Python setattr() Function
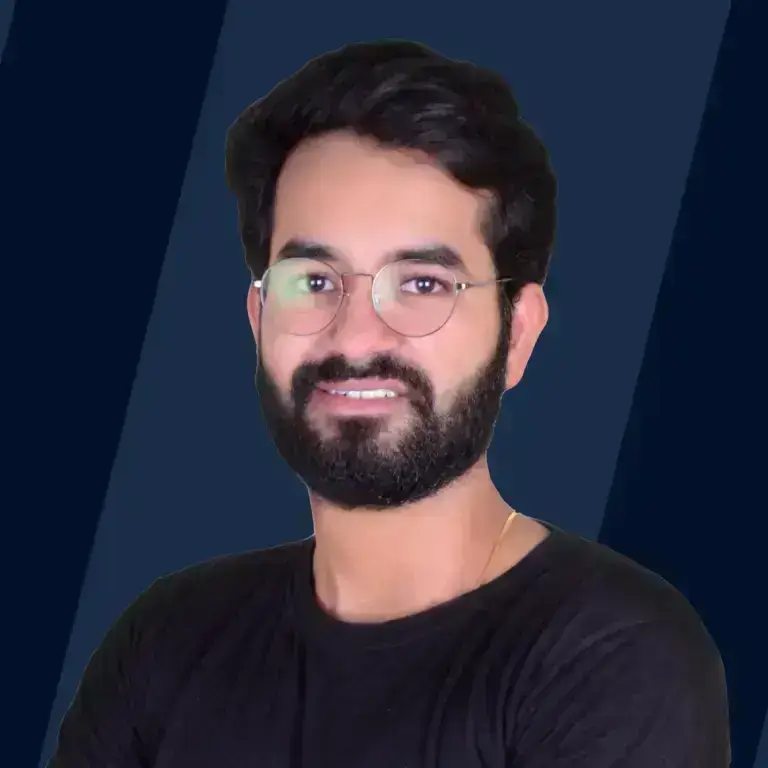
Overview
Setting a value for an object's attribute in Python is done using the setattr() function. It requires three arguments—an object, a string, and an undefined value, and it doesn't return anything. It is useful when we wish to give an object a new attribute and set a value for it. The syntax for this Python setattr() function is given below.
Syntax of Python setattr() Function
Parameters of Python setattr() Function
1. Object- It's an object whose attribute is to be changed. 2. Name- Name of an attribute that is to be changed. 3. Value- Value which is to be assigned to the attribute.
Return Value of Python setattr() Function
The calling function receives None in return.
Exceptions of Python setattr() Function
Using a property function or the property decorator in the object, we can define a read-only attribute.
In such a situation, we would encounter an AttributeError: Can't Set Attribute Exception if we attempted to set the attribute value by using the setattr() function.
Let's have a look at the code given below.
Output
So as you can see in the above code the model is the read-only type of attribute in the class Car and we are trying to set the value for it using the setattr() function which is not possible with the setattr() function so it is raising an error that is "AttributeError: can't set attribute".
Working of Python setattr() Function
An example is given below for understanding the working of setattr() function.
Output
In an example that is given above, an object car is there having an attribute brand having a predefined value as "Rolls-Royce". Now we have changed that value from "RollsRoyce" to "McLaren", so we will use the setattr() function in python and will pass the object name(c here in the example), attribute name(brand here in the example), value(McLaren here in the example).
Uses of Python setattr() Function
Some uses of the setattr() function are given below.
1. setattr() function is used for changing the value that is assigned to the specified attribute of an object. We have seen that in the example explained above.
2. If an attribute that is passed as a parameter to the setattr() function is not there in the object then that setattr() function creates that new attribute inside the object and assigns that specified value to it. An example is given below for a better understanding.
Output
As we can see in an example given above that the attribute is being passed to the setattr() i.e Model is not present in the Car object so this setattr() function will create that new attribute inside the car object and also assign that value Z4 to that newly created attribute Model.
Examples of Python setattr() Function
1. Using the setattr() function with dictionaries in Python
Here, we're using the setattr() function to dynamically create the class's attributes by passing the dictionary class init() method a dictionary containing some keys and values.
Output
2. Displaying Properties of setattr() Function
- Any object attribute can be given None using the setattr() method.
- A new object attribute can be initialized with setattr().
Let's understand the above 2 properties with help of an example given below:
Output
As you can see from the above example the how setattr() function can be used to set the attribute value to None and a new attribute can also be initialized by using setattr(). So by this, we have understood both the mentioned properties of the setattr() function.
Related Functions in python
Conclusion
After going through this article we have got to know that:
- Python setattr() function is a useful one to alter the values of the predefined class attributes.
- New attribute can also be added up later after the creation of an object using this Python setattr() function.
- Dictionary keys can also be used as a name of attributes in the object.