Random Class in Java
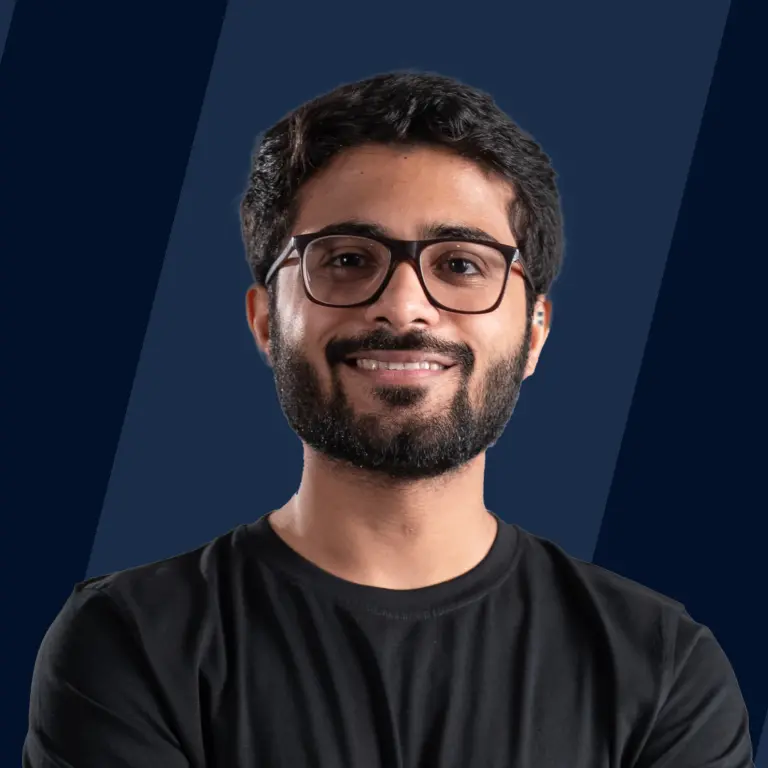
Overview:
This article mainly explains the Random class present in the util package in Java. Java Random class helps in generating a stream of pseudorandom numbers. This article explains about the constructor of the class, different methods present in the Random class in java.
What is a Random Class in Java?
Random class in Java which is present in the util package helps in generating random values of different data types like integer, float, double, long, boolean, etc. We can even mention the range in which we want the random number to be generated. Instances of java. util.Random is thread-safe. However concurrent usage of this instance can be misleading, sometimes it results in poor performance of the program. So, the best solution for this can be instead of using java. util.Random instance we can use ThreadLocalRandom, this is especially used in multithreaded programs. Instances of java. util.Random are not cryptographically secure. Therefore instead of that, we can use SecureRandom to get a cryptographically secure pseudo-random number generator for the use of security-sensitive applications. Classes that are implemented by using class Random can generate up to 32 pseudorandomly generated bits. It uses a protected utility method. For a simple application of generating a random number, we can also use a method which is Math. random().
Declaration of Random Class in Java:
After a brief introduction to the Random class in Java, let's learn more about how to declare a random class in Java. This section mainly explains it. The syntax for declaring a Random class in Java is as follows:
Constructors of Random Class in Java:
This section explains the constructors of the Random class in Java. There are two constructors for the Random class in the Java util package. They are mentioned below:
- Random()
- This constructor creates a new random generator. This method helps in instantiating the Random class and helps in generating random numbers.
- Random( long seed )
- This constructor creates a new random number generator using a single long seed.
- The seed is considered to be the initial value of the internal state of the pseudorandom number which has to be generated.
- Generally, new Random(seed) is equivalent to: Random rand = new Random(); rand.setSeed(seed); Where seed represents the initial value The implementation of method setSeed() used by class Random uses only 48 bits of the given seed.
Methods of Random Class in Java:
S.No | Method | Syntax | Description |
---|---|---|---|
1. | nextBytes(byte[]) | public void nextBytes(byte[] arr) | arr - this arr is a byte array filled with random bytes. This method generates random bytes and stores them in a user-supplied byte array. This method throws a Null Pointer Exception when the parameter arr is empty. |
2. | next(int) | protected int next(int bits) | This method generates the next pseudorandom number. Subclasses should override this, as this is used by all other methods. This method returns the next pseudorandom value from this random number generator's sequence. |
3. | nextInt() | public int nextInt() | This method returns the next pseudorandom, which is a uniformly distributed int value from this random number generator's sequence. The basic idea behind the nextInt() method, is that one int value is pseudorandomly generated and returned. Since for 32 bits, the numbers from o to 2^32^ are possible int values and they are produced with(approximately) equal probability. |
4. | nextInt(int) | public int nextInt(int bound) | The parameters here are bound specifies the range till which the number can be generated. The range of the generated number is from [0, bound-1]. It can generate an int value between 0(inclusive) and bound(exclusive). All bound possible int values are produced with(approximately) equal probability. This method throws a runtime exception i.e, IllegalArgumentException when the bound mentioned is negative. It only allows positive values. |
5. | nextLong() | public long nextLong | This method returns the next pseudorandom which is a uniformly distributed long value from this random number generator's sequence. Since the Random class's seed size has a maximum of 48 bits, it returns randomly all the possible values of long, in the range which is possible. |
6. | nextBoolean() | public boolean nextBoolean() | This method returns either `True or False. The values True and False are generated with(approximately) equal probability. |
7. | nextFloat() | public float nextFloat() | This method returns the next pseudorandom float value, which is a uniformly distributed float value between 0.0 which is included, 1.0 excluded, from the random number generator's sequence. |
8. | nextDouble() | public double nextDouble() | This method returns the next pseudorandom double value, which is a uniformly distributed double value between 0.0 which is included, and 1.0 which is not included, from this random number generator's sequence. |
9. | nextGaussian() | public double nextGaussian() | This method doesn't have any parameters. This method returns the next pseudorandom Gaussian (a double value) distributed double value having the value of a mean as 0.0 and standard deviation as 1.0. |
12. | ints() | public IntStream ints() | This method returns unlimited number of pseudorandom int values. These numbers are generated internally as the result of calling the method nextInt(). |
11. | ints(long) | public IntStream ints(long streamSize) | Here parameter streamSize indicates the number of random numbers to be generated in the program. The value of streamSize is always greater or equal to 0. If it is less than zero, then we encounter a runtime exception i.e, IllegalArgumentException. This method generally returns the stream of pseudorandom int values. |
12. | ints(long, int, int) | public IntStream ints(long streamSize, int randomNumberstart, int randomNumberend) | The parameters here are streamSize indicates the number of values needed to be generated, randomNumberstart indicates the start int from where the numbers can be generated, randomNumberend indicates the bound range. This method returns the streamSize number of int values that are between the range randomNumberstart (inclusive) and randomNumberend (exclusive). The value of streamSize is always greater or equal to 0. If it is less than zero, and also even if the value of randomNumberstart is greater than the value of random numbered then we encounter a runtime exception i.e, IllegalArgumentException. |
13. | ints(int, int) | public IntStream ints(int randomNumberstart, int randomNumberend) | This method returns an unlimited stream of integers in the given range between randomNumberstart (inclusive) and randomNumberend (exclusive). Here randomNumberstart indicates the start point from where the numbers(ints) can be generated, and randomNumberend indicates the bound range. This method gives a runtime exception IllegalArgumentException when the value of randomNumberstart is greater than the value of randomNumberend. |
14. | longs() | public LongStream longs() | This method returns unlimited stream of long values. This method is internally implemented by the result of calling the method nextLong(). |
15. | longs(long) | public LongStream longs(long streamSize) | Here parameter streamSize indicates the number of random numbers to be generated in the program. The value of streamSize is always greater or equal to 0. If it is less than zero, then a runtime exception i.e, IllegalArgumentException. This method generally returns the stream of pseudorandom long values. |
16. | longs(long, long, long) | public LongStream ints(long streamSize, long randomNumberstart, long randomNumberend) | Here the parameters like streamSize indicates the number of values which are to be generated, randomNumberstart indicates the starting point from where the numbers can be generated, randomNumberend indicates the bound range. This method returns the streamSize number of long values which are in the range between randomNumberstart (inclusive) and randomNumberend (exclusive). |
17. | doubles() | public DoubleStream doubles() | This method returns unlimited stream of double values. This method is internally implemented by the result of calling the method nextDouble() |
18. | doubles(long) | public DoubleStream doubles(long streamSize) | Here parameter streamSize indicates the number of random numbers to be generated in the program. The value of streamSize is always greater or equal to 0. If it is less than zero, a runtime exception i.e, IllegalArgumentException. This method generally returns the stream of pseudorandom double values. |
19. | doubles(long, double, double) | public DoubleStream doubles(long streamSize, double randomNumberstart, double randomNumberend) | Here the parameters like streamSize indicates the number of values needed to be generated, randomNumberstart indicates the starting point from where the numbers can be generated, randomNumberend indicates the bound range. This method returns the streamSize number of double values which are in the range between randomNumberstart (inclusive) and randomNumberend (exclusive). This method gives a runtime exception IllegalArgumentException when the value of streamSize is negative and also when the value of randomNumberstart is greater than the value of randomNumberend. |
20. | doubles(double, double) | public DoubleStream doubles(double randomNumberstart, double randomNumberend) | This method returns an unlimited stream of double values in the given range between randomNumberstart (inclusive) and randomNumberend (exclusive). Here randomNumberstart indicates the start int from where the numbers can be generated, and randomNumberend indicates the bound range. This method gives a runtime exception IllegalArgumentException when the value of randomNumberstart is greater than the value of randomNumberend. |
Examples of Random Class in Java:
Below is the Java program using the above-mentioned methods present in the Random class:
Output:
The second example of Java implementation mentions all the different methods
Output:
Another example of a Random class in Java
Output:
Conclusion
- This article explained about Random class from the util package in Java.
- Random() class in Java helps in generating pseudorandom numbers uniformly and returning them.
- Different methods are present in this Random() class which are discussed in the above article.
- Random() method when used continuously can be having poor performance, so to avoid that we use Math. Random() in Java for simple applications.