Random Number Generator in C
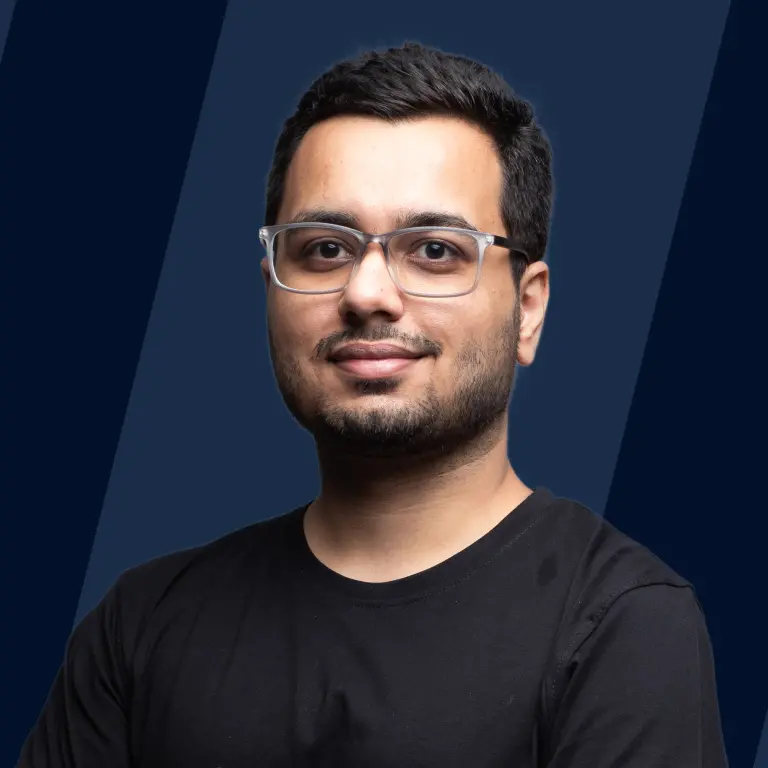
Overview
For random number generator in C, we use rand() and srand() functions that can generate the same and different random numbers on execution.
Pseudo-Random Number Generator (PRNG) In C++
A program where the conversion starting number that is called the seed is transformed to another number different from the seed is known as Pseudo-Random Number Generator (PRNG).
The functions rand() and srand() are inbuilt functions in C that are used for random number generator in C/C++.
rand()
The function rand() is used for random number generator in C in a certain range that can take values from [0, Range_max]. Range_max value can be an integer.
Syntax
It does not take any parameters, and it returns random numbers.
srand()
The srand() function is used to initialize the starting point i.e., the value of the seed. If srand() is not initialized then the seed value in rand() function is set as srand(1).
Syntax
srand takes a parameter that is used to set the starting value of the random number generator. There is no return value.
srand is known as the set seed for the rand() function.
Difference Between rand () and srand () with example
rand() | srand() |
---|---|
It is used for random number generator in C. | It is used to initialize the seed value of PRNG. |
It does not take any parameters. | It takes the value that seeds the random number generator. |
It can be called any number of times the user wants. | It is only called once to see the random number. |
Returns random numbers whenever called | There is no return value in srand() |
Example of rand() function
Program - Generate a random number using rand() function in C.
Output
In the above result, the same number is generated after every execution it is because the value of srand() is fixed, which is 1.
To generate different random numbers we will use srand(time()). We use the time library here because it will change the value of the generated number on every execution.
Example of srand() function
Program - To generate random numbers on every execution using srand() in C.
Output
The random numbers generated change on every execution because we are using UNIX timestamp, time function from time.h library.
C++ Random Float
As we can see from the above results, the value generated on every execution is an integer value that can be very long.
So, we can use float or double values.
To do that we can use the following syntax
Program to generate random float number
Output
In the result, we can see that we get a random float number.
C++ Random Number Between 0 And 1
Program to generate different random numbers between 0 and 1.
Output
In the above result, we can see that different random numbers are generated between 0 and 1.
C++ Random Number Between 1 And 10
Program using only rand() function:
The above program will give the same result on every execution. The randomly generated numbers will remain the same.
Output
The above results give 10 different random numbers generated using the rand() function.
Now, to get different random numbers on execution we will use the srand() function.
Program to generate 10 random numbers usingsrand()function.
The above program will generate different random numbers on every execution.
Output
Result 1
Result 2
Conclusion
- The rand() and srand() functions are used to generate random numbers in C/C++ programming languages.
- The rand() function gives same results on every execution because the srand() value is fixed to 1.
- If we use time function from time.h library in srand() function, we can generate different random numbers on every execution.