Python Reload Module
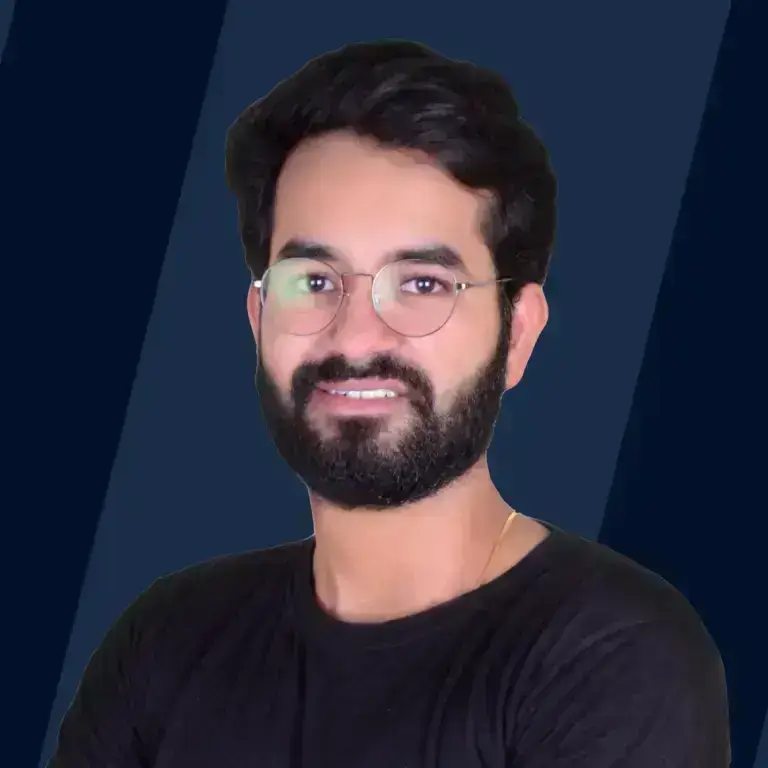
Overview
The Python reload module is used in reloading any module that has previously been imported into the program. The Python reload module is useful in situations like an interactive session where we are frequently running any test script because in such cases we always use the module's first version even if any changes have been made in the code. Thus we use the Python reload modules to make sure that the modules are being reloaded.
Python Reload Module
As discussed earlier, the Python reload module is used to reload a module that has previously been imported into the Program. The Python reload module helps in cases where we are trying to use a newer version of any module and the existing version of that module had already been edited using any external editor. The Python reload module helps us achieve this without making us leave the Python interpreter.
- The Python reload module returns a module object.
Execution of Python Reload Module
- The python module’s code is recompiled and the module-level code is re-executed, defining a new set of objects which are bound to names in the module’s dictionary by reusing the loader which originally loaded the module. However, the init function of the modules is not loaded again.
- The old objects are only reclaimed after their reference counts reach zero.
- The names in the module namespace is changed to new object if any.
- Other references of the old objects (like names external to the module) are not necessarily referred to the new objects and must be updated in each namespace where they occur if that is required.
Loading a Module in Python
In this section, we will learn how to load a module in Python. In order to load a module in Python, we need to use the import keyword followed by the module name.
note: It is advised to import the module at the top of the program.
Syntax:
- Here the module_name is the name of the module that we want to load.
Example
Code:
Output:
Explanation of the Example:
In the above example, we are loading the module random using import random. This will import the random module which is used to generate random numbers. Then we will loop five times to execute random.randint(0, 9) which will display a random number between 0 and 9. Thus the output is 7, 3, 2, 2, 7.
Reloading a Module in Python
In this section, we will learn how to reload a module in Python. In order to reload a module in Python we need to use the reload() method which takes the module that has to be reloaded as an argument.
The reload() method is used to reload a module. The argument passed to the reload() must be a module object which is successfully imported before.
Syntax:
- Here the module_name is the name of the module that we want to load.
Example
Code:
Output:
Explanation of the example:
In the above example, we are loading the module random using import random. This will import the random module used to generate random numbers. In this program we have also imported importlib which then will use the importlib.reload() method to reload the random library. Then we will loop five times to execute random.randint(0, 9) which will display a random number between 0 and 9. Thus the output is 6, 3, 2, 7, 5.
note: The module that we want to reload should have already been imported previously in the program. In case we do not import the module previously then the program will throw an error.
- For example*, suppose in the above code we do not import the random module, we will get the following output:
Code:
Output:
Example for Understanding
In this section, we will go through some examples to learn more about the Python reload module.
Reloading a Module in Python
In this example, we will use the Python reload module to reload the math module in Python.
Code:
Output:
Explanation of the example:
In the above example, we are importing the math module which is used to perform mathematical calculations in Python. We have also imported the importlib module and will use the importlib.reload(math) to reload the math module. We have set the radius (rad = 2) and obtained the value of pi (pie = math.pi). Thus the areaOfCircle = pie * rad * rad will display the area of the circle which is 12.566370614359172 units.
Suppose we have created a module exampleModule in our program that has a function show() that displays "Welcome!". The exampleModule.py will look like the following:
-
exampleModule.py
Code:
Now, we will create will create another file named example.py which will import the exampleModule.py as the following:
example.py
Code:
Output:
-
Loading the module:
Code:
Output:
Explanation of the above code:
In the above code, we have imported the exampleModule in our program. Now the repl will access the show() method in the exampleModule using exampleModule.show. Thus, when exampleModule.show() is called it displays "Welcome!".
What will happen if the print statement of the show() is changed?
Now, suppose we change the print statement of the show() method in exampleModule to "Hello World!". Now, what will happen if example.py is executed?
-
exampleModule.py
Code:
example.py
Code:
Output:
-
Reloading the module:
Code:
Output:
Explanation of the above code:
In the above code, we have already imported the exampleModule in our program and it was loaded before the change. thus, when exampleModule.show() is called it will still display "Welcome!" as it is not synced with the new changes in exampleModule.
Thus in order to tackle the above problem Python reload module is used.
-
exampleModule.py
Code:
example.py
Code:
Output:
-
Reloading the module:
Code:
Output:
Explanation of the above code:
In the above code, we have imported the reload module in our program using importlib. Now the im.reload(exampleModule) will reload the exampleModule thus when exampleModule.show() is called it will display "Hello world!".
FAQs
Q: What is Importlib in Python?
A: The importlib package is used to provide the import statement's implementation in our Python program, making it portable for other Python interpreters.
This also provides an implementation that is easier to comprehend than one implemented in a programming language other than Python.
Q: What is REPL in Python?
A: The Python REPL stands for Read, Evaluate, Print, and Loop. The Python REPL is used by developers all over the world for communicating with the Python Interpreter. Rather than running a Python file, in Python REPL one may just input commands to see the results getting displayed immediately.
Q: What is a Module in Python?
A: The Python module refers to an arbitrary object with named attributes that can be used for binding and references. The Python code for a module named aname normally resides in a file named aname.py, as covered in Module Loading. In Python, modules are objects (values) and are handled like other objects.
Q: Which Is the Minimum Version of Python Required to Use the Python Reload Module?
A: The Python reload module feature has been added in Python 3.3, thus we need Python 3.3 or above installed in our system to use the Python reload the module. It is not supported for Python 2.x versions.
Conclusion
- The Python reload module is used to reload any module in the Python program.
- The Python reload module returns a module object.
- It is advised to import the module at the top of the program.
- The module that we want to reload should have already been imported previously in the program.
- The Python reload module is useful in situations like an interactive session where we are frequently running any test script.
- The Python reload module helps us reload modules without making us leave the Python interpreter.