raw_input() in Python
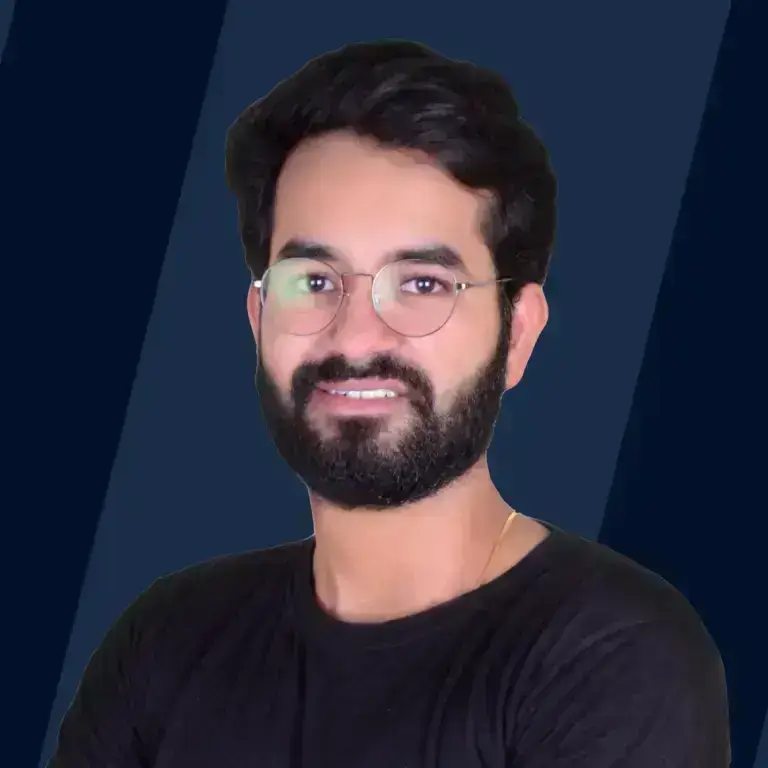
Overview
Programs often need to interact with the user to take input from the user and perform some desired operation and give the output. The next question that pops into our mind as a programmer is, "How do we take an input from the user?". There are many ways in which we can take input from the user in Python. The raw_input() function in python 2. x is one of the methods to take the input from the user.
Syntax of raw_input() function in Python
The syntax of raw_input() function in python is as follows :
The raw_input() function takes a prompt (String) as a parameter. The value returned by the function is stored in the variable named var.
Parameters of raw_input() in Python
The raw_input() function takes a string as a parameter. As we saw previously in the syntax, The raw_input() in python takes prompt as the parameter. Here, prompt refers to the message to be displayed while the program waits for the user to give the input. It is not necessary that we always give a parameter to the raw_input() function, but to make it user-friendly, it is recommended to add the prompt. A prompt lets the user know what is required by the program and what has to be entered.
raw_input() Function with a Parameter
The above code snippet of code when executed, will prompt a message "Enter your name" and waits for the user to give input and store it into the variable name before executing the next line of code.
raw_input() function without a parameter
The same code can be written without the prompt message as follows -
When we run the above snippet of code, the program will stop executing and wait for the user to give any input. As we have not included any prompt message, the program will simply stop for the user to enter the input. Once the user has given input, the program will continue executing the next line.
Return Values of raw_input() function in Python
The raw_input() function in python 2 returns the value as a string. Once the user has given the input, whether the input is in the form of int, double, string, etc. The raw_input() function explicitly converts the entered data into string and will return a string.
Example of raw_input() function in Python
With String as Input
Code:
Output:
With Integer as Input
Code:
Output:
Explanation:
From the above examples, we can infer that the raw_input() function returns a string by explicitly converting it irrespective of the type of data entered.
Exceptions of raw_input() in Python
The raw_input() function works only in python 2 and does not work in python 3 . python 2 supports both input() and raw_input() functions but python 3 supports only input() function.
Note The properties of the input() function are different in python 2 and python 3
What is raw_input() in Python?
The raw_input() function in python 2 is used to take the input from the user through an input device. The raw_input() function is different from the input() function in python 2 which we will discuss in the latter part of this article. The raw_input() takes a string as a parameter, which will be printed in the output for the ease of user in entering the input. The function returns the value entered by the user is converted into string irrespective of the type of input given by the user.
Note: It is important to note that the raw_input() function works only in python 2.
More Examples
Program in Python 2
Program with a string as input
Code:
Output:
Explanation:
In the above example, we took the favorite color of the user as input and stored it in the fav variable. Later we printed it out using the print() function.
Program with an integer as input
Code:
Output:
Explanation:
In the above example, we took the shoe size of the user as input and stored it in the size variable. Later we printed it out using the print() function.
Program without parameter (prompt)
Code:
Output:
Explanation:
In the above code, we used the raw_input() function without a parameter or a prompt. When the program is executed, the program executes the first line of code and waits for the user to give the input, only then the next line is executed.
It is difficult and confusing for the user to understand why the program stopped and what should be given as input. Therefore it is recommended to use the prompt while using the raw_input() function.
Program in Python 3
Code:
Output:
Explanation:
The above program did not run as we expected and we encountered an error. This is because python 3 doesn't support the raw_input() function which can be seen in the error output.
Difference between input() and raw_input() Function in Python
-
Python 2.x : In python 2, we have both the raw_input() function and the input() function, The main difference between both the functions is the return type of the function. The raw_input() function explicitly converts the input and returns a string as output whereas the input() function takes the value and type of the input you enter as it is without modifying the type.
-
Python 3.x : Python 3 doesn't support the raw_input() function. Python 3 supports only input() function but input() function in python 3 explicitly converts the input into string.
Conclusion
- The raw_input() function which is available in python 2 is used to take the input entered by the user.
- The raw_input() function explicitly converts the entered data into a string and returns the value.
- The raw_input() can be used only in python 2.x version.