Random Password Generator in Python
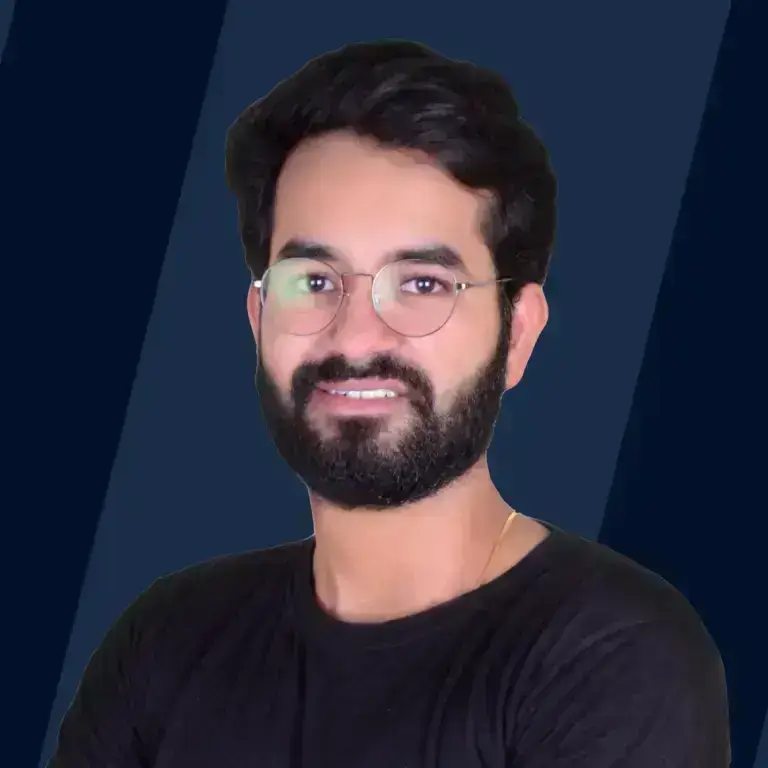
Overview
The idea of building a "random password generator" in Python is very simple. First, we will create a string or list consisting of all the alphabets (both small and capital alphabets), numbers, and symbols. We will take the length of the required password as input from the user. Finally, we will use a loop ranging between 0 and the length of the password, and in every iteration, we will randomly choose a letter from the set of letters defined above and store them into a resultant password.
What we are Building ?
So, in this article, we will be building a random password generator in Python. The "random password generator" in Python is a program that will generate strong random passwords of the specified length using alphabets, numbers, and symbols. Let us first learn the prerequisites and build the intuition of the program so that the implementation can become easier.
Pre-requisites
A good understanding of Python programming language along with the data structures and random module is what we need to develop a random password generator in Python.
We will be using :
- the list data structure,
- the string data structure,
- loops (for loop and while loop).
We will primarily use the built-in Python module namely- the random module. We will use the various methods of the random module to randomly choose the letters of the password from the list of alphabets, symbols, and numbers.
Apart from these, a basic understanding of the Tkinter module and its associated function is very helpful in understating the GUI approach of developing a random password generator in Python.
Let us now learn the overall idea and the steps needed to develop a random password generator in Python.
How are We Going to Build a Random Password Generator?
The idea of building a random password generator in Python is very simple. First, we will create a string or list consisting of all the alphabets (both small and capital alphabets), numbers, and symbols.
Now, we will take the length of the required password as input from the user. Finally, we will use a loop ranging between 0 and the length of the password, and in every iteration, we will randomly choose a letter from the set of letters defined above and store them into a resultant password.
Refer to the following sections for more clarity and implementation.
Final Output
If the user inputs length as then one of the randomly chosen passwords will look like this :
Requirements
For developing a random password generator in Python, we will need a good understanding of Python programming like the working of loops, operators, and functions. Along with that, we will be using some data structures like lists, strings, etc.
Apart from these, we need to use the random module of Python. The random module in Python provides us with various functions that help us to randomly choose among various values. Some of the most important functions of the random module are randint(), sample(), choice(), seed(), sample(), etc.
In this random password generator program, we will be using some of the functions of the random module. Let us discuss them before using them in the implementation part.
-
choice() :
The choice() method is one of the most important methods of the random module. The choice() method selects a random value (or element) from the provided sequence. Now this sequence can be any sequential data type like strings, lists, tuples, or any other sequence of numbers or objects. -
sample() :
The sample() method is used to obtain a sequence of randomly selected values from the provided input sequence. The sample() method returns a list of unique choices. The sample() method takes two parameters. The first parameter is the original sequence from which the output random sequence has to be generated. The second parameter is the length of the random sample that needs to be generated.
In this article, we will also be developing a simple GUI (Graphical User Interface). For developing the GUI, we can use the Tkinter module in Python. The Tkinter module is not a built-in module, so we need to use install the Tkinter module before using it.
The command for installing the "Tkinter" module is :
Building the Password Generator
Let us now implement the random password generator in Python for better understanding.
1. Generate a Password Using All Alphanumeric Characters
Before getting into the implementation of generating random passwords using all alphanumeric characters, let us discuss the steps involved.
Note :
At the very start, we need to import the random module.
- Create a function generatePassword() that will return a randomly generated password. The generatePassword() function takes one argument i.e. n which denotes the length of the password to be generated.
- Create a string or list consisting of all the alphabets (both small alphabets and capital alphabets), numbers, and symbols.
- Initialize an empty string that will store the randomly selected password.
- At last, we will run a loop from to n. In each iteration, we will randomly choose a character from the list of characters (using the random.choice() method) and add the selected character to the password string. Finally, we will return the password string at the end of the loop.
Output :
As we can see above, the generated password has some repeated character(s). Now, we can also generate a password that contains a unique character using the sample() method of the random module. The sample() method only chooses unique value(s) from the provided sequence. We can specify the length of the sequence needed. So, we will provide the value of n into the sample() method so that we do not need to use the loop. Let us see the implementation for a better understanding.
Output :
2. Update the Password Generator to Receive the Password Length as the User Input
In the earlier program, we explicitly provided the length of the required password. We can take the length of the password from the user using the input() method in Python. Let us see the implementation for a better understanding.
Output :
3. Create a Python Password Generator that Generates Multiple Passwords
So far we have seen how we can generate a random password of a specified length but we can also generate multiple passwords using the same logic as discussed above.
For generating multiple random passwords, we can use an infinite loop that will take the length of the required password and generate the password. We can ask a simple question Please enter 'yes' if you want to generate a password else type 'no' to exit.
Whenever the user types yes we will generate the password and when the user types no. The infinite loop will break and the program execution will stop.
Let us implement the program for a better understanding.
Output :
4. Integrating GUI in the Password Generator Using Tkinter
Tkinter is a standard Python library used to develop GUI applications in Python.
Please note that we need to install the Tkinter module before importing and using it as Tkinter is an external module that does not come with a Python interpreter.
1. Importing required libraries :
Apart from the Tkinter, we need to use the Checkbox library from the msilib.schema module. The Checkbox library is used to create a checkbox that will contain the size of the password to be generated.
So the importing statements will look like this :
2. Creating window screen :
Now, after all the required libraries and modules are imported, we will create a GUI window of size (600 x 600 or any other size of your choice). We will make this window and provide a title of our choice.
We will also use the label() method of the Tkinter module to insert a label on the screen. The label will is placed on the screen using the pack() method of the Tkinter module. We can also specify the text, font color, background color, etc on the label for better visuals.
The window generation code will look like this :
3. Creating the "passwordGeneration()" function :
In this function, we will create a random password string of the specified length using the random.sample() method. After that, we will create a label and add the generated password to that label. At last, we will add that label on the window using the place() method.
The passwordGeneration() function will look like this.
4. Creating the checkboxes :
Now, we will create some checkboxes using the Checkbox() method and we will place the checkbox on the window label.
The checkbox code will look like this :
5. Getting the length of the password :
We will convert the length obtained from the checkbox into an integer value.
The checkbox code will look like :
6. creating a button for the window :
At last, we will create a button using the Button() method and then place it on the window.
The button code will look like this :
As we can see, we have provided the getLength() function that will call the passwordGeneration() method with the specified length.
Entire Code :
Let us see the implementation of the entire code and see the output
Output :
The first screen will look like this :
Now, we have selected the length on the checkbox.
Finally, we will press the Generate button and the output (the required password) will be shown on the output window screen.
Why Create a Random Password Generator ?
A good and strong password is one of the most essential things in today's world where our data and security is our prime concern. People usually set common passwords that can be easily used by hackers to obtain our data.
A better way to learn any programming language is by learning by doing. Specifically developing a good starter project like the random password generator in Python helps brush up on the fundamentals. This project also helps us to understand the usage of various built-in and external modules of the Python programming language.
How to Generate Strong Passwords in Python ?
We have discussed the various ways of developing the random password generator in Python. We can use the sample() method in place of the choice() method to generate a stronger password having no repetition of digits also, we can ask the user to enter the number of letters, characters, and symbols that he/she wants in the password.
Let us see the implementation for a better understanding.
Output :
The reason for using the shuffle() method is that the list of passwords will first contain characters, then numbers, and then symbols in every execution. So, we have used the shuffle() method to shuffle the password so that the numbers, characters, and symbols get shuffled.
Conclusion
- The random password generator in Python is a program that will generate strong random passwords of the specified length using alphabets, numbers, and symbols.
- We first create a string or list consisting of all the alphabets, numbers, and symbols.
- We will use a loop ranging between 0 and the length of the password and in every iteration we will randomly choose a letter from the set of letters and store them into a resultant password.
- We can also generate multiple random passwords by using an infinite loop that will take the length of the required password and generate the password.
- The choice() method selects a random value (or element) from the provided sequence. Now this sequence can be any sequential data type like strings, lists, tuples, or any other sequence of numbers or objects.
- The sample() method is used to obtain a sequence of randomly selected values from the provided input sequence. The sample() method return a list of unique choices.
- We can use the sample() method in place of the choice() method to generate a stronger password having no repetition of digits.
- We can use the Tkinter module which is an external module to create a GUI for the random password generator