Python ValueError
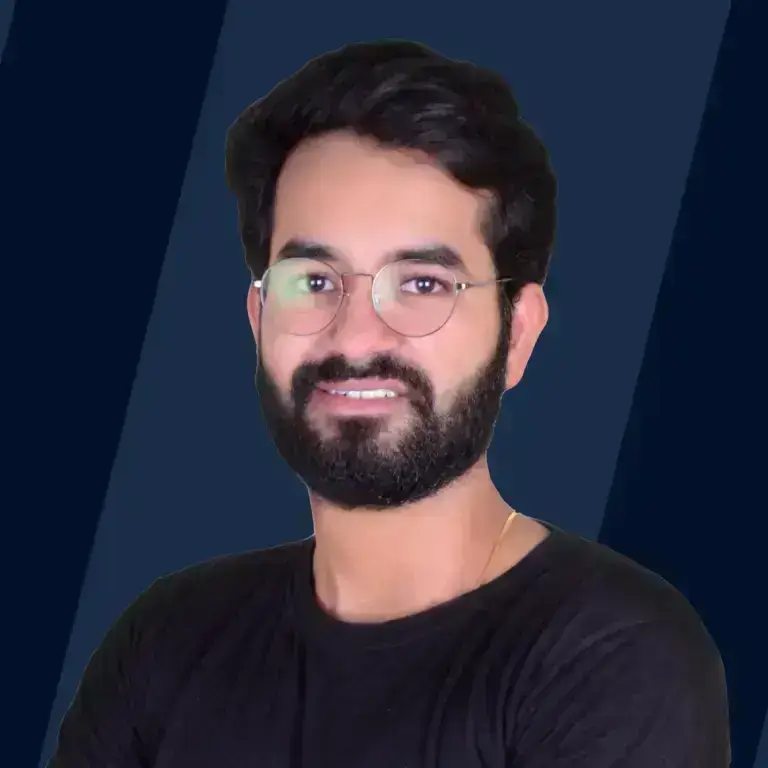
Overview
As you already know, an exception happens when an error occurs during the execution of a script. Python's try-except block is used to manage exceptions. Python has many built-in exceptions to handle common failures, such as IndexError, KeyError, NameError, TypeError, Python ValueError, and so on. In Python, a ValueError is a rather straightforward concept. A Python ValueError is thrown when you assign the incorrect value to an object. That means Python ValueError is raised when a function receives an argument of the correct type but an inappropriate value.
What is Python ValueError?
In Python, a ValueError occurs when a correct argument type but an incorrect value is supplied to a function. This type of inaccuracy is most common in mathematical operations. When the ValueError Python arises and how to handle it in Python is demonstrated in this article.
Python won't accept your code when you have the same type of object but the incorrect value. Let’s understand this by taking a real-life example. Suppose that you are trying to place a 7-inch phone into a 6-inch phone box. Did you see the problem? The phone will not fix in the box. That is a Python ValueError in a nutshell.
Make sure you don’t mix up a Python ValueError and TypeError. A TypeError, as opposed to a ValueError, is raised when an operation is performed that uses an incorrect or unsupported object type. For example, consider our previous example, if you are trying to fit a TV in a phone box, then Python is smart enough to know that these two types do not match.
Let’s consider a simple example to understand the Python ValueError.
Here is the output of the program.
As shown in the above code snippet, you will get a python ValueError with the mathematical operations, as you can see there is no such thing as the square root of a negative number, therefore the python raises a ValueError.
How Does Python ValueError Work?
As discussed above, Value Error in python occurs when a correct argument type but an incorrect value is supplied to a function. There are many reasons you might see the Python ValueError pop-ups and these are mentioned below:
- Trying to “unpack” more values than you have
- When you attempt to operate on a value that does not exist.
- The value is impossible (For example, finding the root of a negative number will result in a ValueError exception)
Steps to Avoid Python ValueError
There are various steps to avoid the Python ValueError. You can handle the ValueError using the try and except block. When the errors arise from an invalid output, in that case, the try and except block is very much useful to handle the Python ValueError that you’ll study in the next section.
You can also use the raise keyword to avoid the ValueError.
Handling Python ValueError
Let’s see how you can handle the Python ValueError using the try and except block in python.
In the below example, the main part of the code is in between the try and except block. The user is asked to enter the present age. If the ValueError has occurred for the input value, then the except block will be executed.
Here is the output of the program with different types of input.
Value Error Python Examples
Let’s take some more examples to understand how you can handle the Python ValueError.
Example:
In the below example, a classic example of obtaining a square root is discussed. We have used the try and except block to handle the error.
Here is the output of the program with different types of input.
Example:
Now, let's take another example in which the Python ValueError is handled without the try-except block and by using the raise keyword inside the function.
Here is the output of the program with different types of input.
Conclusion
- A Python ValueError is thrown when you assign the incorrect value to an object.
- In Python, a ValueError occurs when a correct argument type but an incorrect value is supplied to a function. This type of inaccuracy is most common in mathematical operations.
- A TypeError, as opposed to a ValueError, is raised when an operation is performed that is using an incorrect or unsupported object type.
- You can handle the ValueError using the try and except block.