Python Program to Write List to File
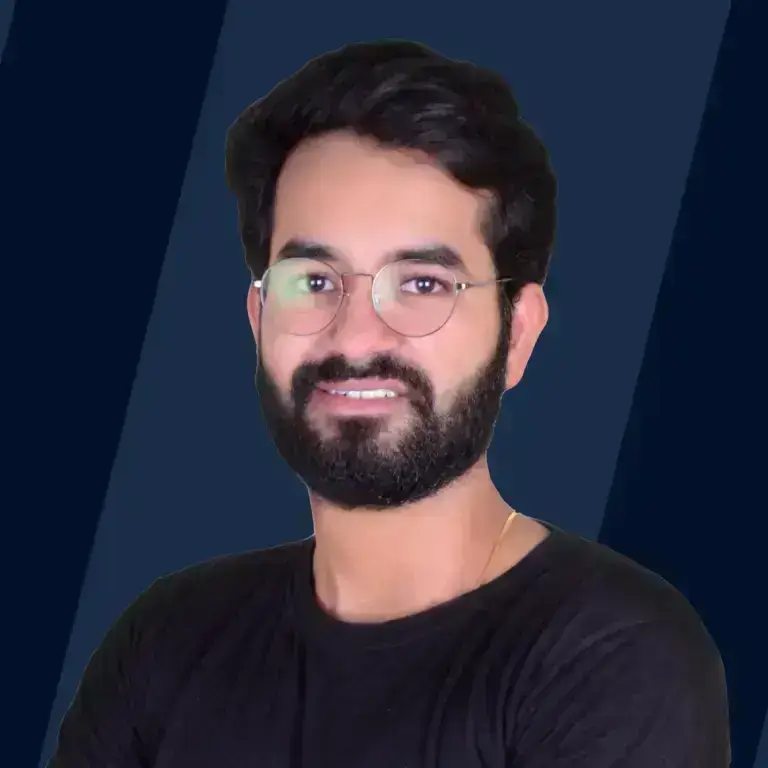
Overview
The List in Python is a built-in data structure that stores heterogeneous or homogeneous data in a sequential form. Elements in a list may be unique or duplicates and are identified by a unique position called an index. Python writes list to file and saves the data contained in the list to a text file.
How to Write List to File in Python
The write() or writelines() method helps Python write lists to files. A list in Python can be written in a file in various ways. The basic steps include:
- Open a text file in write mode
- Write current items from the list into the text file
- Close the file after completing the write operation
Method 1: Using write()
The list is iterated using a loop, and during every iteration, the write() method writes an item from the list to the file along with a newline character.
Steps
- Open a .txt file function in w mode (here w signifies write). The open() function shows the file path.
- Next, create a list of items. Using a for loop to iterate through all the items in the list.
- The write() function adds the list of items to the text file.
- Close the file using the close() function.
Code
Output
:::
Method 2: Using writelines()
The writelines() takes a list as its argument and writes all the elements of the list to a file. In the text file, the list elements are appended one after another without any space or newline characters.
Steps
- Open a .txt file function in w mode (here w signifies write). The open() function shows the file path.
- Create a list of items.
- The writelines() function takes the list of items as its parameter and writes them in the text file.
- Close the file using the close() function.
Code
Output
:::
Method 3: Using String Join Along with "with open" syntax
The with open syntax automatically closes the file after executing all statements inside it. Thus, the close() function does not need to be called explicitly.
Steps
- Create a list of items.
- Open a .txt file function in w mode (here w signifies write). The open() function shows the file path.
- The write function in this block adds the list of items to the text file.
Code
Output
Which is the Best Method to Write a List to File in Python?
The simplest solution for Python to write the list to a file is to use a file.write() method that writes all the items from the list to a file. The open() method opens the file in w mode. The list is looped through and all the items are written one by one.
Don't miss the opportunity to become a certified Python specialist. Enroll in Scaler Topics Python certification course and level up your career.
FAQs
Q: What does access mode 'w' mean?
A: The w mode refers to writing. It creates a new file if a file with the specified name is absent, else overwrites the existing file.
Q: How many arguments does the open() function take?
A: The open() function takes two arguments the filename along with its complete path, and the access mode.
Q: How many arguments does the close() function take?
A: The close() function doesn't take any argument.
Q: Why is using the with open() method not safe?
A: In the case of with open(), if some exception occurs while opening the file, then the code exits without closing the file.
Similar Python Programs
Conclusion
- Creating, reading, opening, writing, and closing files using Python comes under File Handling.
- Python write list to file can be implemented using multiple ways.
- write() method inserts a string to a single line in a text file.
- writelines() is used for inserting multiple strings from a list of strings to the text file simultaneously.
- No close() statement is required as with open syntax automatically closes the file after executing all statements inside it.