How to Read *.CSV File in JavaScript?
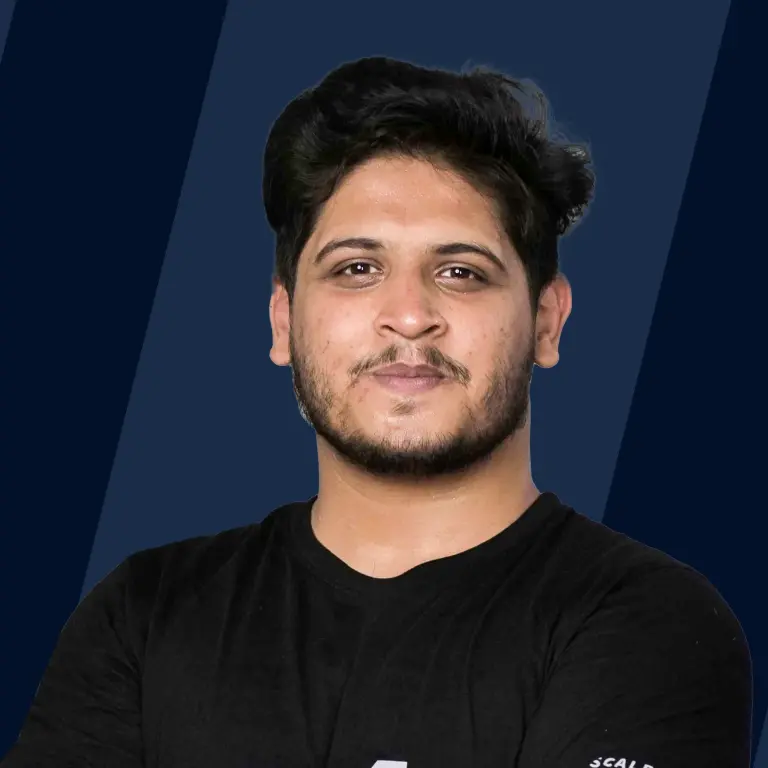
Overview
The CSV is the acronym for comma-separated values. In javascript, we can read CSV files using various modules, methods, and node.js streams. We can read large datasets that are stored as a CSV file, we can modify the data, and also drop the data.
Introduction
The plain text file format known as a CSV is used to store tabular data. Values in table cells are separated in the CSV file by commas, and the beginning and end of each row are indicated by new lines. Most databases and spreadsheet apps support exporting and importing CSV files. There are various modules for reading a csv file for example, node-csv, fast-csv, and papaparse.
We can read the javascript CSV files using the Node.js streams that allows the user to read huge datasets without consuming much capacity of RAM. We can use the SQL lite database to transfer the data from a javascript CSV file. Apart from this, we can also transfer data in chunks by first getting the data from the database, parsing it using node-CSV, and then using the Node.js streams for output.
JavaScript Program to Read *.CSV File in JavaScript Using csv-parse
We can read a CSV file from the environment of Node.js by using the CSV-parse module that is available in npm. The CSV-parse module is an efficient and reliable CSV parser module that Node developers are using for many years. The CSV-parse module in javascript is a subset of the node-csv module. It consists of a set of libraries that are used to manage the CSV files by using Node.js. Given below are the steps that we need to follow for reading a *.CSV File in JavaScript Using CSV-parse:
- First, we need to install the CSV-parse module with the help of npm by the following command:
npm install csv-parse
Then we will import the fs and csv-parse modules into that specific JavaScript file by using the given command:
For reading a csv file using the csv-parse, first, we will create a stream that is readable by the use of the fs.createReadStream() function. Given below is an example that is used to show how to create a readable stream file by using the fs and then we need to replace the parameter ./example.csv with the path of our csv file:
Now, the stream data will be connected to the parse() function (the stream data will be treated as the input) by using the given code:
The csv file will be read at the .on("data) event. As we can see there are rows where each data of the CSV file is filled. For example, let us assume we have a csv file namely example.csv in which the below contents are filled.
When we run the JS file, the program will look like this:
Also, the user can make an object for each of the CSV file rows and in this row, the data is kept as key-value pair. The first row will be used as the key and then the values will be filled. If we want to do this, we need to modify the options that are being passed to the parse() method by using the code as shown below:
The ltrim is used for cutting the whitespace that is present in the data file. Next, we need to create an empty array variable namely data and then we will push each of the data containing row into the array. This will look like this:
These are the steps that we need to do for reading a CSV file using the JavaScript CSV-parse. After doing this, the final output will look like this:
JavaScript Program to Read *.CSV File in JavaScript with Node.js Native Module
To read a csv javascript file using the Node.js native module, we will use the fs and readline of the native modules. For reading the file at once we can use the code given below:
The above code will result in a string that we can later process as an array or as an object. We can also read the file row by row by using the fs.createReadStream() and readline modules by creating a readable stream as shown below:
The readline interface helps to read and trigger each of the line events. When this gets executed, the close event gets executed by the readline. We can also read a javascript csv file without any third-party module, but this will need extra work to format the output.
The header row in the above example is treated in the same manner as the data rows. For better formatting of the CSV text, you must write more code.
JavaScript Program to Read *.CSV File in JavaScript from the Browser with D3.js
D3.js is a javascript library that is used for the manipulation and processing of data. For reading the csv file using the D3.js, we need to go through the following steps:
First, we need to call the csvParse() method and then pass a string as given below:
This will produce a result like this:
However, you often need to receive a CSV file using the HTML <input> element if you're parsing a CSV file that came from the browser. We can also read a given csv file using the FileReader API and D3.js as shown in the code below:
In the above code, first, we created a form element <form>, and then we added an event listener for the submission of the form element using javascript. And then the file that is being uploaded as csv file will be processed by the D3.js. That's how we can read a csv javascript file using the D3.js.
JavaScript Program to Read *.CSV File in JavaScript Using the fs Module
The term fs is the acronym for "file system". The file system module has many commands that are used for making interaction with the file system in Node.js. There are two ways for reading a CSV file using the fs module that we are going to cover below one by one.
Read the whole file at once There are two commands in the fs module that can be used to read the csv file in Node.js. The first command is readFile() and the second command is readFileSync(). They both are almost the same but the only thing that makes them different is the command readFileSync() is synchronous. It means when we use this command to read the csv file, it blocks the other contemporary reading process. But the command readFile() is asynchronous which means it does not blocks the other commands from executing when it is getting executed. As we know, CSV files are generally time taking in case of large files, so it is advised to use the asynchronous readFile() which is shown below:
Read line by line When we use the readFile() to read the CSV file, it also takes much memory because it reads the whole csv file at once. So, to overcome this problem, we use the command namely fs.createReadStream() as shown below:
In the above code, we are passing the csv file name to the fs.createReadStream() method that creates a readable stream. The stream helps the user to access a large amount of data by creating simpler chunks of larger data.
After creating the readable stream, we need to pass it to the read.createInterface() method. An interface for reading the data one line at a time is provided by the readline module. As each row is read, you may now push it into the data array.
However, keep in mind that this code only divides each row on commas. Although the simplest CSV file can be used with this, the format is actually more complex than what its name suggests. Manually parsing CSV files is not a reliable method, especially if you do not own the data. The majority of the time, a CSV library is the best option.
JavaScript Program to Read *.CSV File in JavaScript Using fast-csv
We can use the fast-csv in javascript to read a csv file very faster, reliably, and easily. The fast-csv can be found in the npm package. Using the fast-csv, we can easily read the csv file, and also we can format the data easily. For using the fast-csv first we need to initialize the npm and then install the fast-csv by using the command given below:
Then we use the fast-csv to read the data as given below:
In this code first, we created a readable stream from the csv file and then piped the file into the parse method by using the pipe() method. One thing to keep in mind is that here we are passing the headers option to the csv.parse() method. This makes the method skip the first row. So, set the header to False if there is an absence header in the first row.
Each row is pushed to the data array as the CSV file is read one row at a time. You can edit the data array's contents once the entire file has been read.
JavaScript Program to Read *.CSV File in JavaScript Using Node-CSV
Given below are the steps that need to be followed to read csv javascript file using the Node-CSV:
First, we need to create a readCSV.js in the editor as shown below:
In the readCSV.js file, we will import the fs and csv-parse modules using the command given below:
Then we will add the given lines to the CSV file:
The filename you want to read can be passed as input to the createReadStream() method of the fs module; in this case, the filename is migration data.csv. The process then generates a readable stream, which divides a big file into smaller pieces. You can only read data from a readable stream; you cannot write data to it.
The parse() method that accepts properties is treated as an object. Then the objects give more details about the data that the method is going to parse. The data event gets triggered when the data is transformed from the parse() method. You must give a callback to the on() method, which accepts a row parameter, in order to retrieve the data. A data chunk turned into an array serves as the row parameter. You use the console.log() method to log the data in the console from within the callback.
Additional stream events will be added before running the file. When all the data in the CSV file has been ingested, these stream events handle issues and output a success message to the console.
The final file will look like this:
Now, we need to save the file namely readCSV.js by using the shortcut CTRL+X. And then we will run the command given below and it will read csv javascript file:
JavaScript Program to Read *.CSV File in JavaScript Using Papa Parse
We can simply read csv javascript files by core program, but the papa parse plugin provides us more and better options for parsing our CSV file. The papa parse is almost supported in all browsers except the Internet Explorer below 10 version. There are some features of the papa parse that are listed below:
- It is easy to use and it parses the csv file very fast.
- It parses the csv files directly.
- We can resume, abort and pause the parsing of the csv file.
- Numbers and booleans can be converted into their respective types.
- It supports the header concept.
- We can also perform reverse parsing.
- Good for larger files.
The steps to read csv javascript using the papa parse method is as follows:
- First we need to include the papa parse and JQuery files into the header of the file namely index.html as shown below:
- Now, we will create an HTML form where we will upload a file. Given below is the code:
- Now, initialize the papa parse to parse the csv file where we will set the configurational parameters. Add the code shown below at the bottom of the file.
The above code gets executed when the user clicks the submit file button. Some parameters, including handlers, callback complete function, and delimiter, can be configured using configuration objects. When the CSV file has been uploaded and the data has been sent to the papa parse instance, we use the submit callback function to perform the parsing operation and display the CSV data in an HTML table.
- At last we will define the function namely displayHTMLTable() that is used to display the CSV file in the table. Given below is the code for that:
In the above code, the table rows are created by using the CSV data. Line 18 of the code is used for setting the HTML string data to the div container in which the csv data is listed.
Conclusion
- There are various modules in javascript for reading a csv file such as node-csv, fast-csv, and papa parse.
- To read a CSV file using Node.js streams, which allows you to read huge datasets without using a lot of RAM, use the node-CSV package.
- The CSV-parse module in javascript is a subset of the node-csv module. It consists of a set of libraries that are used to manage the CSV files by using Node.js
- The ltrim is used for cutting the whitespace that is present in the data file.
- To read a csv javascript file using the Node.js native module, we will use the fs and readline of the native modules.
- The readline interface helps to read and trigger each of the line events.
- The file system module has many commands that are used for making interaction with the file system in Node.js.
- There are two commands in the fs module that can be used to read the csv file in Node.js that are the first command is readFile() and the second command is readFileSync().
- Manually parsing CSV files is not a reliable method, especially if you do not own the data. The majority of the time, a CSV library is the best option.
- Some features of the papa parse are it supports the header concept, and we can also perform reverse parsing, good for larger files.