Random String Generator in JavaScript
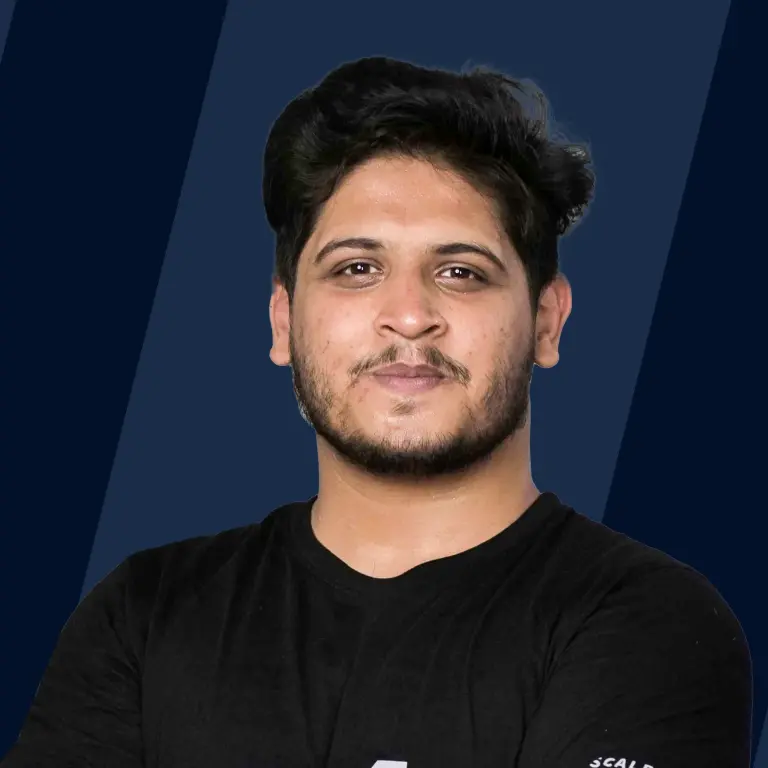
Overview
Generating random strings is a common task in web development, often used for creating secure passwords, unique identifiers, or simulating data. JavaScript offers several methods to generate random strings efficiently. In this article, we will explore different approaches to random string generator javascript, along with their use cases and code examples.
Use of Random String Generator
Random string generators Javascript are versatile tools in JavaScript, finding applications in various scenarios. Here are ten common use cases where random string generators prove invaluable:
- Password Generation:
Create strong and secure passwords for user accounts, enhancing the security of web applications and user data. - Token Generation:
Generate unique authentication tokens for user sessions or API endpoints, ensuring secure and controlled access to resources. - Data Simulation:
Simulate random data for testing and prototyping applications, allowing developers to assess system behavior under diverse conditions. - Usernames and Display Names:
Generate unique usernames or display names for online platforms, ensuring a distinct identity for each user. - File or Folder Naming:
Create random names for files, folders, or temporary resources, preventing naming conflicts and maintaining system organization. - Session Identifiers:
Generate session IDs for tracking user interactions and maintaining state in web applications. - Coupon Codes:
Create unique coupon or discount codes for e-commerce platforms, promotions, or loyalty programs. - CAPTCHA:
Generate CAPTCHA codes to verify that website interactions are performed by humans rather than automated bots, enhancing security. - Database Records:
Populate databases with random data for testing and development purposes, mimicking real-world scenarios. - Unique URLs:
Generate unique URLs for temporary access to shared documents or resources, ensuring privacy and security.
These diverse use cases highlight the flexibility and importance of random string generators in JavaScript, contributing to the functionality and security of web applications and systems.
Let's dive into the methods to generate random strings in JavaScript.
Generating Random Strings in JavaScript
Basic Approach
The basic approach to random string generator javascript involves selecting characters from a predefined character set and arranging them in a specific order to form the desired string. The steps include:
- Define a character set containing all possible characters for the random string.
- Generate random indices within the range of the character set.
- Concatenate the selected characters to form the random string.
Example:
Output:
Methods to Generate Random Strings in JavaScript
Approach - 1: Generating the Random String from the Specified Length of the Character Set
This approach allows you to create random strings of a fixed length by selecting characters from a character set. The following is the approach:
- Define a character set containing all possible characters for the random string.
- Generate random indices within the range of the character set.
- Concatenate the selected characters to form the random string.
Example - 1:
Code:
Output:
Explanation of example:
This code defines a function generateRandomString that takes a length parameter for the desired string length and a charset parameter for the character set to choose from. It then iterates length times, selecting random characters from the provided charset and concatenating them to create a random string generator javascript.
Example - 2:
Code:
Output:
Explanation of example:
In this example, we define a custom character set customCharset containing specific characters. The generateRandomString function is used to create a random string generator javascript of length 6 by selecting characters from this custom character set. The output is a random string composed of characters 'A', 'B', 'C', '1', '2', and 'X' from the custom set.
Example - 3:
Code:
Output:
Explanation:
In this example, we define a custom character set specialCharset containing special characters commonly used in passwords and secure tokens. The generateRandomString function is used to create a random string generator javascript of length 12 by selecting characters from this custom character set. The output is a random string composed of special characters.
Approach - 2: Generating the Random String by Using the String.fromCharCode() Function
This approach generates random strings by selecting characters based on their Unicode values using the String.fromCharCode() function. The following is the approach:
- Generate random character codes within the ASCII range (33-126).
- Convert the character codes to characters using String.fromCharCode().
Example - 1:
Code:
Output:
Explanation of example:
This code defines a function generateRandomString that takes a length parameter for the desired string length. It generates random character codes within the ASCII range (33-126) and converts them to characters using the String.fromCharCode() function. The resulting characters are concatenated to form a random string generator javascript of the specified length.
Example - 2:
Code:
Output:
Explanation of example:
In this example, we use the generateRandomString function to create a random string generator javascript of length 8 by selecting characters from the ASCII range 'A' to 'Z'. It generates random character codes within this range and converts them to characters using the String.fromCharCode() function. The output is a random string generator javascript composed of uppercase letters.
Example - 3:
Code:
Output:
Explanation:
In this example, we use the generateRandomString function to create a random string generator javascript of length 6 by selecting characters from the ASCII range '0' to '9'. It generates random character codes within this range and converts them to characters using the String.fromCharCode() function. The output is a random string generator javascript composed of numeric digits.
FAQs
Q. How can I generate a random string with specific characters only?
A. You can define your custom character set and use it as an argument in the generateRandomString function.
Q. Is there a way to generate cryptographically secure random strings?
A. Yes, you can use the crypto.getRandomValues method or libraries like crypto-random-string to generate secure random strings.
Q. What's the maximum length of a random string I can generate using these methods?
A. The maximum length of a random string depends on factors like the character set and the JavaScript environment. In practice, you can generate strings of several thousand characters, but it's essential to consider performance and memory constraints.
Q. Can I use random strings for generating unique filenames in a file storage system?
A. Yes, random strings are commonly used to generate unique filenames to prevent naming conflicts when storing files in a storage system.
Conclusion
- JavaScript provides various methods for generating random strings, catering to different use cases.
- These methods include "Character Set Selection" and "Unicode Character Codes" approaches.
- Custom character sets can be used to tailor random string generation.
- JavaScript's flexibility allows developers to adapt these methods for diverse scenarios.
- Secure random string generation is crucial for protecting sensitive data.
- Random strings can serve as unique identifiers and enhance web application functionality.
- These methods are versatile and can produce random strings of varying lengths.