Python Program to Convert Str to Bool
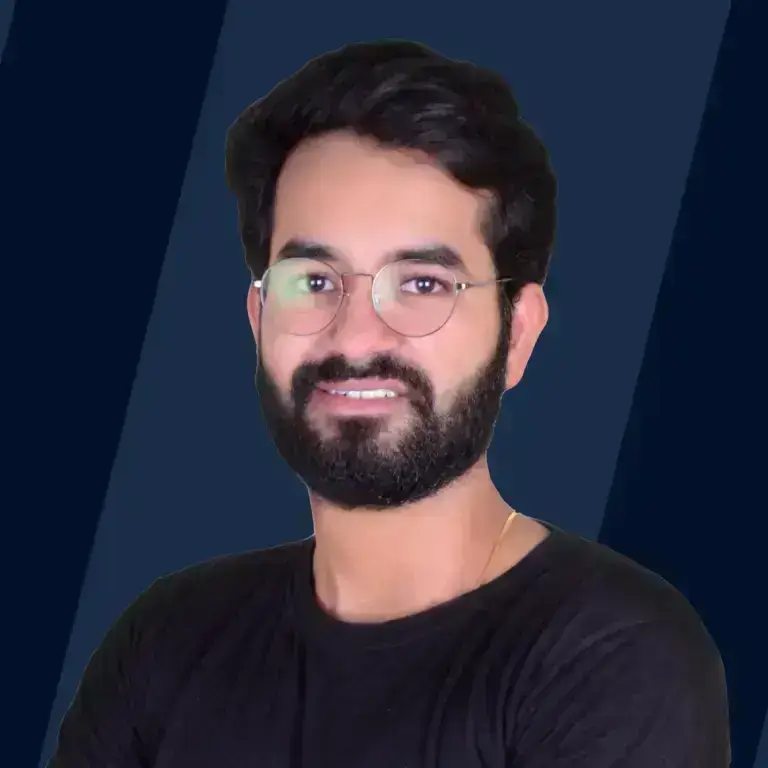
Overview
Boolean is one of Python's built-in data types, which can only return 2 possible values: true or false, which is why the bool data type is best suited and appropriate for various problem statements. It is a necessary data type often used in Python.
This article shows how to convert Python str to bool.
Converting String Values to Boolean in Python
There are a few python methods, namely bool(), eval(), the concept of List Comprehension as well as the Lamba+Map() method in Python.
Method 1: Using bool()
Python's bool() function converts any given value into the desired Boolean value. It employs the basic truth-testing process, which states that if the condition passed as an attribute is true, then it returns the output as True; otherwise, if the condition passed as an attribute is false or no attribute is passed, the value returned as output is False.
Syntax
bool(object)
Parameters
object : Any object, like a String, List, Number, etc.
The bool() function converts String to Boolean in Python.
Output:
When the argument passed in the above-given program is not empty, then this method returns the output value as True; otherwise, it returns a False value. Let's see one more example to understand the concept of bool() function in a better way.
Output:
Since no argument is passed in the method, the output is returned as False.
Method 2: Using eval()
The eval() method in Python Programming language can be used to parse the values or arguments passed as a parameter and evaluate it as an expression in Python.
If the string's value is either "True" or "False," the eval() method can be used. If the string meets these criteria and is legal, it will be executed.
Syntax
eval(expression, globals, locals)
Parameters
expression : String that will be evaluated
globals(Optional) : A dictionary containing global parameters
locals(Optional) : A dictionary containing local parameters
In Python, the eval() method is used to convert a string to a Boolean value. Let's write a small Python code to understand the concept of eval() function in Python straightforwardly.
Output:
Output:
Method 3: Using List Comprehension
List comprehension is defined as a comparatively quick and efficient method for creating lists based on given elements of an existing list. This method only checks one of the True or False values, while the other value is assumed to be the inverse of the checked value.
The following code converts Python str to bool value using list comprehension in Python. When this method is used, we only check for the true value, and the remaining values are automatically converted to False booleans.
Output:
The False values are inspected in this code, while the True values are assumed to be True.
Method 4: Using Lambda + map()
The map() method in Python is used to apply a specific method to all components in any provided iterable. As a consequence, it reverts the iterator itself.
A lambda method is defined as a convenient anonymous method that can accept any number of parameters while containing only one expression.
Output:
The basic idea behind this approach and list comprehension is the same. The primary difference is that we utilized the map() method in conjunction with the lambda method to generate a list instead of list comprehension.
Which is the Most Useful Method?
In Python, the simplest method is to use the str(boolean) method and then pass in the Boolean value to transform it into a string value, which is very simple. Boolean True is converted to string "True", and Boolean False is converted to the string value "False".
The str() method is considered to be one of the easiest and simplest methods to convert any given Python str to bool.
FAQs
Q: How do programmers encode a boolean type in Python?
A: True and False can be converted to strings 'True' and 'False' using the str() method. Because the non-empty strings are assumed True by default, converting False to strings with str() and afterward back to bool type with bool() will result in True.
Q: How do programmers change the encoding type in Python?
A: Easily utilize the type name as a method to convert between different kinds. Numerous built-in functions convert the data from one form to another. All such methods return a new object that represents the value that was transformed.
Q: How does one go from string false to false in boolean type?
A: The easiest way to accomplish this is to make comparisons between the string value to "true" - if such string is (purely) equal to "true," then the result will be boolean true. To get a similar result, you can also use the ternary operator in conjunction with the loose equality operator.
Conclusion
- True or false are the only two possible values for the boolean data type.
- The map() method extends the logic of the lambda function's values. We can use the map and lambda functions to convert any given string value to its boolean value.
- As discussed above, Python eval() parses the expression argument and evaluates it as a Python expression before running the program's Python expression(code).