Random Access File in Java
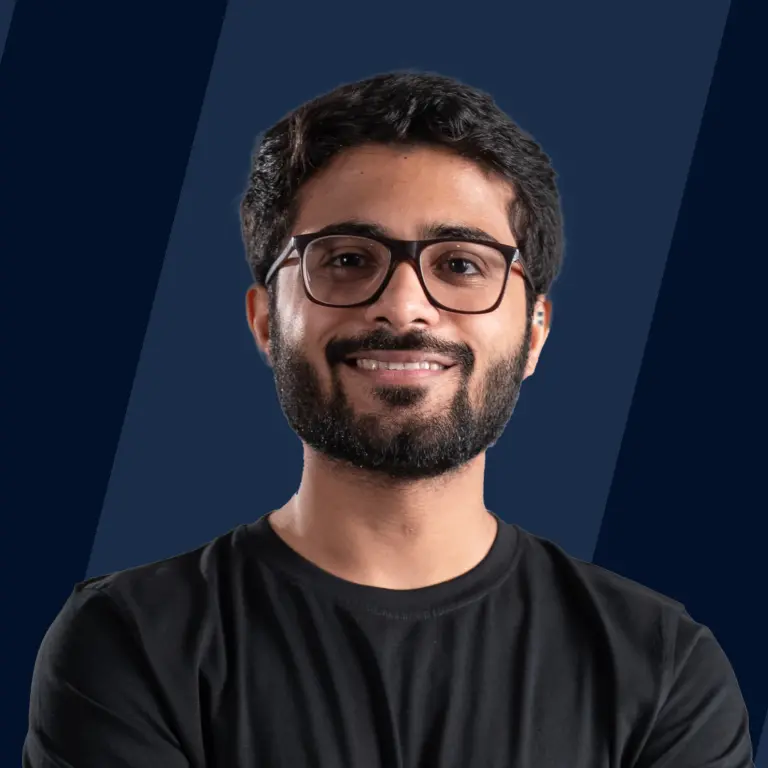
Overview
RandomAccessFile is a Java class that allows the reading and writting of data to any location in the file respectively. It is used to read and write data simultaneously i.e. we can read the file, write to a file, and move the file pointer to another location in the same program.
What is Random Access File in Java ?
RandomAccessFile in java is a class that lets the user read and write to a file at the same time. It extends the Object class and implements DataInput and DataOutput interfaces. These interfaces facilitate the conversion of primitive type data to a sequence of bytes and bytes to specified type data. It is used to read and write data to a file simultaneously. The file acts as a large array of bytes stored in the file system.
Methods of this class usually throw EOFException i.e. End of File Exception if the end of the file is reached before the desired number of bytes are read. It is a type of IOException.
IOException is thrown if any error occurs other than EOFException like if the byte cannot be read or written.
RandomAccessFile Class Declaration
Class Declaration of Random access file in java is given below :
DataOutput and DataInput interfaces provide the functionality to convert data from any of the Java primitive data types to a series of bytes, writing these bytes to a binary stream and vice versa.
Closeable and AutoCloseable interfaces are used to release the resources at the end of the execution of the program.
File Pointer in RandomAccessFile Class
A file behaves like a large array of bytes, thus we use a cursor or index for the array called the file pointer. Data is read byte by byte starting at the file pointer and the write operation is performed starting at the file pointer too. File pointer can be moved or retrieved using seek() and getFilePointer() methods.
Constructors of RandomAccessFile Class
The RandomAccessFile class in Java has the following two constructors :
- RandomAccessFile(File fileObj, String mode) :
It creates a random access file stream to read, write, or execute the specified File Object. - RandomAccessFile(String fileName, String mode) :
It creates a random access file stream to read, write, or execute the file with the specified name.
Methods of RandomAccessFile in Java
Random access file has multiple methods that make the access of file easier. Let us look at the important methods of the class in detail.
1. seek(long pos) : java.io.RandomAccessFile.seek(long pos)
It sets the file pointer to the specified location. It starts measuring from the beginning of the file till the pos bytes in the file. The file pointer points to the location where the next read or write should occur.
Syntax :
Parameters :
It takes only one integer value as a parameter that describes the number of bytes to place the file pointer.
Return value :
It doesn't return any value.
2. getFilePointer(): java.io.RandomAccessFile.getFilePointer()
It returns the current offset or position of the File Pointer in the form of bytes from the beginning of the file.
Syntax :
Parameters :
It doesn't take any parameters.
Return value :
It returns a long value denoting the number of bytes, after which the file pointer is located.
3. length(): java.io.RandomAccessFile.length()
It returns the size of the file in the form of the number of bytes occupied by it.
Syntax :
Parameters :
It doesn't take any parameters.
Return value :
It returns a long value that denotes the number of bytes in the file.
4. read() : java.io.RandomAccessFile.read()
It reads the 1 byte of data from the file and returns it as an integer in the range 0-255.
Syntax :
Parameters :
It doesn't take any arguments.
Return type :
It returns an integer value such that if the file is not empty it reads one-byte data and returns it in the form of an integer and -1 if the end of the file is reached.
5. read(byte[] b) java.io.RandomAccessFile.read(byte[] b)
It reads n bytes from the file where n is the length of b i.e. .
Syntax :
Parameters :
It takes an array of bytes as a parameter.
Return type :
It returns an integer value such that if the file is not empty it returns a byte of data read up to n and it returns -1 if the end of the file is reached.
Example :
Let us take an example of the read method where we will read the content of the file in java.
file.txt :
myFile.java :
Output :
In the example above, we are creating an object of the RandomAcessFile class. It takes two parameters the file along with its location and the mode in which we wish to open the file. The mode can read, write or execute. We are moving the pointer 4 bytes ahead using the seek() method. We read 12 bytes of data from the file pointer into bytes and print it in the output by converting it into the string.
6. read((byte[] b, int offset, int len) : java.io.RandomAccessFile.read((byte[] b, int offset, int len)
reads bytes in buffer i.e. b from offset position to offset + len from the file in form of bytes.
Syntax :
Parameters :
- b is the buffer in which data is read.
- offset is the position from which we start adding bytes to the buffer.
- len is the number of bytes to be read inside the buffer starting from the offset.
Return Value :
It also returns an integer value that is equal to the number of bytes read from the file i.e. len.
For example :
If b is of length 14, , and . Then it will read 8 bytes from the beginning of the file in the buffer starting at the index 6-1 i.e. 5 up to index 5+8 i.e. 13 .
Example :
Let us see the same example as above using the additional two parameters offset and length.
Output :
In the example above first 8 indexes are left empty in the bytes array also called buffer. After this 3 bytes are written beginning from the file pointer to the array.
7. readFully(byte[] b) : java.io.RandomAccessFile.readFully(byte[] b)
It reads n bytes from the file into the buffer starting from the file pointer where n is the length of b.
Syntax :
Parameters :
public int read(byte[] b)
Return Value :
It returns the integer value equal to the length of b.
8. readFully(byte[] b, int offset, int len) : java.io.RandomAccessFile.readFully(byte[] b, int offset, int len)
It reads the len number of bytes initializing from an offset position.
Syntax :
Parameters :
It takes an array of bytes b i.e. buffer, integer offset to start from, and an integer len.
Return Value :
It returns integer value len i.e. number of bytes read.
Example :
Let us see an example of the readFully method with the same file.txt file. We will read all the contents of the file and print it.
Output :
It reads all the bytes of the file.txt and stores it inside the buffer array. raf.length() method returns the length of the file in bytes. The rest of the bytes of the array is empty.
9. close() : java.io.RandomAccessFile.close()
It closes the file stream of random access files and releases all the system resources associated with the stream.
Syntax :
Parameters :
It doesn't take any parameters.
Return value :
It doesn't return any value.
10. write(byte[] b) : java.io.RandomAccessFile.write(byte[] b)
It writes n bytes from the array of bytes to the file starting from the file pointer.
Syntax :
Parameters :
It takes a byte array as a parameter.
Return value :
It doesn't return any value.
Example :
Let us see an example to read data from one file and write that data to another file using the java program's read-and-write methods using a byte array.
file.txt :
tempFile.txt :
myFile.java :
Output :
In the above example, we take two files file1 and file2 both have content in them initially. We read data from file1 and write that data to file2. We use byte arrays to read the data of file1. This same array is used to write data in file2. To test the data in file2 we are using another array file2array to read the data in file2.
Note :
The file1 is opened in reading mode whereas file2 is opened in reading write mode as we are updating file2.
11. readLine(): java.io.RandomAccessFile.readLine()
These methods read the text of the next line from the file.
Syntax :
Parameters :
It doesn't take any parameters.
Return value :
It returns a string value.
RandomAccessFile Additional Methods in Java
1. readByte() : java.io.RandomAccessFile.readByte()
It reads an eight-bit value from the file starting from the File pointer.
Syntax :
Parameters :
It doesn't take any parameters.
Return Value :
It returns a signed eight-bit value.
2. readChar() : java.io.RandomAccessFile.readChar()
It reads a character from the file starting from the File pointer.
Syntax :
Parameters :
It doesn't take any parameters.
Return Value :
It returns a character value read from the file.
3. readBoolean() : java.io.RandomAccessFile.readBoolean()
It reads a boolean value from the file.
Syntax :
Parameters :
It doesn't take any parameters.
Return Value :
It returns a boolean value.
4. readDouble() : java.io.RandomAccessFile.readDouble()
It starts reading from the file pointer and returns a double value from the file.
Syntax :
Parameters :
It doesn't take any parameters.
Return Value :
readDouble() method returns a double value.
5. readFloat() : java.io.RandomAccessFile.readFloat()
readFloat() returns a float value starting from the file pointer.
Syntax :
Parameters :
It doesn't take any parameters.
Return Value :
It returns a float value from the file.
6. readLong() : java.io.RandomAccessFile.readLong()
This method reads a long value starting from the file pointer.
Syntax :
Parameters :
It doesn't take any parameters.
Return Value :
It returns a long value from the file.
7. readInt() : java.io.RandomAccessFile.readInt()
It returns an integer value starting from the file pointer.
Syntax :
Parameters :
It doesn't take any parameters.
Return Value :
It returns an integer value.
8. writeInt(int value) : java.io.RandomAccessFile.writeINt(int value)
This method writes an integer value to the file. It starts writing from the current position of the file pointer.
Syntax :
Parameters :
It takes an integer value as a parameter to be written in the file.
Return value :
It doesn't return any value.
9. writeFloat(float fl) : java.io.RandomAccessFile.write(float fl)
It writes a float value to the file where the file pointer points.
Syntax :
Parameters :
It takes a float value as a parameter.
Return value :
It doesn't return any value.
10. writeDouble(double d) : java.io.RandomAccessFile.write(double d)
It writes a double value to the file.
Syntax :
Parameters :
It takes a double value as a parameter to be written in the file where the file pointer points.
Return value :
It doesn't return any value.
RandomAccessFile Example Programs
Example : 1 - Read Write Operations
To experience the various read and write methods of the RandomAccessFile let us see this example that reads as well as writes different types of data to the file.
As we intend to write to a file, we will open the file in rw mode i.e. read-write mode.
Output :
In the example, we write and read various types of data like int, double, character, boolean, and String. The seek() method is used to change the position of the file pointer. writeBytes() method takes a String as an input and writes to a file in the form of bytes. As these methods may throw exceptions we are writing the code to try and catch blocks.
Example : 2 - File Pointer and Other Methods
Let us take another example to use various remaining methods of RandomAccessFile class such as seek(), length(), getFilePointer(), etc.
file.txt :
myFile.java :
Output :
In the example above, the length() method returns the length of the file in terms of bytes. The readLine method reads data until the line is terminated. The getFilePointer() method returns the number of bytes after which the file pointer is located.
Conclusion
- Random access file in java is used to read and write files in java programs. It is different from the File class as it allows one to perform reading and writing operations simultaneously.
- It extends Object class and implements DataInput and DataOutput interfaces.
- File pointer acts as an index or cursor for the file where the file is a large array of bytes.
- RandomAccessFile class provides various useful inbuilt methods to perform operations on the file.
- Some of the important methods are seek(), getFilePointer(), length(), read(), write(), readFully(), etc.