Set add() in Python
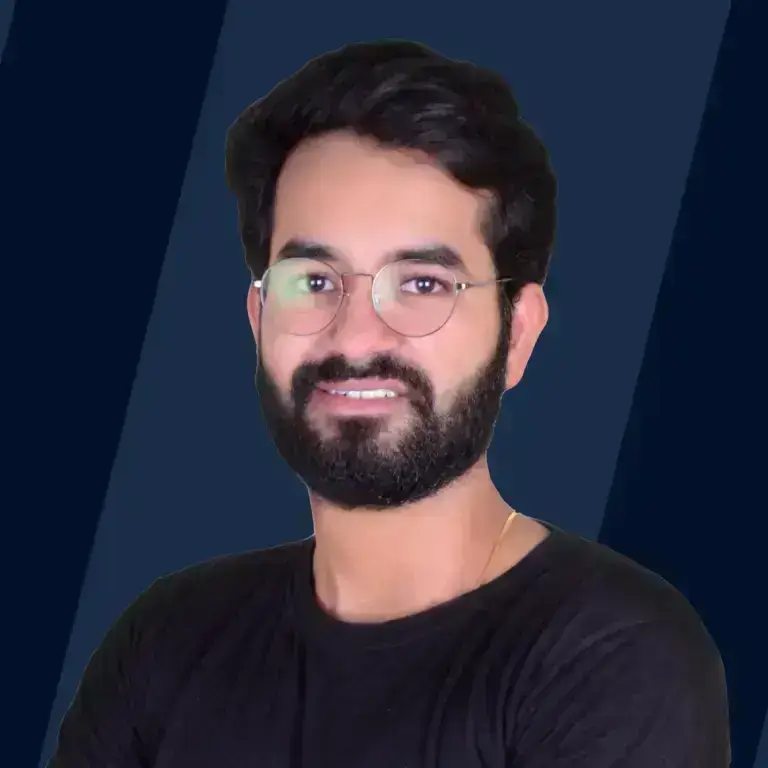
Overview
The set data type in Python provides an unordered collection with unique elements. We use the add() method to add an item to a set. Its syntax is pretty straightforward; we just need to indicate the element to be added as an argument, and Python does the rest of the work. Sets do not allow duplicate values; therefore, if you try to add an element already in the set, it will not change the set, and hence, the element won't be added.
Syntax of set.add() in Python
In Python, the set.add() method adds a single element to a set. Its syntax is straightforward:
Here, element is the item you want to add to the set. For example:
Parameters of set.add() in Python
The set.add() function in Python is helpful in handling sets, which are collections of unique items. You may use this approach to introduce a single item into a set. It just requires one argument: the element to be added.
So in, set.add(element).
- set: Your target specifies where the element should be inserted.
- element: This is an object you want to include in the collection.
For instance, if you have a set named my_set and wish to add 42 to it, you would use my_set.add(42). This guarantees that your collection is clear of duplicates and retains its originality.
Remember that set.add() does not return the updated set but changes the same set. So, utilize it when necessary to include a single, distinct element in your set collection.
Return Value of set.add() in Python
The set.add() has a simple rule: if the provided element does not already exist in the set, it adds it and returns None. If the element is already in the set, the method does not change the set and returns None.
Here's a practical example:
So, when you use set.add(), remember it returns None rather than the changed set. It's a small but important distinction when dealing with Python sets.
Exceptions of set.add() in Python
In Python, adding elements to a set is typically a simple operation when utilizing the set.add() function. However, being aware of any potential deviations during this process is critical.
-
A TypeError is a frequent error when adding an unhashable type to the set. Sets in Python need hashable items, such as integers, texts, or tuples. For example, you'll get this exception if you try to add a list or another set.
-
Another error is a MemoryError, which occurs when your system runs out of memory while attempting to add elements to a set. This is uncommon but worth considering, mainly when working with many components.
How does the set.add() in Python Work?
To add an element to a set in Python, use the set.add() function. A set is an unsorted collection of distinct components. Python checks if the element is already in the set when you execute set.add(element). If it isn't, Python adds it to the set; otherwise, it does nothing to ensure that it contains no duplicate entries. This technique is very beneficial for keeping a collection of unique values while avoiding duplication.
Examples
Example - 1: Adding Elements to an Empty Set
Output:
In this example, we create an empty set, my_set, and use add() to insert elements.
Example - 2: Avoiding Duplicates
Output:
The set.add() method automatically removes duplicates.
Example - 3: Adding Strings to a Set
Output:
Use set.add() with non-integer values.
In conclusion, the set.add() method is a powerful tool for managing unique elements within a set. It assists you in keeping your collection clear of duplicates, whether you're working with numbers or strings.
Conclusion
- The set.add() is a quick and effective technique to insert a single element into a Python set while avoiding duplication.
- For example, if you have a set named my_set and want to add the element apple, the syntax would be my_set.add("apple"). This straightforward strategy allows you to keep your set's pieces unique and free of duplication.
- Sets do not allow duplicate values; therefore, if you try to add an element already in the set, it will not change.
- Sets are mutable in Python, but set.add() allows you to edit sets without affecting their identity, making it handy when immutability is necessary.
- This approach is suitable for preserving collections of unique values, such as deleting duplicate items from a list or assuring dataset uniqueness.
- Unlike lists or dictionaries, Sets do not throw errors when adding an existing element, making set.add() a safe option for dealing with probable duplication.
- The set.add() function has a constant time complexity of , making it a good choice for huge sets.