item assignment in a tuple.
Another execption is that the parameter sequence of the random.shuffle() function is mandatory and if we skip it then we will encounter the TypeError: shuffle() missing 1 required positional argument: 'x'' error.
Shuffle List in Python
The random.shuffle() method serves to reorder the elements within a sequence, typically a list, in a random manner. This operation alters the position of the elements directly within the sequence itself, thus modifying it in place.
Syntax:
Example:
Output:
Conclusion
- The Python Random Module is a built-in module which is used to produce random numbers.
- We need to import random before using the Python Random Module.
- We can generate random integers by using the randint() function.
- We can generate random floating point numbers by using random.uniform(start, end)
- To select any random element from any list(or iterable), choice() function can be used.
- Shuffling a list can be done using the random.shuffle() function in the Python random module.