What is Python Singleton Method?
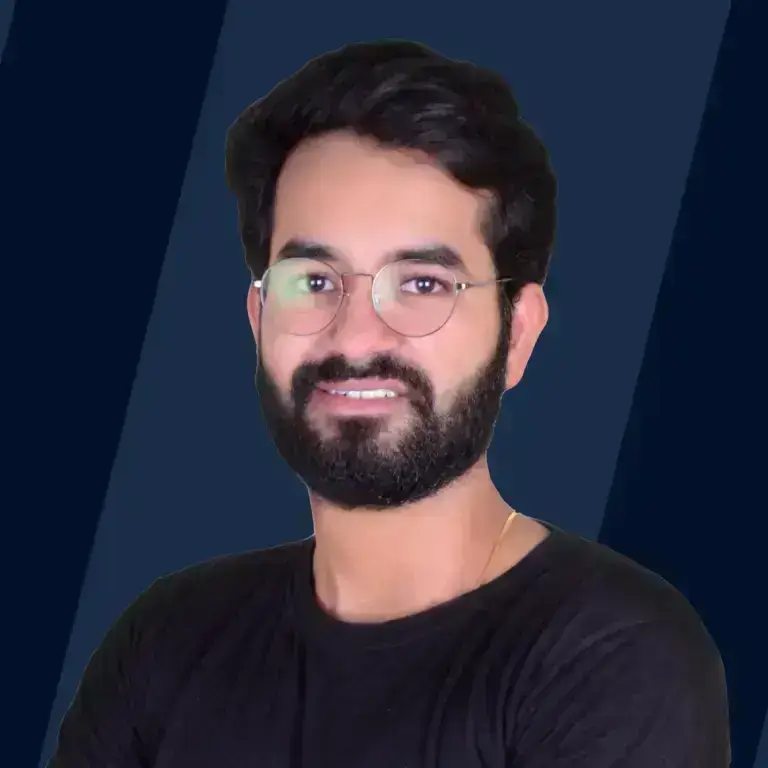
What is Python Singleton Method?
Before understanding the singleton method in Python, let's first understand what singleton means. A singleton is a set consisting of exactly one element in it. Or else, a tuple of length 1 can be referred to as a singleton.
Definition Singleton methods are those whose objects cannot be instantiated more than once in a program. It is a creational design pattern. The Singleton method ensures that only a single object of its type exists. It allows a single (global) point of access to the newly created instance of a class. In other words, in the singleton method, we create an object or instance of a class only once. We use the same object to get multiple instances.
Example We can take the example of database connectivity to understand the singleton method. In a particular program, if every object makes its own database connection to access the database, then the program will be slow and will be very heavy. So instead of making different connections, we make a single connection, and every object uses the same connection to access the database. It is how the singleton method works.
Borg/Monostate Singleton Design Pattern
In the Borg / Monostate singleton design pattern, we create multiple instances of the class instead of creating a single instance. All these multiple instances share the same state. We do not focus on sharing instances in the Borg / Monostate singleton design pattern. Instead, we focus on sharing state. The following example will help you understand the Borg / Monostate singleton design pattern.
Code
Output
Explanation In the above example, we have shared data between all the instances in the program. We have created multiple objects of the same class, but still, every class shares common data. Changing the data by object 1 will affect the data of object two and object 3. Similarly, if object 2 or object 3 tries to change the data, then it will affect globally because the data is shared between all three instances.
Applicability of Python Singleton Method
The applicability of the Python Single Design Pattern is as follows:
- In huge projects, we often need to maintain the global variables. We must use a singleton design pattern to have total control over the global variables.
- Singleton patterns frequently work in conjunction with the Factory design pattern to tackle the most frequent issues, including caching, logging, configuration setup, and thread pools.
- When only one instance of a class is required to control an action's execution, then singleton design patterns are highly utilized.
How to Create Singleton in Python?
In this section, we will deal with the classical way of designing the singleton design pattern. In the classical method, we use the static method to create the getInstance() method. This getInstance() method is created to return the shared resources. We also make use of a virtual private constructor. This constructor will help in raising the exceptions. However, it is not mandatory to create a singleton method. We use it for better coding practice.
Code
Output
Explanation In the above example, we have created a single instance of the singleton_design class. Then later, we pick the instance of the singleton_design from the already created object. We pick the instances using the getInstance() method. In the getInstance() method, we check if the object is already created. If the object is not created, then we return the new object, or else we call the constructor of the singleton_design class (Further, the constructor will raise an exception).
Raising Exception
What if we create multiple instances of the singleton_design class? Let us see the following example to see what happens if we create two or more objects of the singleton_class.
Code
Output
Explanation In the above example, we are trying to make two instances of the singleton_design class. When we try to make the second instance of the singleton_design class, it throws an exception. This is because singleton class cannot be instantiated more than once.
Advantages of Using the Python Singleton Method
- Initializations: The object of the singleton method is initialized when requested for the first time.
- Access to the object: The object created by the singleton method will have global access. Therefore it is called a shared instance.
- Count of instances: The singleton methods cannot have more than one instance. Only a single object of the same type can be created.
Disadvantages of Using Python Singleton Method
- Multithread Environment: Using the singleton method in a multithreaded environment is challenging because we have to watch out for several instances of the singleton object being created by the multithread.
- Single responsibility principle: The Singleton approach violates the single responsibility principle because it addresses two issues at once.
- Unit testing process: Unit testing is quite difficult due to the introduction of the global state to the program.
Learn More
The above article was part of the advanced Python programming. You can refer to more such interesting articles listed below.
Conclusion
- The Singleton design pattern is widely used in software development to ensure that a class has only one instance throughout the application.
- Python provides several ways to implement the Singleton pattern, including the Borg pattern, metaclasses, and decorators.
- The Borg pattern uses a shared state to ensure that all instances of a class share the same state and behave like a singleton.
- Metaclasses are used to create classes, and they can be used to enforce a singleton pattern by modifying the creation of a class.
- Decorators are functions that modify the behavior of other functions or classes, and they can be used to create a singleton by modifying the behavior of a class's constructor.
- Each approach to implementing the Singleton pattern in Python has its own advantages and disadvantages, and the choice of approach may depend on the specific use case.
- While the Singleton pattern can be useful in certain scenarios, it may also introduce some drawbacks, such as making the code harder to test and maintain.
- Understanding the Singleton pattern and its implementation in Python can be a valuable skill for Python developers.